-
Bitcoin
$87,160.3686
2.59% -
Ethereum
$1,577.5291
-0.58% -
Tether USDt
$1.0000
0.00% -
XRP
$2.0804
0.22% -
BNB
$596.3334
0.99% -
Solana
$136.5658
-0.15% -
USDC
$1.0000
0.00% -
Dogecoin
$0.1584
1.77% -
TRON
$0.2458
-0.32% -
Cardano
$0.6208
0.05% -
Chainlink
$13.1006
-1.96% -
UNUS SED LEO
$9.1412
-1.97% -
Avalanche
$19.9880
2.50% -
Stellar
$0.2528
3.56% -
Shiba Inu
$0.0...01238
-0.19% -
Toncoin
$2.8930
-3.79% -
Sui
$2.2031
4.44% -
Hedera
$0.1692
1.25% -
Bitcoin Cash
$343.2002
2.28% -
Polkadot
$3.8296
-1.99% -
Hyperliquid
$17.9378
1.39% -
Litecoin
$78.0777
0.75% -
Dai
$0.9999
0.00% -
Bitget Token
$4.4322
0.90% -
Ethena USDe
$0.9993
0.00% -
Pi
$0.6347
-0.88% -
Monero
$214.7110
-0.08% -
Uniswap
$5.2643
-0.22% -
Pepe
$0.0...07787
2.99% -
Aptos
$4.9970
-0.84%
How to use Upbit's WebSocket interface?
Upbit's WebSocket interface offers real-time market data, enabling quick trades; this guide covers setup, subscription, and data handling for efficient trading.
Apr 14, 2025 at 10:35 pm
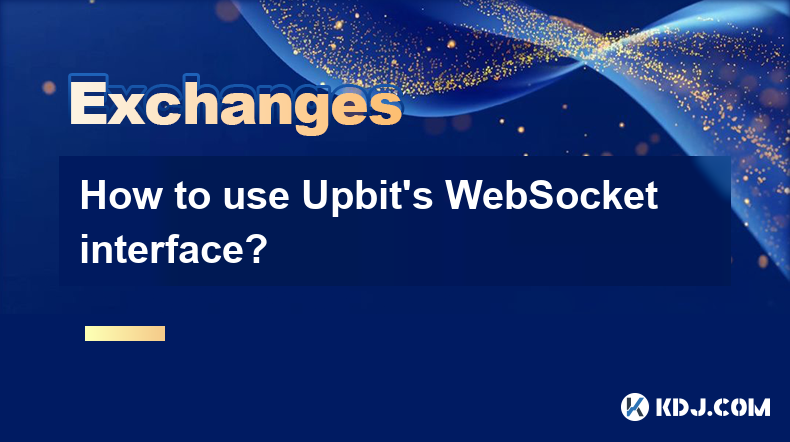
Using Upbit's WebSocket interface can significantly enhance your ability to receive real-time market data and execute trades with minimal delay. This article will guide you through the process of setting up and using Upbit's WebSocket interface, covering everything from initial connection to handling real-time data.
Understanding Upbit's WebSocket Interface
Upbit's WebSocket interface is designed to provide real-time market data, including order book updates, trade executions, and other critical information. Unlike RESTful APIs, which require periodic polling, WebSocket connections maintain a persistent link, allowing for immediate data transmission as events occur. This is particularly useful for applications requiring real-time updates, such as trading bots and market analysis tools.
Setting Up the WebSocket Connection
To establish a connection with Upbit's WebSocket server, you will need to use a WebSocket client library. Many programming languages offer such libraries, including JavaScript, Python, and Java. Here's how to set up a connection using Python's websocket-client
library:
- Install the WebSocket client library: You can do this by running
pip install websocket-client
in your terminal. - Import the necessary modules: In your Python script, add
import websocket
. - Define the WebSocket URL: Upbit's WebSocket URL is
wss://api.upbit.com/websocket/v1
. - Establish the connection: Use the
websocket.create_connection()
function to connect to the WebSocket URL.
