-
Bitcoin
$85,236.8618
0.90% -
Ethereum
$1,615.9773
1.41% -
Tether USDt
$0.9996
-0.02% -
XRP
$2.0883
0.66% -
BNB
$591.2394
-0.31% -
Solana
$138.7789
3.57% -
USDC
$0.9997
-0.02% -
Dogecoin
$0.1571
-0.57% -
TRON
$0.2426
0.82% -
Cardano
$0.6304
0.44% -
UNUS SED LEO
$9.3083
0.85% -
Chainlink
$12.9679
2.88% -
Avalanche
$20.2445
5.87% -
Stellar
$0.2481
3.09% -
Toncoin
$2.9678
-0.99% -
Shiba Inu
$0.0...01230
0.25% -
Hedera
$0.1667
0.48% -
Sui
$2.1626
1.33% -
Bitcoin Cash
$334.6897
-2.24% -
Hyperliquid
$18.0279
6.84% -
Polkadot
$3.8084
3.15% -
Litecoin
$76.0021
-0.32% -
Bitget Token
$4.5255
2.93% -
Dai
$0.9999
-0.01% -
Ethena USDe
$0.9992
-0.02% -
Pi
$0.6488
4.29% -
Monero
$211.1432
-2.44% -
Uniswap
$5.3268
2.64% -
Pepe
$0.0...07424
2.66% -
OKB
$50.9311
0.82%
How to use Bithumb's API?
Using Bithumb's API can enhance trading strategies and automate processes; this guide covers registration, API key setup, and making your first API call effectively.
Apr 18, 2025 at 08:49 pm
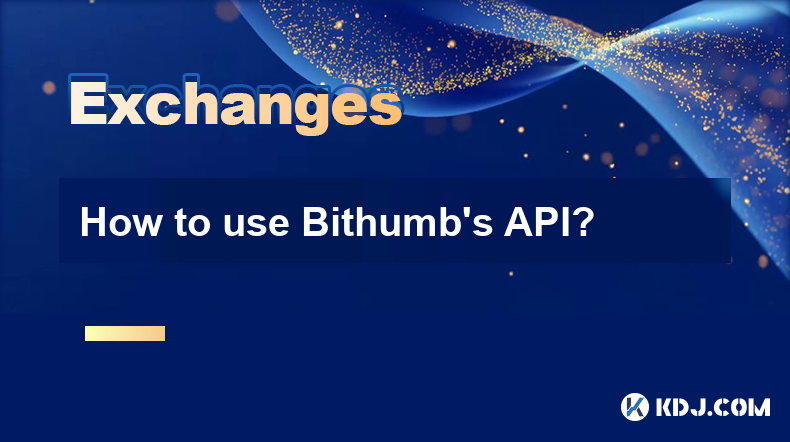
Using Bithumb's API can significantly enhance your trading strategies, automate processes, and access real-time data. In this guide, we will walk you through the steps to use Bithumb's API effectively, covering everything from registration to executing your first API call.
Registering and Setting Up an API Key
Before you can use Bithumb's API, you need to register for an account and set up an API key. Here's how you can do it:
- Visit the Bithumb website and sign up for a new account if you don't already have one. You'll need to provide your personal details and complete the verification process.
- Log into your Bithumb account. Navigate to the API Management section, which is usually found under the account settings or security settings.
- Create a new API key. You will be prompted to enter a name for your API key, which helps you manage multiple keys if needed. Make sure to enable the necessary permissions based on what you intend to do with the API.
- Generate the API key. You will receive an API Key and an API Secret. These are crucial for authenticating your API requests, so keep them secure.
Understanding Bithumb's API Endpoints
Bithumb's API is divided into several endpoints, each serving a specific purpose. Here are some key endpoints you should know:
- Public API: These endpoints do not require authentication and are used to fetch market data, such as ticker information, order books, and transaction history.
- Private API: These endpoints require authentication and allow you to perform actions like placing orders, canceling orders, and managing your account balance.
