-
Bitcoin
$84,993.8786
0.34% -
Ethereum
$1,599.9353
1.27% -
Tether USDt
$0.9999
-0.01% -
XRP
$2.0813
0.78% -
BNB
$592.4105
0.82% -
Solana
$138.1937
3.17% -
USDC
$1.0000
0.00% -
Dogecoin
$0.1589
2.71% -
TRON
$0.2413
-1.55% -
Cardano
$0.6307
2.60% -
UNUS SED LEO
$9.3881
1.71% -
Chainlink
$12.8366
1.65% -
Avalanche
$19.2740
1.26% -
Stellar
$0.2445
1.24% -
Toncoin
$2.9868
-0.07% -
Shiba Inu
$0.0...01221
3.10% -
Hedera
$0.1669
0.87% -
Sui
$2.1351
1.39% -
Bitcoin Cash
$336.8750
2.70% -
Hyperliquid
$18.0804
6.57% -
Polkadot
$3.7246
1.15% -
Litecoin
$76.4144
1.52% -
Dai
$0.9999
0.00% -
Bitget Token
$4.4597
2.23% -
Ethena USDe
$0.9992
0.00% -
Pi
$0.6437
5.71% -
Monero
$212.9449
-1.50% -
Uniswap
$5.2448
1.29% -
Pepe
$0.0...07289
2.88% -
OKB
$50.8316
2.01%
How to export Upbit's historical K-line data?
To export Upbit's historical K-line data, set up Python, use the Upbit API's candles endpoint, handle pagination, and save the data in CSV format.
Apr 15, 2025 at 09:01 pm
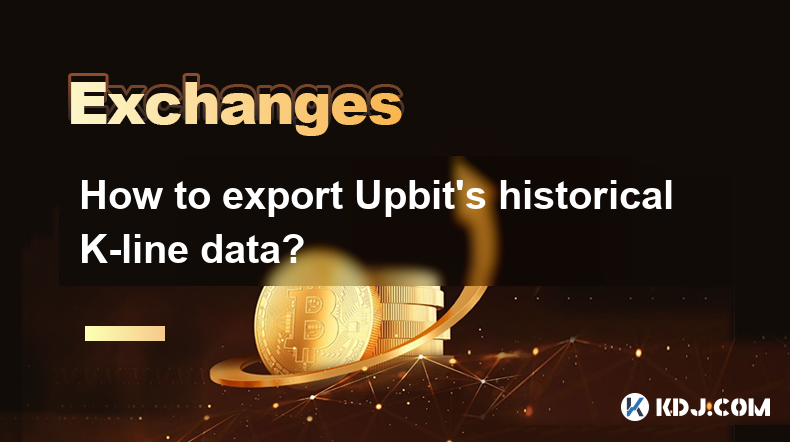
How to Export Upbit's Historical K-line Data?
Exporting historical K-line data from Upbit can be crucial for traders and analysts who need to study market trends and make informed trading decisions. Upbit, being one of the largest cryptocurrency exchanges in South Korea, provides a wealth of data that can be accessed through its API. In this article, we will guide you through the process of exporting Upbit's historical K-line data in detail.
Understanding Upbit's API
Before diving into the steps for exporting data, it's important to understand the basics of Upbit's API. Upbit's API allows users to access real-time and historical market data, place orders, and manage their accounts programmatically. For exporting historical K-line data, we will focus on the candles endpoint, which provides the necessary information in the form of candlestick data.
Setting Up Your Environment
To begin, you will need to set up your development environment. Here are the steps to do so:
Install Python: Ensure you have Python installed on your system. You can download it from the official Python website if you haven't already.
Install Required Libraries: You will need to install the
requests
library to make HTTP requests to the Upbit API. You can install it using pip:pip install requests
API Access: You will need to create an API key on Upbit's website. Navigate to the API management section, create a new key, and keep the API key and secret safe.