Here is a sample code snippet to establish the connection:
import websocketws = websocket.create_connection("wss://api.upbit.com/websocket/v1")
Subscribing to Market Data
Once connected, you need to subscribe to the specific market data you are interested in. Upbit allows you to subscribe to various types of data, such as order book updates, trade ticks, and ticker data.
- Send a subscription request: After establishing the connection, send a JSON-formatted subscription request. For example, to subscribe to the order book of the BTC/KRW pair, you would send:
{
"type": "subscribe",
"channels": [{
"name": "orderbook",
"symbols": ["KRW-BTC"]
}
]
}
- Send the subscription request using Python: Use the
ws.send()
method to send the subscription request.
subscription = {
"type": "subscribe",
"channels": [{
"name": "orderbook",
"symbols": ["KRW-BTC"]
}
]
}
ws.send(json.dumps(subscription))
Handling Real-Time Data
Once subscribed, you will start receiving real-time data from Upbit. You need to set up a mechanism to process this data effectively.
- Set up a loop to receive messages: Use a loop to continuously receive messages from the WebSocket connection. In Python, you can use the
ws.recv()
method to receive data.
import jsonwhile True:
result = ws.recv()
data = json.loads(result)
print(data)
- Parse and process the received data: Depending on the type of data received, you will need to parse it and process it accordingly. For example, if you receive order book data, you might want to update your local order book representation.
Managing the Connection
Maintaining a stable WebSocket connection is crucial for real-time applications. Here are some tips for managing the connection:
- Implement reconnection logic: If the connection is lost, your application should attempt to reconnect. You can use a try-except block to handle connection errors and attempt to reconnect.
while True:
try:
ws = websocket.create_connection("wss://api.upbit.com/websocket/v1")
# Send subscription requests and handle data
except websocket.WebSocketException as e:
print(f"WebSocket error: {e}")
time.sleep(5) # Wait for 5 seconds before retrying
- Handle WebSocket ping/pong: Upbit's WebSocket server may send ping messages to keep the connection alive. Ensure your client responds to these pings with pong messages to maintain the connection.
Unsubscribing from Market Data
If you no longer need to receive certain data, you can unsubscribe from it. This helps in managing the data flow and reducing unnecessary network traffic.
- Send an unsubscribe request: Similar to subscribing, you need to send a JSON-formatted unsubscribe request. For example, to unsubscribe from the order book of the BTC/KRW pair, you would send:
{
"type": "unsubscribe",
"channels": [
{
"name": "orderbook",
"symbols": ["KRW-BTC"]
}
]
}
- Send the unsubscribe request using Python: Use the
ws.send()
method to send the unsubscribe request.
unsubscription = {
"type": "unsubscribe",
"channels": [{
"name": "orderbook",
"symbols": ["KRW-BTC"]
}
]
}
ws.send(json.dumps(unsubscription))
Closing the WebSocket Connection
When you are done using the WebSocket connection, it's important to close it properly to free up resources.
- Close the connection: Use the
ws.close()
method to close the WebSocket connection.
ws.close()
Frequently Asked Questions
Q: Can I subscribe to multiple markets at once using Upbit's WebSocket interface?
A: Yes, you can subscribe to multiple markets by including multiple symbols in your subscription request. For example, to subscribe to both BTC/KRW and ETH/KRW order books, you would send:
{
"type": "subscribe",
"channels": [{
"name": "orderbook",
"symbols": ["KRW-BTC", "KRW-ETH"]
}
]
}
Q: How can I handle rate limiting with Upbit's WebSocket interface?
A: Upbit's WebSocket interface does not have explicit rate limits like RESTful APIs. However, to avoid overwhelming the server, you should manage your subscriptions and data processing efficiently. If you encounter issues, consider reducing the number of subscriptions or implementing a backoff strategy.
Q: Is it possible to receive both trade and order book data through the same WebSocket connection?
