-
Bitcoin
$85,241.3100
0.88% -
Ethereum
$1,597.5221
0.89% -
Tether USDt
$0.9998
-0.01% -
XRP
$2.0841
0.61% -
BNB
$590.1959
0.22% -
Solana
$139.3082
4.16% -
USDC
$0.9999
-0.01% -
Dogecoin
$0.1580
1.99% -
TRON
$0.2418
-1.29% -
Cardano
$0.6272
2.42% -
UNUS SED LEO
$9.3579
2.37% -
Chainlink
$12.7797
1.30% -
Avalanche
$19.5621
2.86% -
Stellar
$0.2467
1.40% -
Toncoin
$2.9798
-0.36% -
Shiba Inu
$0.0...01214
1.76% -
Hedera
$0.1661
0.58% -
Sui
$2.1403
1.35% -
Bitcoin Cash
$337.7260
2.14% -
Hyperliquid
$18.0656
8.27% -
Polkadot
$3.7297
0.81% -
Litecoin
$76.0123
0.66% -
Dai
$0.9999
-0.01% -
Bitget Token
$4.4640
2.35% -
Ethena USDe
$0.9990
-0.01% -
Pi
$0.6448
5.54% -
Monero
$215.3607
-1.36% -
Uniswap
$5.2620
1.52% -
OKB
$50.8127
1.50% -
Pepe
$0.0...07190
1.14%
How to batch operate positions through API on OKX?
Batch operations on OKX via the API enable efficient management of multiple positions, automating trades and reducing errors for high-volume traders.
Apr 11, 2025 at 12:56 am
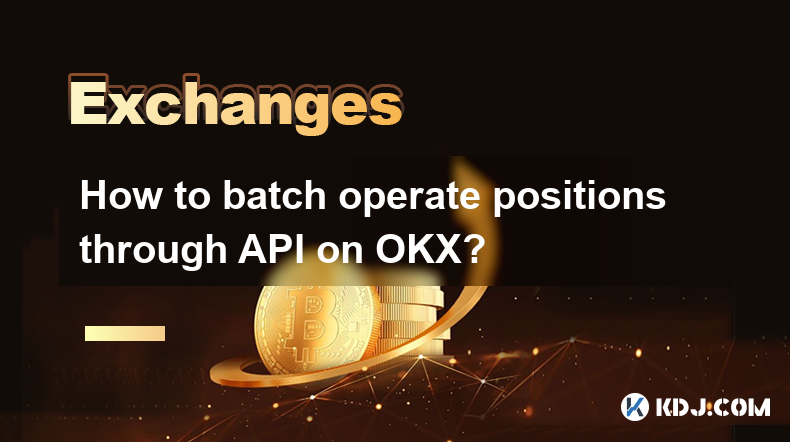
Introduction to Batch Operations on OKX
Batch operations on OKX allow users to manage multiple positions efficiently through the use of the OKX API. This functionality is particularly useful for traders who need to handle large volumes of trades or manage multiple positions simultaneously. By leveraging the OKX API, users can automate their trading strategies, reduce manual errors, and save time. In this article, we will explore how to batch operate positions through the OKX API, covering the necessary steps, tools, and considerations.
Understanding the OKX API
Before diving into batch operations, it's essential to understand the basics of the OKX API. The OKX API is a set of protocols and tools that allow developers to interact with the OKX platform programmatically. It supports various functions, including trading, account management, and data retrieval. To use the OKX API for batch operations, you will need to:
- Register for an OKX account and obtain API keys.
- Familiarize yourself with the API documentation, which provides detailed information on endpoints, parameters, and response formats.
- Set up a secure environment for API interactions, ensuring that your API keys are protected.
Setting Up Your API Environment
To begin batch operations, you need to set up your API environment. This involves:
- Generating API keys: Log into your OKX account, navigate to the API management section, and create a new API key. Ensure you set appropriate permissions for trading and account management.
- Securing your API keys: Store your API keys securely, preferably using environment variables or a secure vault. Never hardcode your keys in your scripts.