Making Your First API Call
To make your first API call, you'll need to use a programming language like Python, JavaScript, or any other language that supports HTTP requests. Here's a step-by-step guide using Python:
- Install the necessary libraries. You'll need
requests
to make HTTP requests andhmac
for signing your requests. You can install them using pip:
pip install requests
- Import the libraries in your Python script:
import requests
import hmac
import hashlib
import time
- Set up your API credentials:
api_key = 'YOUR_API_KEY'
api_secret = 'YOUR_API_SECRET'
- Construct the API call. Let's use the public API to fetch the ticker information for Bitcoin (BTC):
endpoint = 'https://api.bithumb.com/public/ticker/BTC'
response = requests.get(endpoint)
print(response.json())
- For private API calls, you need to sign your requests. Here's an example of how to place a buy order:
endpoint = 'https://api.bithumb.com/trade/place'
params = {'order_currency': 'BTC',
'payment_currency': 'KRW',
'units': '0.001',
'price': '1000000',
'type': 'bid'
}
params['endpoint'] = endpoint
nonce = str(int(time.time() * 1000))
params['nonce'] = nonce
Create the signature
query_string = '&'.join([f"{k}={v}" for k, v in sorted(params.items())])
signature = hmac.new(api_secret.encode('utf-8'), query_string.encode('utf-8'), hashlib.sha512).hexdigest()
headers = {
'Api-Key': api_key,
'Api-Sign': signature,
'Content-Type': 'application/x-www-form-urlencoded'
}
response = requests.post(endpoint, data=params, headers=headers)
print(response.json())
Handling Errors and Responses
When using Bithumb's API, it's important to handle errors and parse responses correctly. Here's how you can do it:
- Check the status code of the response. A status code of 200 indicates success, while other codes indicate errors.
- Parse the JSON response. Bithumb's API returns data in JSON format, which you can easily parse using Python's
json
module.
import jsonif response.status_code == 200:
data = response.json()
if data['status'] == '0000':
print("Success:", data)
else:
print("Error:", data['message'])
else:
print("HTTP Error:", response.status_code)
Using Bithumb's WebSocket API
For real-time data, Bithumb offers a WebSocket API. Here's how you can connect to it using Python:
- Install the
websocket-client
library:
pip install websocket-client
- Connect to the WebSocket:
import websocket
import json
def on_message(ws, message):
data = json.loads(message)
print(data)
def on_error(ws, error):
print(error)
def on_close(ws):
print("### closed ###")
def on_open(ws):
print("### opened ###")
websocket.enableTrace(True)
ws = websocket.WebSocketApp("wss://pubwss.bithumb.com/pub/ws",
on_message=on_message,
on_error=on_error,
on_close=on_close)
ws.on_open = on_open
ws.run_forever()
Best Practices for Using Bithumb's API
To ensure you use Bithumb's API effectively and securely, consider the following best practices:
- Secure your API keys. Never share your API keys or store them in version control systems. Use environment variables or secure vaults to store them.
- Rate limiting. Be mindful of Bithumb's rate limits to avoid having your API access restricted. Implement retry mechanisms with exponential backoff for handling rate limit errors.
- Error handling. Always include robust error handling in your code to deal with API failures gracefully.
- Testing. Before deploying your code to production, thoroughly test it in a sandbox or test environment to ensure it works as expected.
Frequently Asked Questions
Q: Can I use Bithumb's API for automated trading?
A: Yes, Bithumb's API supports automated trading through its private endpoints, allowing you to place orders, cancel orders, and manage your account balance programmatically.
Q: How often is the data updated on Bithumb's API?
A: The public API endpoints, such as ticker and order book data, are typically updated in real-time, while private API endpoints may have varying update frequencies based on your account's activity.
Q: Is there a limit to the number of API keys I can create on Bithumb?
A: Bithumb may have a limit on the number of API keys you can create per account. You should check the API documentation or contact Bithumb support for specific details.