Making API Requests
Once your environment is set up, you can start making API requests to retrieve the historical K-line data. Here's how to do it:
Import Required Libraries: Start by importing the necessary libraries in your Python script.
import requests
import json
from datetime import datetime, timedeltaDefine API Endpoint: The endpoint for retrieving candles is
https://api.upbit.com/v1/candles/minutes/{unit}
. Here,{unit}
can be 1, 3, 5, 10, 15, 30, 60, or 240, representing the time interval of each candle in minutes.Set Parameters: You need to set parameters such as the market (e.g.,
KRW-BTC
), the candle unit, and the date range. For example, to retrieve 1-minute candles for KRW-BTC over the last 24 hours, you can set the parameters as follows:market = "KRW-BTC"
unit = 1
to = datetime.now()
from_ = to - timedelta(days=1)Construct the URL: Combine the endpoint and parameters to construct the URL for the API request.
url = f"https://api.upbit.com/v1/candles/minutes/{unit}?market={market}&to={to.isoformat()}&count=200"
Send the Request: Use the
requests
library to send a GET request to the constructed URL.response = requests.get(url)
data = response.json()
Processing and Saving the Data
After receiving the data, you need to process it and save it in a suitable format. Here's how to do that:
Parse the Data: The data received will be in JSON format. You can parse it and extract the relevant information such as timestamp, opening price, high price, low price, closing price, and trading volume.
for candle in data:
timestamp = candle['candle_date_time_utc'] opening_price = candle['opening_price'] high_price = candle['high_price'] low_price = candle['low_price'] closing_price = candle['trade_price'] volume = candle['candle_acc_trade_volume'] # Process the data as needed
Save the Data: You can save the processed data in various formats such as CSV, JSON, or even a database. Here's an example of saving it as a CSV file:
import csv
with open('upbit_kline_data.csv', 'w', newline='') as csvfile:
fieldnames = ['timestamp', 'opening_price', 'high_price', 'low_price', 'closing_price', 'volume'] writer = csv.DictWriter(csvfile, fieldnames=fieldnames) writer.writeheader() for candle in data: writer.writerow({ 'timestamp': candle['candle_date_time_utc'], 'opening_price': candle['opening_price'], 'high_price': candle['high_price'], 'low_price': candle['low_price'], 'closing_price': candle['trade_price'], 'volume': candle['candle_acc_trade_volume'] })
Handling Pagination
Upbit's API has a limit on the number of candles it returns in a single request. To retrieve more data, you need to handle pagination. Here's how to do it:
Initial Request: Make the initial request as described earlier.
Check for More Data: Check if there are more candles available by looking at the timestamp of the last candle in the response.
Subsequent Requests: Use the timestamp of the last candle to make subsequent requests. Update the
to
parameter in the URL to the timestamp of the last candle received.while data: last_timestamp = data[-1]['candle_date_time_utc'] url = f"https://api.upbit.com/v1/candles/minutes/{unit}?market={market}&to={last_timestamp}&count=200" response = requests.get(url) new_data = response.json() if new_data: data.extend(new_data) else: break
Error Handling and Best Practices
When working with APIs, it's important to implement error handling and follow best practices. Here are some tips:
Error Handling: Use try-except blocks to handle potential errors such as network issues or API rate limits.
try: response = requests.get(url) response.raise_for_status()
except requests.exceptions.RequestException as e:
print(f"Error occurred: {e}")
Rate Limiting: Be mindful of Upbit's rate limits. Implement delays between requests if necessary to avoid hitting the rate limit.
import time
time.sleep(1) # Wait for 1 second between requests
Data Validation: Validate the data received from the API to ensure it meets your expectations.
if not data:
print("No data received")
else:
for candle in data: if 'candle_date_time_utc' not in candle: print("Invalid data format") break
Frequently Asked Questions
Q: Can I export historical K-line data for multiple cryptocurrencies at once?
A: Upbit's API does not support batch requests for multiple markets in a single API call. You will need to make separate requests for each cryptocurrency you are interested in.
Q: How far back can I retrieve historical K-line data from Upbit?
A: Upbit provides historical data for up to two years for most markets. However, the availability of data may vary depending on the specific market and candle unit.