- Choosing a programming language: Select a language that supports HTTP requests and JSON parsing, such as Python, JavaScript, or Java. For this example, we will use Python.
Writing the Batch Operation Script
Once your environment is set up, you can start writing the script for batch operations. Here's a step-by-step guide to creating a Python script that can batch operate positions on OKX:
- Import necessary libraries: You will need libraries like
requests
for making HTTP requests andjson
for handling JSON data.
import requests
import json
import os
- Set up API credentials: Use environment variables to securely access your API keys.
api_key = os.environ.get('OKX_API_KEY')
api_secret = os.environ.get('OKX_API_SECRET')
api_passphrase = os.environ.get('OKX_API_PASSPHRASE')
- Define the function for batch operations: Create a function that can handle multiple positions. This function will take a list of positions and perform the desired operation (e.g., closing positions).
def batch_operate_positions(positions, operation):base_url = 'https://www.okx.com'
endpoint = '/api/v5/trade/close-position'
headers = {
'OK-ACCESS-KEY': api_key,
'OK-ACCESS-SIGN': '',
'OK-ACCESS-TIMESTAMP': '',
'OK-ACCESS-PASSPHRASE': api_passphrase,
'Content-Type': 'application/json'
}
for position in positions:
payload = {
'instId': position['instId'],
'mgnMode': position['mgnMode'],
'posSide': position['posSide']
}
# Generate the signature and timestamp
timestamp = str(int(time.time() * 1000))
headers['OK-ACCESS-TIMESTAMP'] = timestamp
pre_hash = timestamp + 'POST' + endpoint + json.dumps(payload)
signature = hmac.new(api_secret.encode('utf-8'), pre_hash.encode('utf-8'), hashlib.sha256).hexdigest()
headers['OK-ACCESS-SIGN'] = signature
response = requests.post(base_url + endpoint, headers=headers, data=json.dumps(payload))
if response.status_code == 200:
print(f"Successfully {operation} position: {position['instId']}")
else:
print(f"Failed to {operation} position: {position['instId']}. Error: {response.text}")
- Execute the batch operation: Call the function with a list of positions and the desired operation.
positions_to_close = [
{'instId': 'BTC-USDT-SWAP', 'mgnMode': 'cross', 'posSide': 'long'},
{'instId': 'ETH-USDT-SWAP', 'mgnMode': 'cross', 'posSide': 'short'}
]
batch_operate_positions(positions_to_close, 'close')
Handling Errors and Exceptions
When performing batch operations, it's crucial to handle errors and exceptions gracefully. Here are some tips:
- Implement retry logic: If a request fails, implement a retry mechanism with exponential backoff to handle temporary network issues.
- Log errors: Keep a detailed log of all operations, including successful and failed requests, to aid in troubleshooting.
- Validate inputs: Ensure that the positions you are trying to operate on are valid and exist in your account.
Testing and Validation
Before running batch operations on live positions, it's essential to test and validate your script. Here are some steps to follow:
- Use a testnet: OKX provides a testnet environment where you can simulate trades without risking real funds. Use this to test your script thoroughly.
- Start with small batches: Initially, operate on a small number of positions to ensure everything works as expected.
- Monitor and adjust: Continuously monitor the results of your batch operations and make adjustments as needed.
Security Considerations
Security is paramount when dealing with API operations. Here are some best practices to follow:
- Use HTTPS: Ensure all communications with the OKX API are over HTTPS to prevent man-in-the-middle attacks.
- Limit API key permissions: Only grant the necessary permissions to your API keys. For example, if you only need to close positions, do not enable withdrawal permissions.
- Rotate API keys: Regularly rotate your API keys to minimize the risk of unauthorized access.
Frequently Asked Questions
Q: Can I use the OKX API for batch operations on different types of positions, such as futures and options?
A: Yes, the OKX API supports batch operations on various types of positions, including futures, options, and swaps. You need to ensure that the instId
parameter in your payload matches the instrument ID of the position you want to operate on.
Q: How can I ensure that my batch operations are executed in a specific order?