Q: Can I use Bithumb's API to fetch historical data?
A: Bithumb's API primarily provides real-time data. For historical data, you might need to use third-party services or manually collect and store the data over time.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- The Crypto Market Is Heating Up Again—and It's Not Just Bitcoin or Ethereum This Time
- 2025-04-20 05:35:12
- After years of regulation-by-enforcement, the SEC is now open to public input on crypto policy
- 2025-04-20 05:35:12
- Bitcoin (BTC) Prices Hold Steady Near $83,200 as Thousands Protest Against Trump
- 2025-04-20 05:30:12
- While the Crypto Market Oscillates Without a Clear Direction, Some Internal Dynamics Are Reigniting Tensions
- 2025-04-20 05:30:12
- XploraDEX Presale Enters Its Final 48 Hours, with the Energy Electric Across the XRP Community
- 2025-04-20 05:25:12
- Justin Sun Hints at TRX ETF on SEC Website, Sparking Crypto Community Excitement
- 2025-04-20 05:25:12
Related knowledge
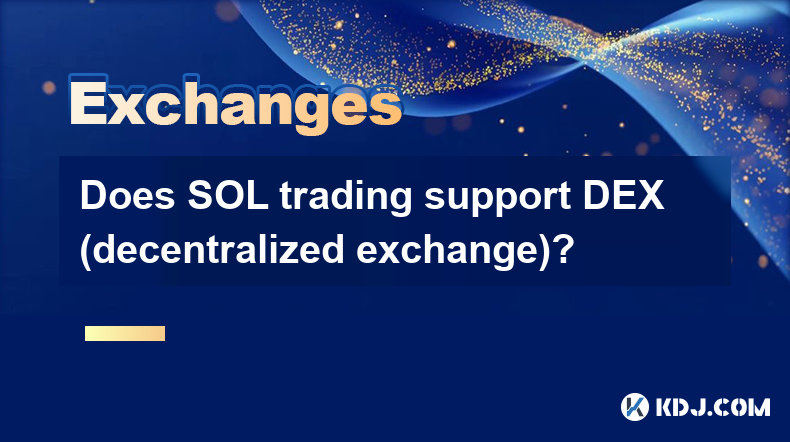
Does SOL trading support DEX (decentralized exchange)?
Apr 19,2025 at 05:21am
Solana (SOL), a high-performance blockchain platform, has gained significant attention in the cryptocurrency community for its fast transaction speeds and low fees. One of the key aspects that traders and investors often inquire about is whether SOL trading supports decentralized exchanges (DEXs). In this article, we will explore this topic in detail, p...
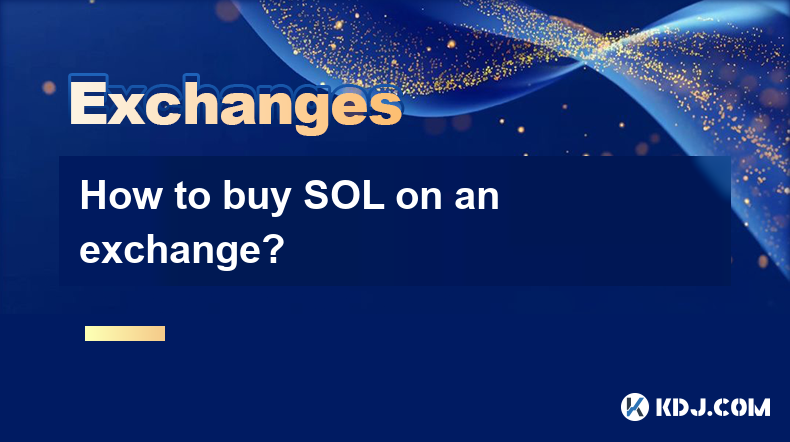
How to buy SOL on an exchange?
Apr 20,2025 at 01:21am
Introduction to Buying SOL on an ExchangeSOL, the native cryptocurrency of the Solana blockchain, has garnered significant attention in the crypto world due to its high throughput and low transaction costs. If you're interested in adding SOL to your investment portfolio, buying it on a cryptocurrency exchange is one of the most straightforward methods. ...