Q: Is there a limit on the number of API requests I can make per day?
A: Yes, Upbit has rate limits on its API. The exact limits depend on your API key type. It's important to check the documentation and implement proper rate limiting in your code to avoid hitting these limits.
Q: Can I use the exported data for commercial purposes?
A: It's essential to review Upbit's terms of service and API usage policy to understand any restrictions on using the data for commercial purposes. Always ensure compliance with their policies.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- Codename:Pepe dips 30% from March highs, sparking investor interest in Codename:Pepe's AI-powered alternative.
- 2025-04-19 14:20:14
- Despite Its Elevated Position as the World's Premier Meme Coin, Dogecoin (DOGE) Has Been Struggling
- 2025-04-19 14:20:13
- Market Update: Crypto Market Remains Steady at $2.79 Trillion with Minimal 0.14% Growth
- 2025-04-19 14:15:13
- Can Mantra (OM) Bounce Back? How High Can the Price Go After 90% Crash?
- 2025-04-19 14:15:13
- President Donald Trump Said at a 2024 Campaign Event That He Wanted All Remaining Bitcoin
- 2025-04-19 14:10:13
- Qubetics ($TICS): The New Frontier in Blockchain Interoperability
- 2025-04-19 14:10:13
Related knowledge
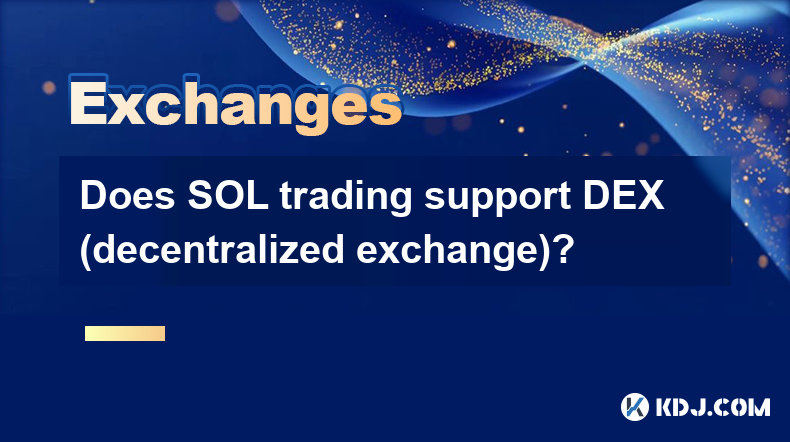
Does SOL trading support DEX (decentralized exchange)?
Apr 19,2025 at 05:21am
Solana (SOL), a high-performance blockchain platform, has gained significant attention in the cryptocurrency community for its fast transaction speeds and low fees. One of the key aspects that traders and investors often inquire about is whether SOL trading supports decentralized exchanges (DEXs). In this article, we will explore this topic in detail, p...
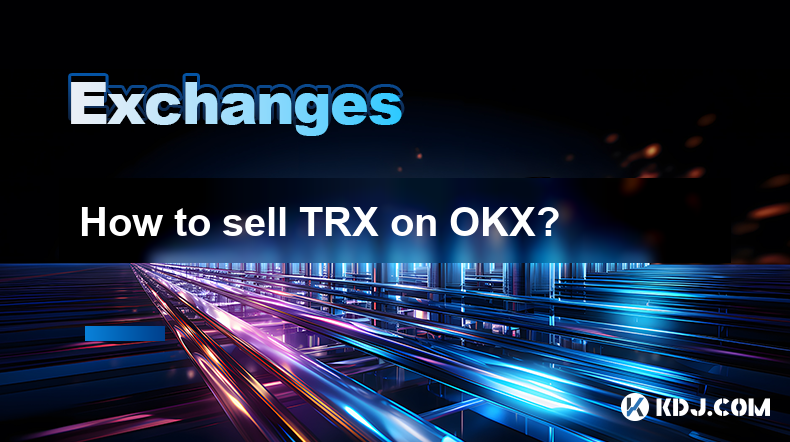
How to sell TRX on OKX?