A: Yes, you can subscribe to multiple types of data through the same WebSocket connection. For example, to receive both trade and order book data for BTC/KRW, you would send:
{
"type": "subscribe",
"channels": [{
"name": "orderbook",
"symbols": ["KRW-BTC"]
},
{
"name": "trade",
"symbols": ["KRW-BTC"]
}
]
}
Q: How can I ensure my WebSocket connection remains stable over long periods?
A: To ensure stability, implement reconnection logic to handle disconnections, manage WebSocket ping/pong messages to keep the connection alive, and monitor your application's performance to avoid resource exhaustion.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- Time to Buy This PEPE and Shiba Inu Rival Before Price Explodes? Presale Goes Live, Combines AI + Football Narrative!
- 2025-04-22 04:30:13
- 72 crypto-related ETFs are currently waiting for approval from the U.S. Securities and Exchange Commission (SEC)
- 2025-04-22 04:30:13
- title: MANTRA Founder and CEO John Patrick Mullin Will Burn 150M OM Tokens Worth $82M to Rebuild Trust
- 2025-04-22 04:25:13
- MANTRA Burns 150 Million $OM Tokens to Boost Its Project Following a Sharp Market Decline
- 2025-04-22 04:25:13
- The cryptocurrency XRP is about to wake up from its slumber. An extreme tightening of the Bollinger Bands warns analysts: a volatility explosion is brewing.
- 2025-04-22 04:20:13
- Most Bitcoin Companies in El Salvador Are Now Dormant
- 2025-04-22 04:20:13
Related knowledge
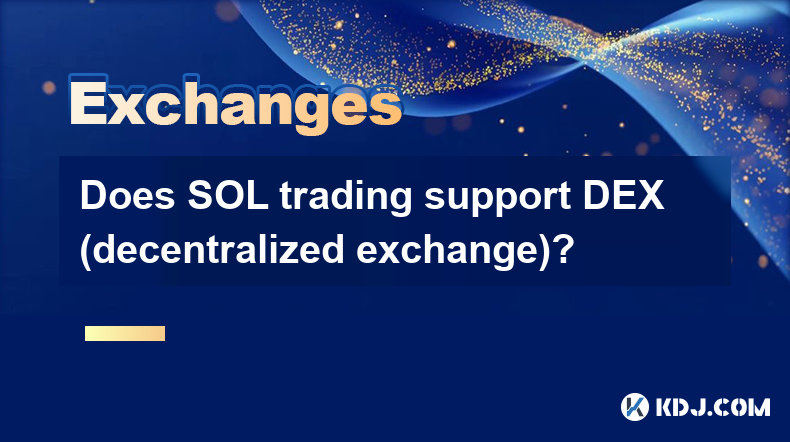
Does SOL trading support DEX (decentralized exchange)?
Apr 19,2025 at 05:21am
Solana (SOL), a high-performance blockchain platform, has gained significant attention in the cryptocurrency community for its fast transaction speeds and low fees. One of the key aspects that traders and investors often inquire about is whether SOL trading supports decentralized exchanges (DEXs). In this article, we will explore this topic in detail, p...
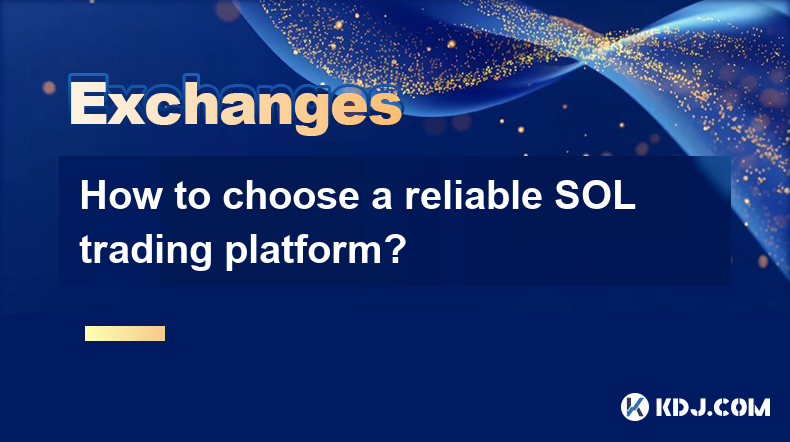
How to choose a reliable SOL trading platform?