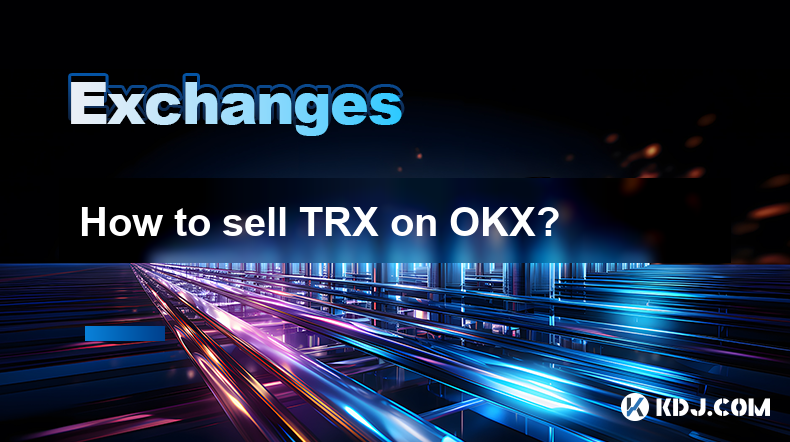
How to sell TRX on OKX?
Apr 18,2025 at 11:07pm
Selling TRX on OKX is a straightforward process that can be completed in a few simple steps. This article will guide you through the entire process, ensuring that you understand each step thoroughly. Whether you are a beginner or an experienced trader, this guide will help you navigate the OKX platform with ease. Preparing to Sell TRX on OKXBefore you c...
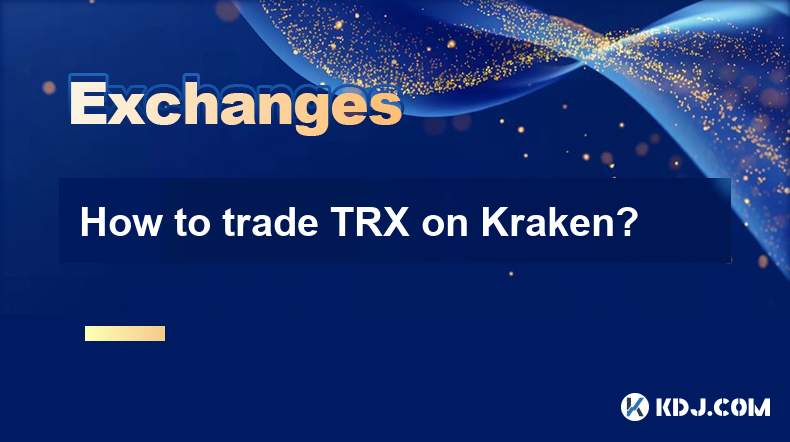
How to trade TRX on Kraken?
Apr 19,2025 at 02:00am
Trading TRX on Kraken involves several steps, from setting up your account to executing your first trade. Here's a detailed guide on how to get started and successfully trade TRX on the Kraken platform. Setting Up Your Kraken AccountBefore you can start trading TRX on Kraken, you need to set up an account. Here's how to do it: Visit the Kraken website a...
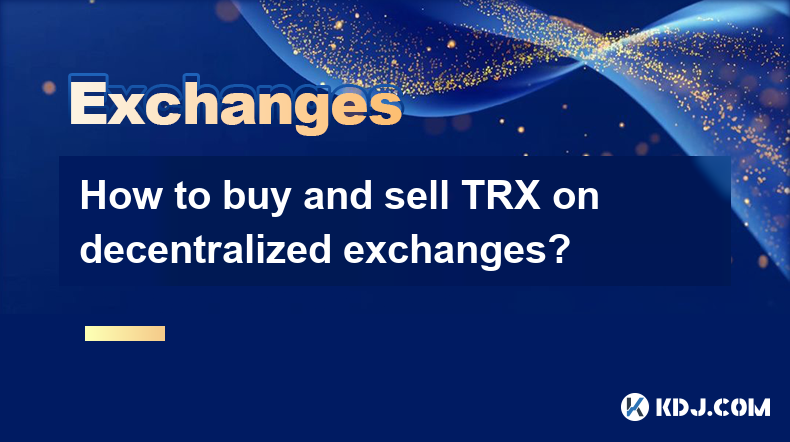
How to buy and sell TRX on decentralized exchanges?