A: The OKX API does not guarantee the order of execution for batch operations. To ensure a specific order, you can implement a sequential execution in your script, where each operation is performed one after the other, waiting for the previous operation to complete before starting the next.
Q: What should I do if I encounter rate limits while performing batch operations?
A: If you encounter rate limits, you should implement a delay between requests or use a queue system to manage your operations. OKX provides rate limit information in the API response headers, which you can use to adjust your script's behavior dynamically.
Q: Is it possible to batch operate positions across multiple accounts using the OKX API?
A: Yes, you can batch operate positions across multiple accounts by using different API keys for each account. However, you need to manage the API keys securely and ensure that each key has the appropriate permissions for the operations you want to perform.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- Renowned Author Robert Kiyosaki Forecasts Bitcoin (BTC) Price Could Skyrocket to $1 Million by 2035
- 2025-04-19 19:30:13
- A Modest Investment of $500 Today Could Potentially Grow into $2 Million by 2026
- 2025-04-19 19:30:13
- Bitcoin (BTC) and Ethereum (ETH) Options Expiry Today: Brace for Volatility
- 2025-04-19 19:25:14
- Ethereum Today Reacts To Base Tweet Frenzy
- 2025-04-19 19:25:14
- Michael Saylor's Bitcoin Playbook
- 2025-04-19 19:20:15
- Bitcoin (BTC) and Ethereum (ETH) ETFs Show Divergent Flows As BTC ETFs Gain 1,147 BTC
- 2025-04-19 19:20:15
Related knowledge
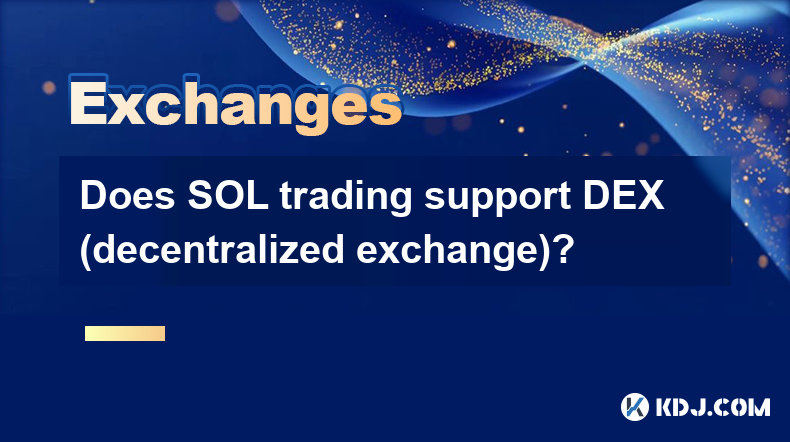
Does SOL trading support DEX (decentralized exchange)?
Apr 19,2025 at 05:21am
Solana (SOL), a high-performance blockchain platform, has gained significant attention in the cryptocurrency community for its fast transaction speeds and low fees. One of the key aspects that traders and investors often inquire about is whether SOL trading supports decentralized exchanges (DEXs). In this article, we will explore this topic in detail, p...
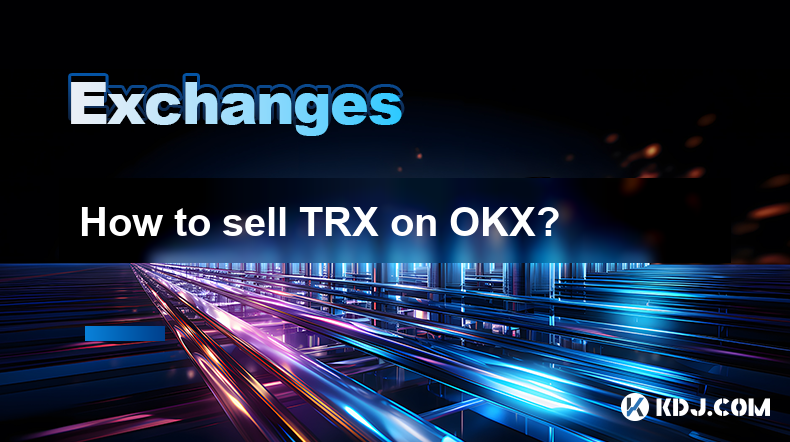
How to sell TRX on OKX?