Apr 21,2025 at 12:07am
Choosing a reliable SOL trading platform is crucial for anyone looking to engage in trading Solana (SOL) cryptocurrency. With the growing popularity of Solana, numerous platforms have emerged, each offering different features and levels of security. This article will guide you through the essential factors to consider when selecting a reliable SOL tradi...
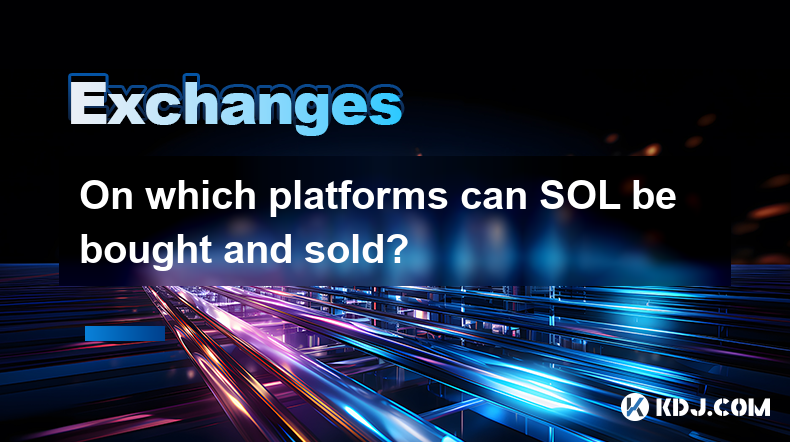
On which platforms can SOL be bought and sold?
Apr 21,2025 at 10:22am
Solana (SOL) is a popular cryptocurrency known for its high transaction speeds and low fees, making it a favored choice among crypto enthusiasts. If you're looking to buy or sell SOL, there are several platforms where you can do so. In this article, we will explore the various platforms that support the trading of SOL, ensuring you have a comprehensive ...
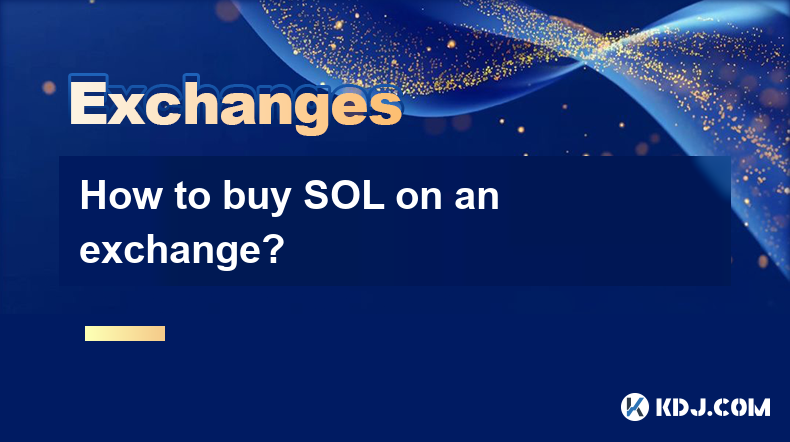
How to buy SOL on an exchange?
Apr 20,2025 at 01:21am
Introduction to Buying SOL on an ExchangeSOL, the native cryptocurrency of the Solana blockchain, has garnered significant attention in the crypto world due to its high throughput and low transaction costs. If you're interested in adding SOL to your investment portfolio, buying it on a cryptocurrency exchange is one of the most straightforward methods. ...