Apr 18,2025 at 11:07pm
Selling TRX on OKX is a straightforward process that can be completed in a few simple steps. This article will guide you through the entire process, ensuring that you understand each step thoroughly. Whether you are a beginner or an experienced trader, this guide will help you navigate the OKX platform with ease. Preparing to Sell TRX on OKXBefore you c...
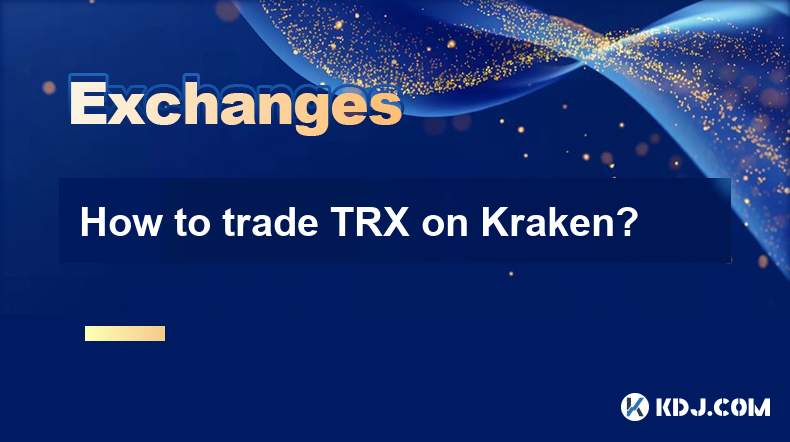
How to trade TRX on Kraken?
Apr 19,2025 at 02:00am
Trading TRX on Kraken involves several steps, from setting up your account to executing your first trade. Here's a detailed guide on how to get started and successfully trade TRX on the Kraken platform. Setting Up Your Kraken AccountBefore you can start trading TRX on Kraken, you need to set up an account. Here's how to do it: Visit the Kraken website a...
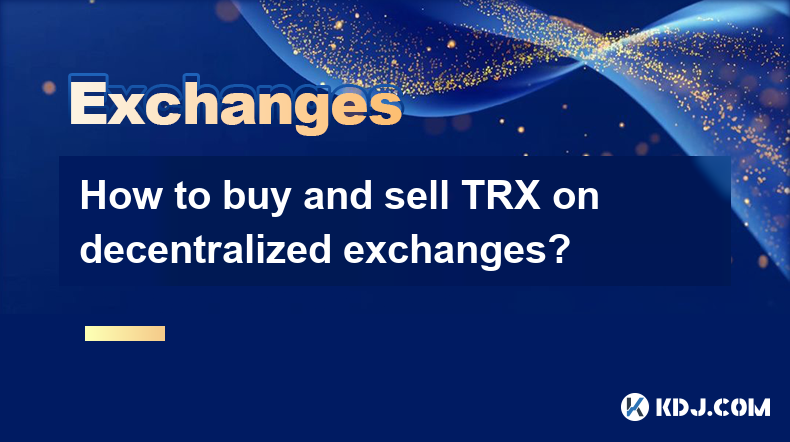
How to buy and sell TRX on decentralized exchanges?
Apr 18,2025 at 08:08pm
Introduction to TRX and Decentralized ExchangesTRX, or Tron, is a popular cryptocurrency that aims to build a decentralized internet and entertainment ecosystem. Decentralized exchanges (DEXs) offer a way to trade cryptocurrencies like TRX without the need for a central authority, providing greater privacy and control over your funds. In this article, w...