Apr 18,2025 at 11:07pm
Selling TRX on OKX is a straightforward process that can be completed in a few simple steps. This article will guide you through the entire process, ensuring that you understand each step thoroughly. Whether you are a beginner or an experienced trader, this guide will help you navigate the OKX platform with ease. Preparing to Sell TRX on OKXBefore you c...
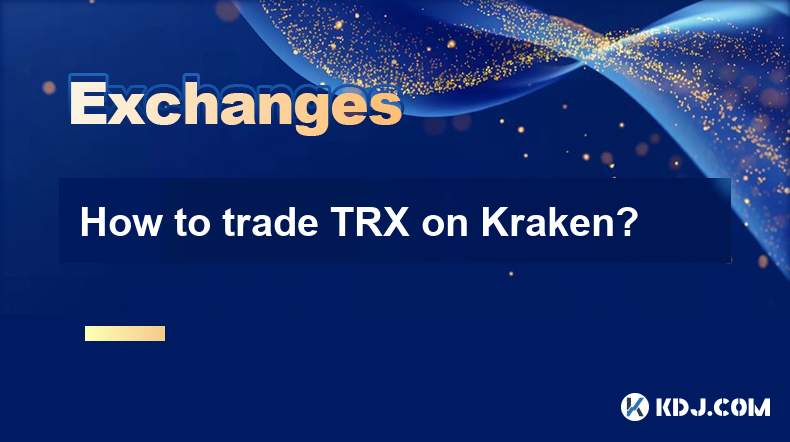
How to trade TRX on Kraken?
Apr 19,2025 at 02:00am
Trading TRX on Kraken involves several steps, from setting up your account to executing your first trade. Here's a detailed guide on how to get started and successfully trade TRX on the Kraken platform. Setting Up Your Kraken AccountBefore you can start trading TRX on Kraken, you need to set up an account. Here's how to do it: Visit the Kraken website a...
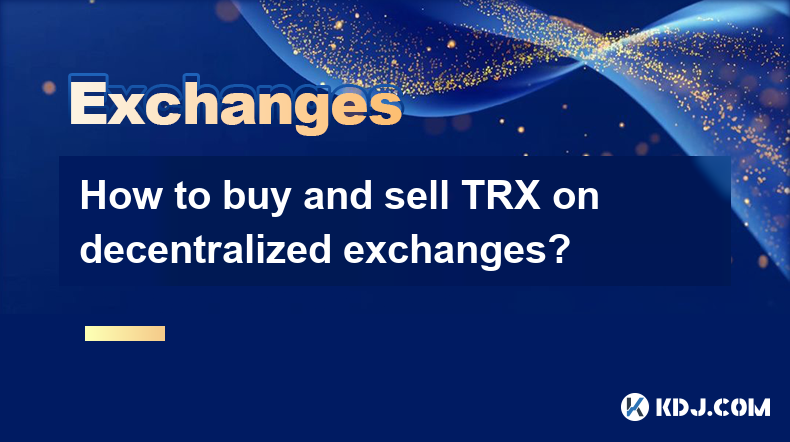
How to buy and sell TRX on decentralized exchanges?
Apr 18,2025 at 08:08pm
Introduction to TRX and Decentralized ExchangesTRX, or Tron, is a popular cryptocurrency that aims to build a decentralized internet and entertainment ecosystem. Decentralized exchanges (DEXs) offer a way to trade cryptocurrencies like TRX without the need for a central authority, providing greater privacy and control over your funds. In this article, w...