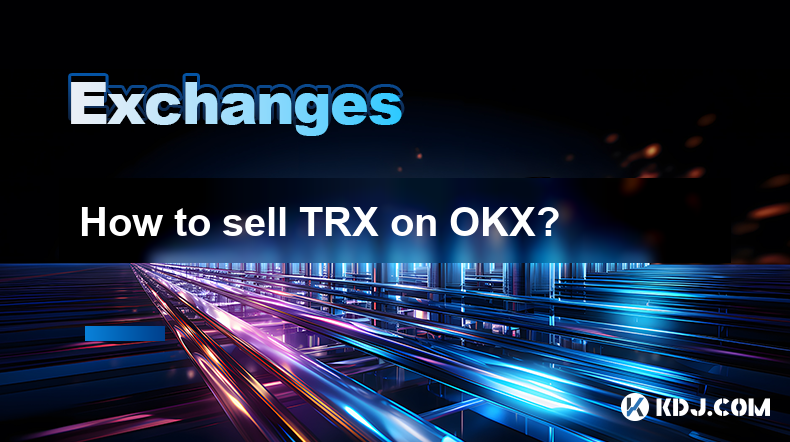
How to sell TRX on OKX?
Apr 18,2025 at 11:07pm
Selling TRX on OKX is a straightforward process that can be completed in a few simple steps. This article will guide you through the entire process, ensuring that you understand each step thoroughly. Whether you are a beginner or an experienced trader, this guide will help you navigate the OKX platform with ease. Preparing to Sell TRX on OKXBefore you c...
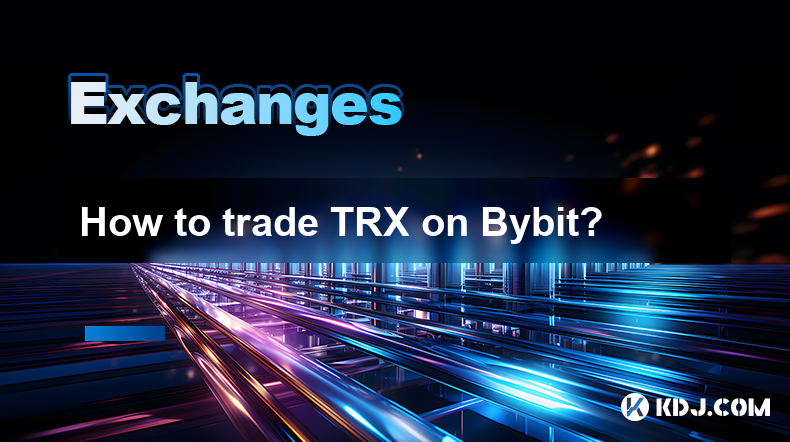
How to trade TRX on Bybit?
Apr 20,2025 at 04:15pm
Trading TRX on Bybit can be an exciting venture for both new and experienced cryptocurrency traders. Bybit, known for its robust trading platform and user-friendly interface, offers a variety of features that can help you trade TRX effectively. In this guide, we'll walk you through the essential steps and tips to successfully trade TRX on Bybit. Setting...
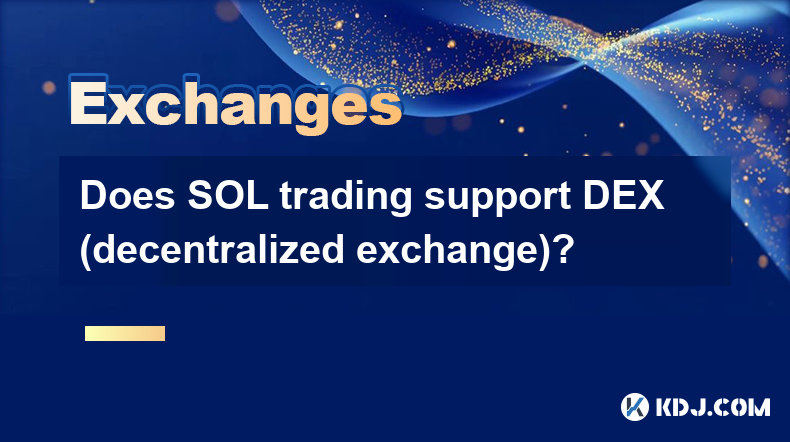
Does SOL trading support DEX (decentralized exchange)?