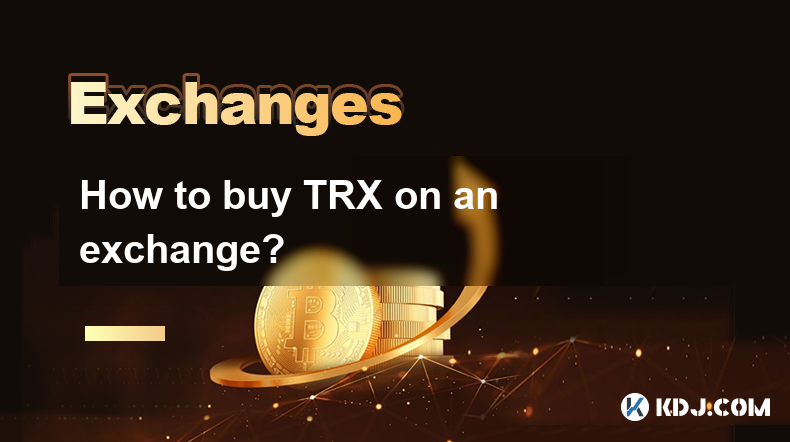
How to buy TRX on an exchange?
Apr 19,2025 at 12:08pm
Buying TRX, the native cryptocurrency of the Tron network, on an exchange is a straightforward process that involves several key steps. This guide will walk you through the process of purchasing TRX, ensuring you understand each step thoroughly. Choosing a Reliable ExchangeBefore you can buy TRX, you need to select a reputable cryptocurrency exchange th...
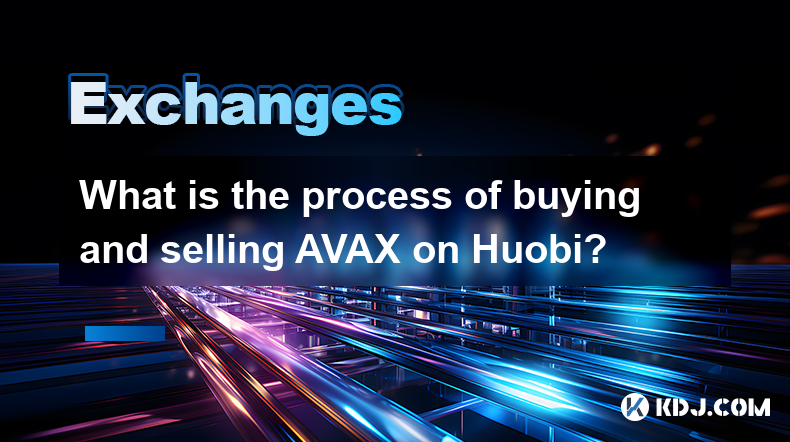
What is the process of buying and selling AVAX on Huobi?
Apr 18,2025 at 07:50pm
Understanding AVAX and Huobi Before diving into the process of buying and selling AVAX on Huobi, it's essential to understand what these terms mean. AVAX is the native cryptocurrency of the Avalanche blockchain, a platform designed for decentralized applications and custom blockchain networks. Huobi, on the other hand, is a leading global cryptocurrency...
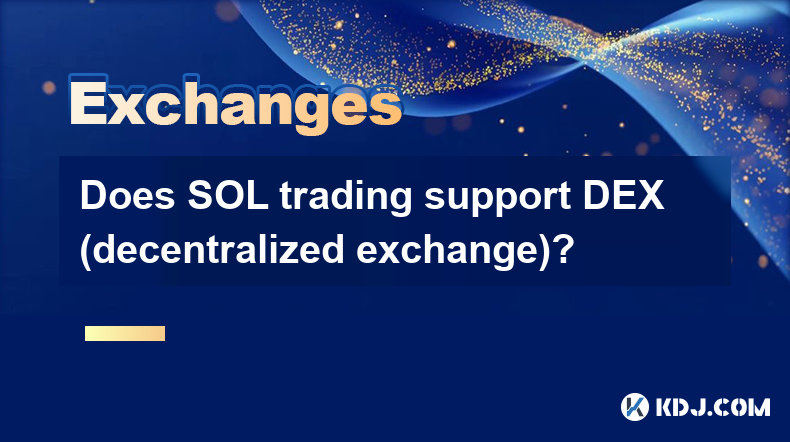
Does SOL trading support DEX (decentralized exchange)?