Apr 18,2025 at 08:08pm
Introduction to TRX and Decentralized ExchangesTRX, or Tron, is a popular cryptocurrency that aims to build a decentralized internet and entertainment ecosystem. Decentralized exchanges (DEXs) offer a way to trade cryptocurrencies like TRX without the need for a central authority, providing greater privacy and control over your funds. In this article, w...
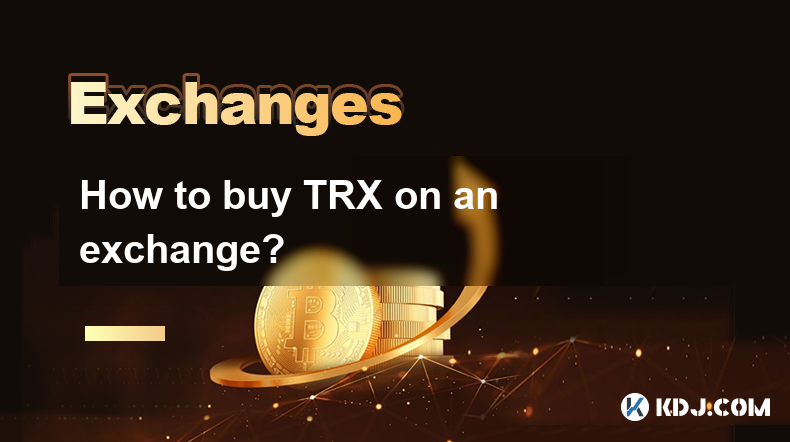
How to buy TRX on an exchange?
Apr 19,2025 at 12:08pm
Buying TRX, the native cryptocurrency of the Tron network, on an exchange is a straightforward process that involves several key steps. This guide will walk you through the process of purchasing TRX, ensuring you understand each step thoroughly. Choosing a Reliable ExchangeBefore you can buy TRX, you need to select a reputable cryptocurrency exchange th...
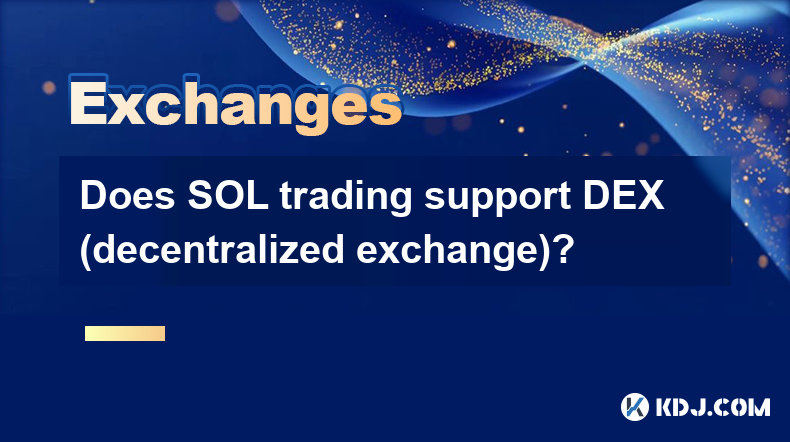
Does SOL trading support DEX (decentralized exchange)?
Apr 19,2025 at 05:21am
Solana (SOL), a high-performance blockchain platform, has gained significant attention in the cryptocurrency community for its fast transaction speeds and low fees. One of the key aspects that traders and investors often inquire about is whether SOL trading supports decentralized exchanges (DEXs). In this article, we will explore this topic in detail, p...