Apr 19,2025 at 05:21am
Solana (SOL), a high-performance blockchain platform, has gained significant attention in the cryptocurrency community for its fast transaction speeds and low fees. One of the key aspects that traders and investors often inquire about is whether SOL trading supports decentralized exchanges (DEXs). In this article, we will explore this topic in detail, p...
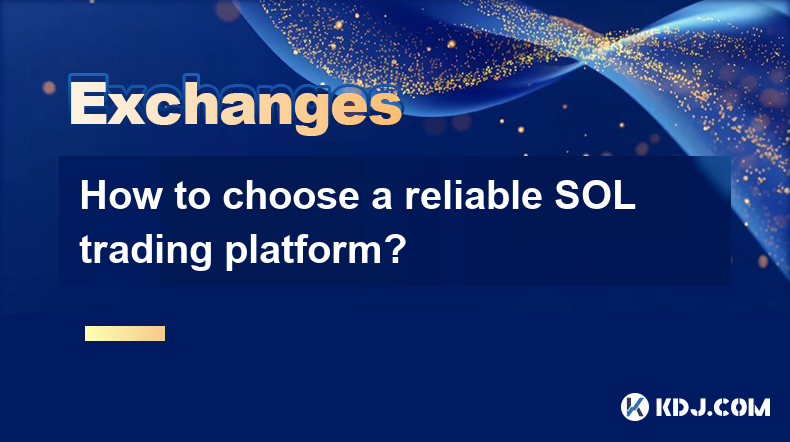
How to choose a reliable SOL trading platform?
Apr 21,2025 at 12:07am
Choosing a reliable SOL trading platform is crucial for anyone looking to engage in trading Solana (SOL) cryptocurrency. With the growing popularity of Solana, numerous platforms have emerged, each offering different features and levels of security. This article will guide you through the essential factors to consider when selecting a reliable SOL tradi...
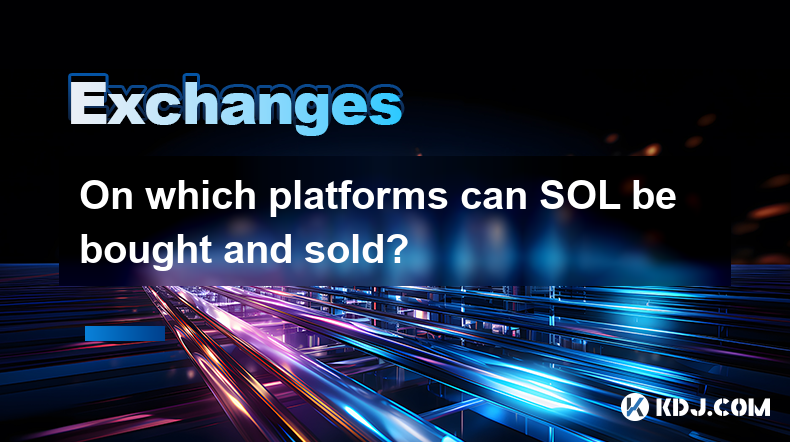
On which platforms can SOL be bought and sold?
Apr 21,2025 at 10:22am
Solana (SOL) is a popular cryptocurrency known for its high transaction speeds and low fees, making it a favored choice among crypto enthusiasts. If you're looking to buy or sell SOL, there are several platforms where you can do so. In this article, we will explore the various platforms that support the trading of SOL, ensuring you have a comprehensive ...
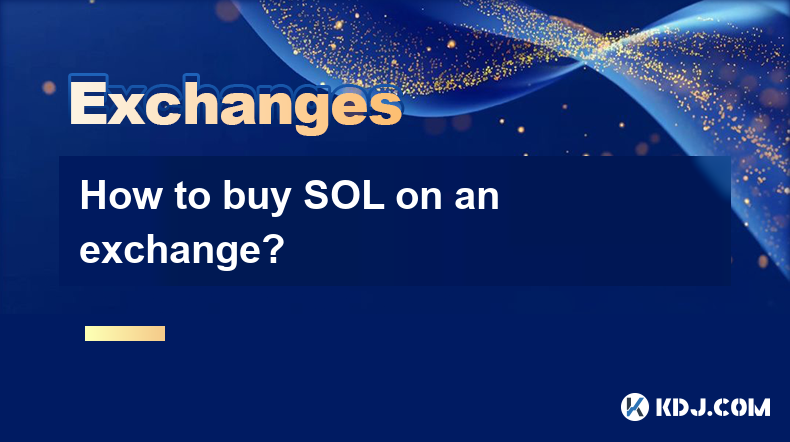
How to buy SOL on an exchange?