Apr 19,2025 at 05:21am
Solana (SOL), a high-performance blockchain platform, has gained significant attention in the cryptocurrency community for its fast transaction speeds and low fees. One of the key aspects that traders and investors often inquire about is whether SOL trading supports decentralized exchanges (DEXs). In this article, we will explore this topic in detail, p...
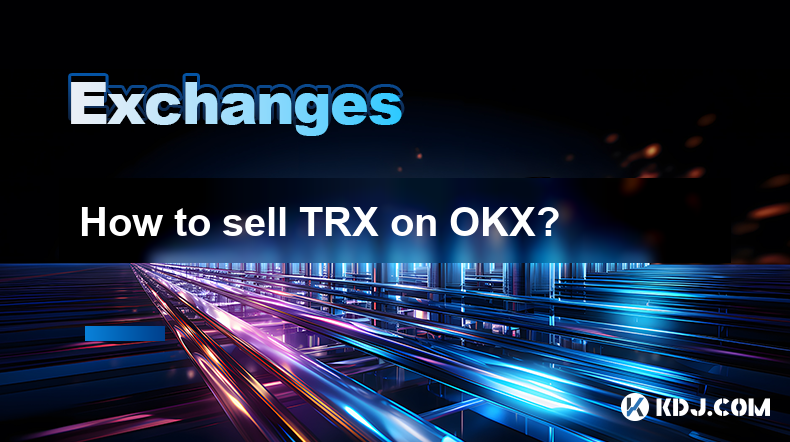
How to sell TRX on OKX?
Apr 18,2025 at 11:07pm
Selling TRX on OKX is a straightforward process that can be completed in a few simple steps. This article will guide you through the entire process, ensuring that you understand each step thoroughly. Whether you are a beginner or an experienced trader, this guide will help you navigate the OKX platform with ease. Preparing to Sell TRX on OKXBefore you c...
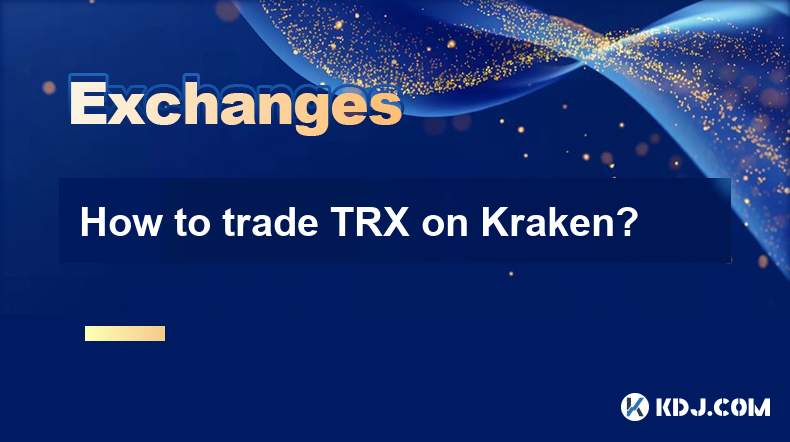
How to trade TRX on Kraken?
Apr 19,2025 at 02:00am
Trading TRX on Kraken involves several steps, from setting up your account to executing your first trade. Here's a detailed guide on how to get started and successfully trade TRX on the Kraken platform. Setting Up Your Kraken AccountBefore you can start trading TRX on Kraken, you need to set up an account. Here's how to do it: Visit the Kraken website a...
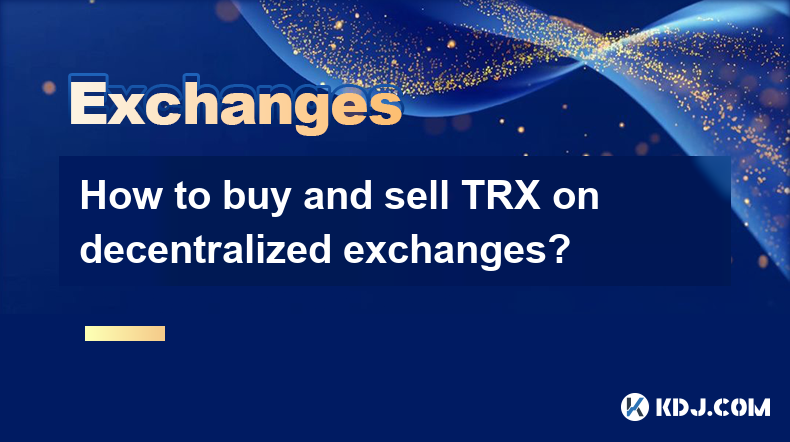
How to buy and sell TRX on decentralized exchanges?