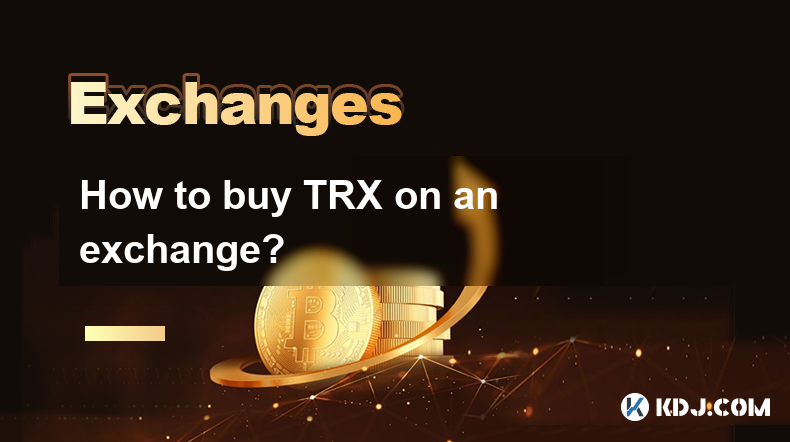
How to buy TRX on an exchange?
Apr 19,2025 at 12:08pm
Buying TRX, the native cryptocurrency of the Tron network, on an exchange is a straightforward process that involves several key steps. This guide will walk you through the process of purchasing TRX, ensuring you understand each step thoroughly. Choosing a Reliable ExchangeBefore you can buy TRX, you need to select a reputable cryptocurrency exchange th...
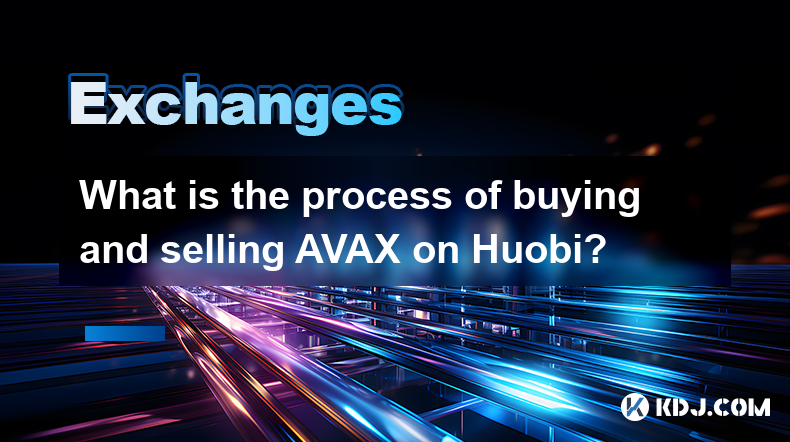
What is the process of buying and selling AVAX on Huobi?
Apr 18,2025 at 07:50pm
Understanding AVAX and Huobi Before diving into the process of buying and selling AVAX on Huobi, it's essential to understand what these terms mean. AVAX is the native cryptocurrency of the Avalanche blockchain, a platform designed for decentralized applications and custom blockchain networks. Huobi, on the other hand, is a leading global cryptocurrency...
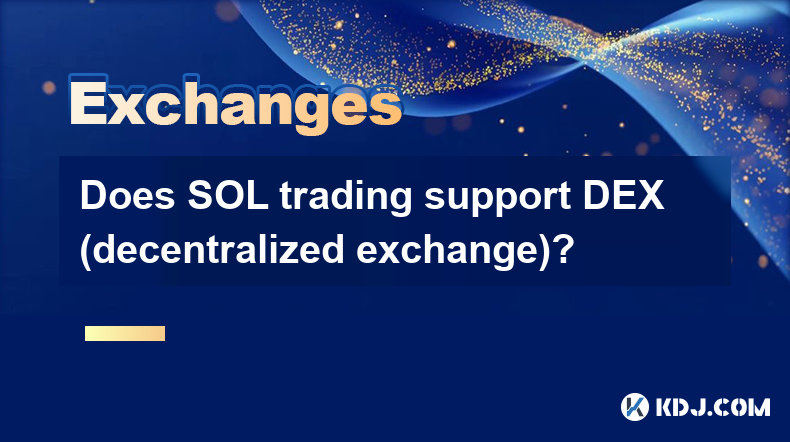
Does SOL trading support DEX (decentralized exchange)?