Apr 20,2025 at 01:21am
Introduction to Buying SOL on an ExchangeSOL, the native cryptocurrency of the Solana blockchain, has garnered significant attention in the crypto world due to its high throughput and low transaction costs. If you're interested in adding SOL to your investment portfolio, buying it on a cryptocurrency exchange is one of the most straightforward methods. ...
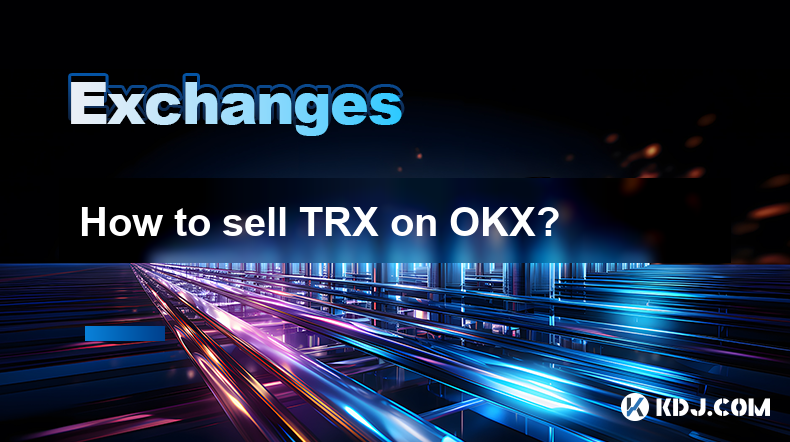
How to sell TRX on OKX?
Apr 18,2025 at 11:07pm
Selling TRX on OKX is a straightforward process that can be completed in a few simple steps. This article will guide you through the entire process, ensuring that you understand each step thoroughly. Whether you are a beginner or an experienced trader, this guide will help you navigate the OKX platform with ease. Preparing to Sell TRX on OKXBefore you c...
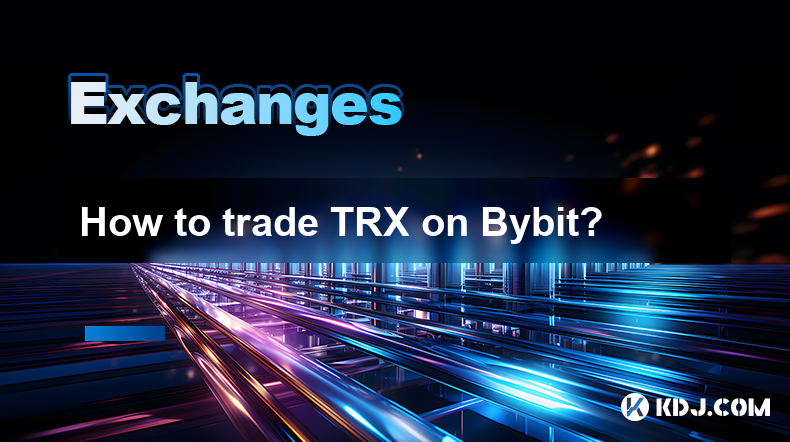
How to trade TRX on Bybit?
Apr 20,2025 at 04:15pm
Trading TRX on Bybit can be an exciting venture for both new and experienced cryptocurrency traders. Bybit, known for its robust trading platform and user-friendly interface, offers a variety of features that can help you trade TRX effectively. In this guide, we'll walk you through the essential steps and tips to successfully trade TRX on Bybit. Setting...
See all articles