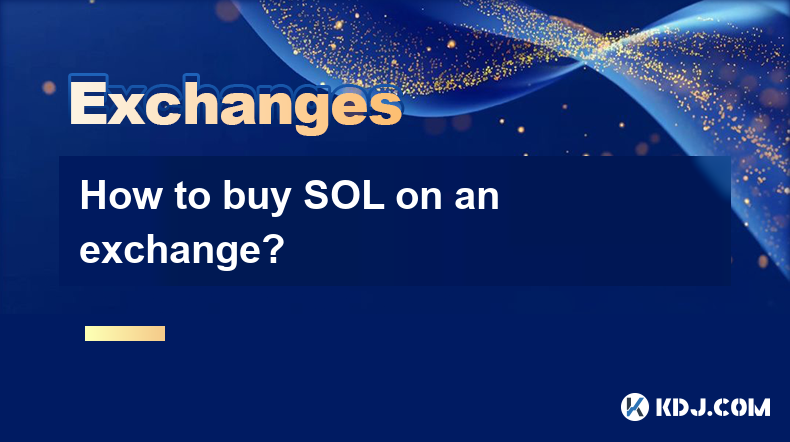
How to buy SOL on an exchange?
Apr 20,2025 at 01:21am
Introduction to Buying SOL on an ExchangeSOL, the native cryptocurrency of the Solana blockchain, has garnered significant attention in the crypto world due to its high throughput and low transaction costs. If you're interested in adding SOL to your investment portfolio, buying it on a cryptocurrency exchange is one of the most straightforward methods. ...
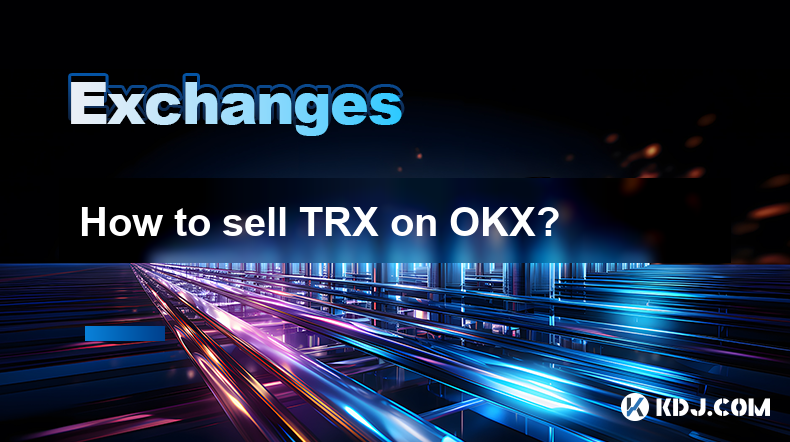
How to sell TRX on OKX?
Apr 18,2025 at 11:07pm
Selling TRX on OKX is a straightforward process that can be completed in a few simple steps. This article will guide you through the entire process, ensuring that you understand each step thoroughly. Whether you are a beginner or an experienced trader, this guide will help you navigate the OKX platform with ease. Preparing to Sell TRX on OKXBefore you c...
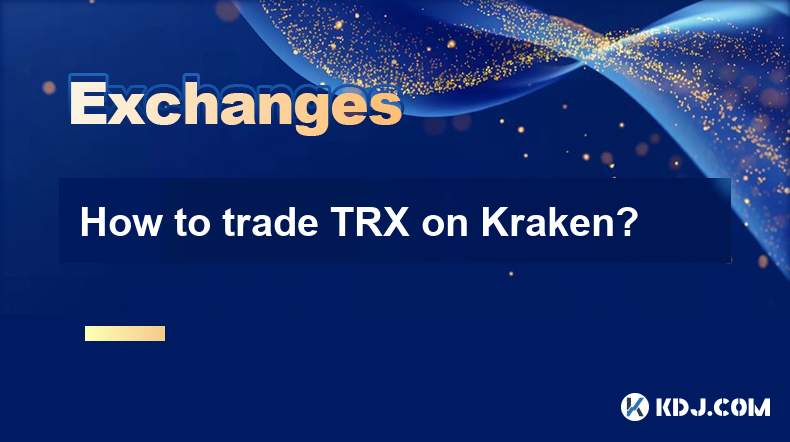
How to trade TRX on Kraken?