Apr 19,2025 at 05:21am
Solana (SOL), a high-performance blockchain platform, has gained significant attention in the cryptocurrency community for its fast transaction speeds and low fees. One of the key aspects that traders and investors often inquire about is whether SOL trading supports decentralized exchanges (DEXs). In this article, we will explore this topic in detail, p...
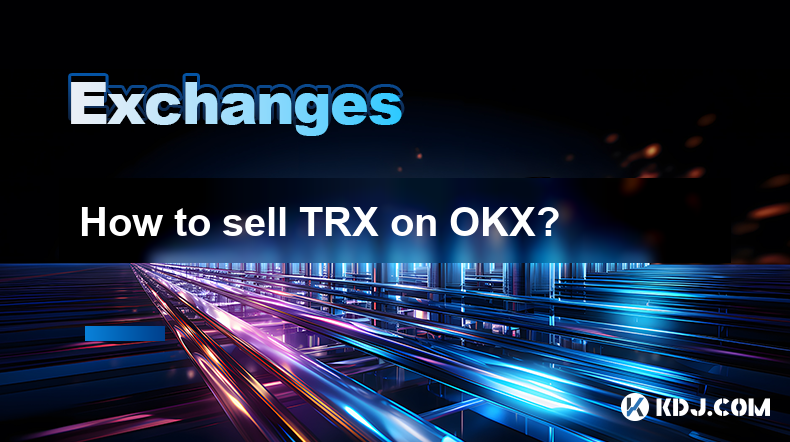
How to sell TRX on OKX?
Apr 18,2025 at 11:07pm
Selling TRX on OKX is a straightforward process that can be completed in a few simple steps. This article will guide you through the entire process, ensuring that you understand each step thoroughly. Whether you are a beginner or an experienced trader, this guide will help you navigate the OKX platform with ease. Preparing to Sell TRX on OKXBefore you c...
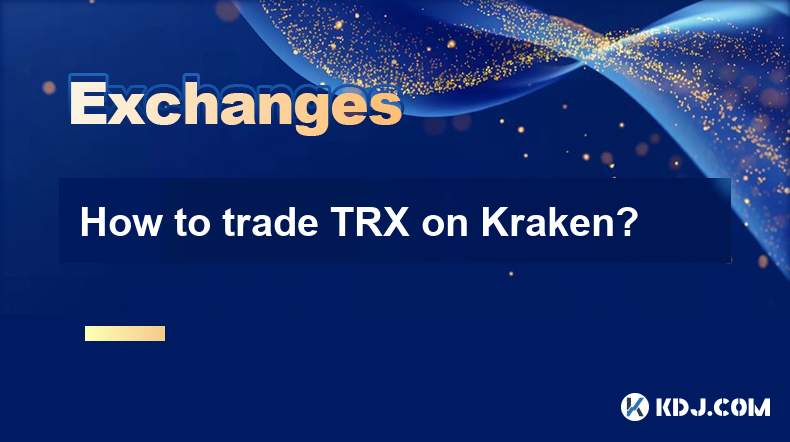
How to trade TRX on Kraken?
Apr 19,2025 at 02:00am
Trading TRX on Kraken involves several steps, from setting up your account to executing your first trade. Here's a detailed guide on how to get started and successfully trade TRX on the Kraken platform. Setting Up Your Kraken AccountBefore you can start trading TRX on Kraken, you need to set up an account. Here's how to do it: Visit the Kraken website a...
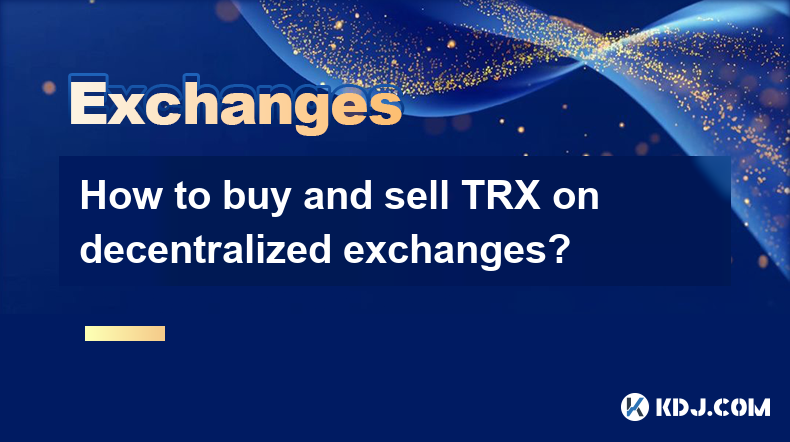
How to buy and sell TRX on decentralized exchanges?