Apr 18,2025 at 08:08pm
Introduction to TRX and Decentralized ExchangesTRX, or Tron, is a popular cryptocurrency that aims to build a decentralized internet and entertainment ecosystem. Decentralized exchanges (DEXs) offer a way to trade cryptocurrencies like TRX without the need for a central authority, providing greater privacy and control over your funds. In this article, w...
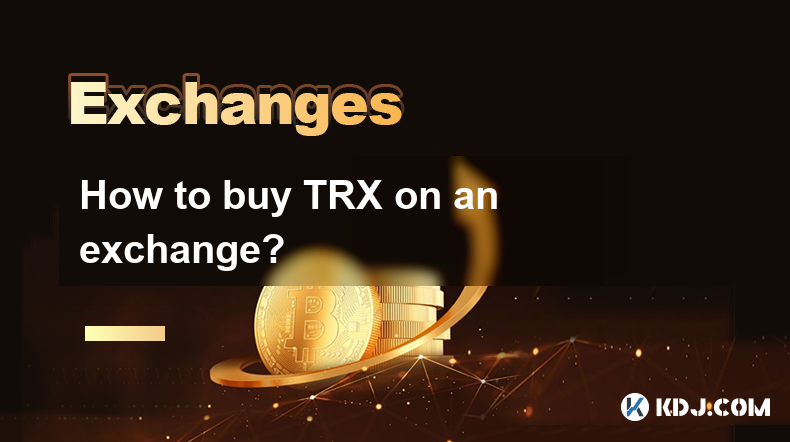
How to buy TRX on an exchange?
Apr 19,2025 at 12:08pm
Buying TRX, the native cryptocurrency of the Tron network, on an exchange is a straightforward process that involves several key steps. This guide will walk you through the process of purchasing TRX, ensuring you understand each step thoroughly. Choosing a Reliable ExchangeBefore you can buy TRX, you need to select a reputable cryptocurrency exchange th...
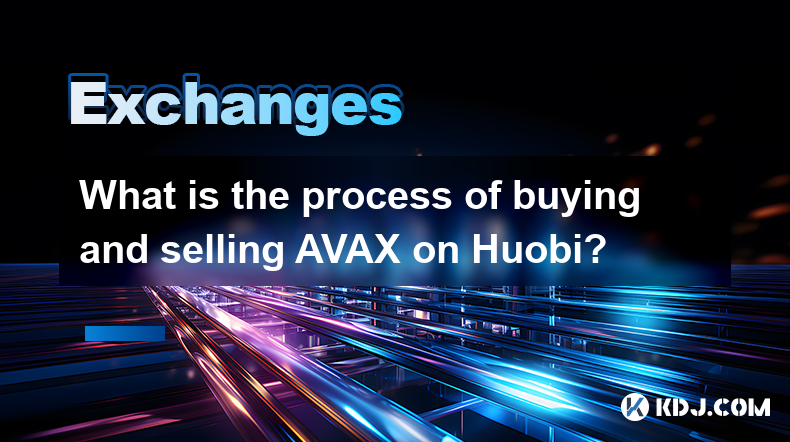
What is the process of buying and selling AVAX on Huobi?
Apr 18,2025 at 07:50pm
Understanding AVAX and Huobi Before diving into the process of buying and selling AVAX on Huobi, it's essential to understand what these terms mean. AVAX is the native cryptocurrency of the Avalanche blockchain, a platform designed for decentralized applications and custom blockchain networks. Huobi, on the other hand, is a leading global cryptocurrency...
See all articles