Apr 19,2025 at 02:00am
Trading TRX on Kraken involves several steps, from setting up your account to executing your first trade. Here's a detailed guide on how to get started and successfully trade TRX on the Kraken platform. Setting Up Your Kraken AccountBefore you can start trading TRX on Kraken, you need to set up an account. Here's how to do it: Visit the Kraken website a...
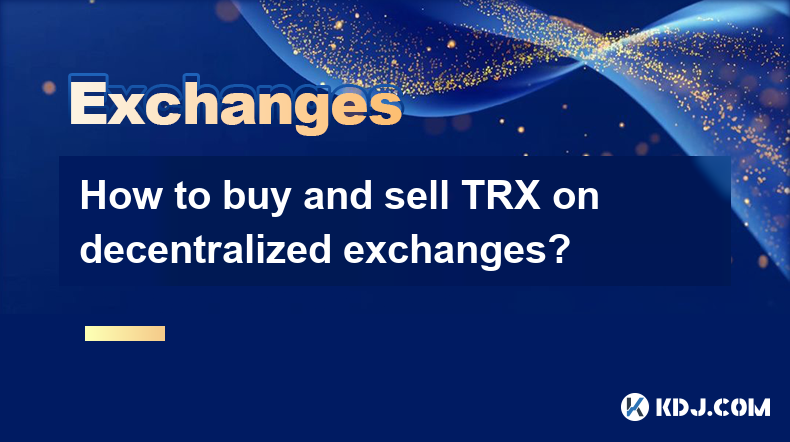
How to buy and sell TRX on decentralized exchanges?
Apr 18,2025 at 08:08pm
Introduction to TRX and Decentralized ExchangesTRX, or Tron, is a popular cryptocurrency that aims to build a decentralized internet and entertainment ecosystem. Decentralized exchanges (DEXs) offer a way to trade cryptocurrencies like TRX without the need for a central authority, providing greater privacy and control over your funds. In this article, w...
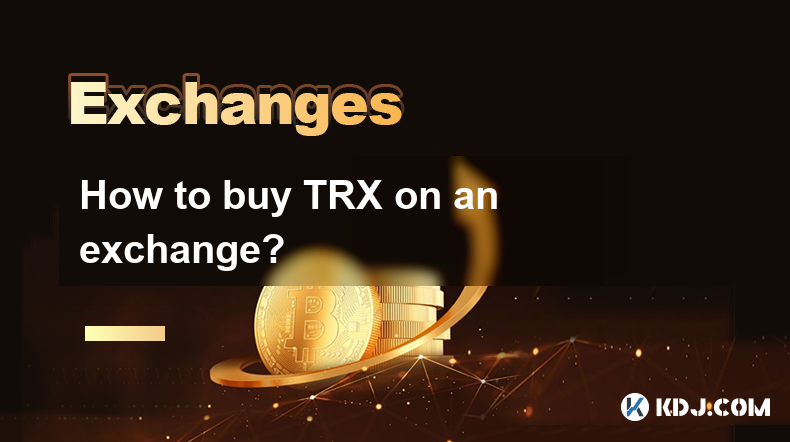
How to buy TRX on an exchange?
Apr 19,2025 at 12:08pm
Buying TRX, the native cryptocurrency of the Tron network, on an exchange is a straightforward process that involves several key steps. This guide will walk you through the process of purchasing TRX, ensuring you understand each step thoroughly. Choosing a Reliable ExchangeBefore you can buy TRX, you need to select a reputable cryptocurrency exchange th...
See all articles