Apr 18,2025 at 08:08pm
Introduction to TRX and Decentralized ExchangesTRX, or Tron, is a popular cryptocurrency that aims to build a decentralized internet and entertainment ecosystem. Decentralized exchanges (DEXs) offer a way to trade cryptocurrencies like TRX without the need for a central authority, providing greater privacy and control over your funds. In this article, w...
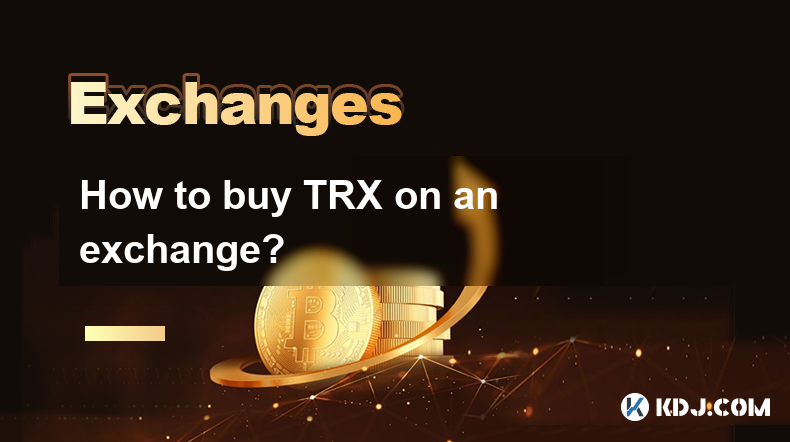
How to buy TRX on an exchange?
Apr 19,2025 at 12:08pm
Buying TRX, the native cryptocurrency of the Tron network, on an exchange is a straightforward process that involves several key steps. This guide will walk you through the process of purchasing TRX, ensuring you understand each step thoroughly. Choosing a Reliable ExchangeBefore you can buy TRX, you need to select a reputable cryptocurrency exchange th...
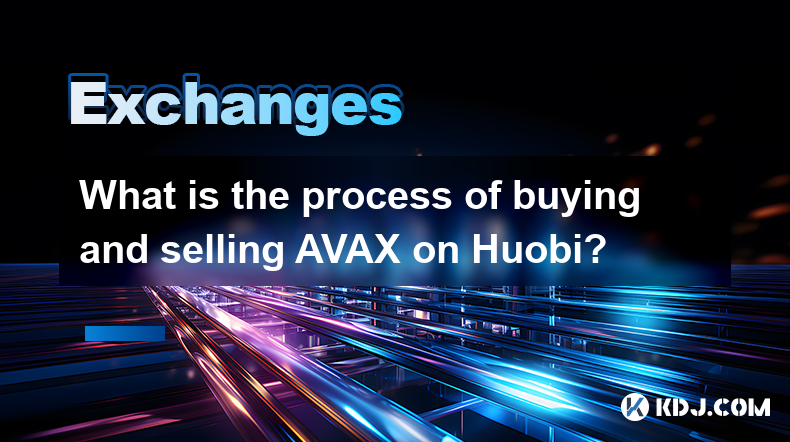
What is the process of buying and selling AVAX on Huobi?
Apr 18,2025 at 07:50pm
Understanding AVAX and Huobi Before diving into the process of buying and selling AVAX on Huobi, it's essential to understand what these terms mean. AVAX is the native cryptocurrency of the Avalanche blockchain, a platform designed for decentralized applications and custom blockchain networks. Huobi, on the other hand, is a leading global cryptocurrency...
See all articles
