-
Bitcoin
$83,877.1483
-2.32% -
Ethereum
$1,580.2280
-3.79% -
Tether USDt
$0.9999
0.01% -
XRP
$2.0721
-3.70% -
BNB
$580.5381
-1.38% -
Solana
$125.3784
-4.85% -
USDC
$1.0000
0.01% -
TRON
$0.2534
0.77% -
Dogecoin
$0.1539
-3.73% -
Cardano
$0.6098
-5.48% -
UNUS SED LEO
$9.3966
-0.35% -
Chainlink
$12.2635
-3.41% -
Avalanche
$18.9039
-5.31% -
Stellar
$0.2347
-2.67% -
Toncoin
$2.8692
-3.67% -
Shiba Inu
$0.0...01165
-2.69% -
Sui
$2.0967
-4.89% -
Hedera
$0.1580
-5.07% -
Bitcoin Cash
$322.5592
-3.37% -
Litecoin
$76.0764
-2.46% -
Polkadot
$3.5464
-4.03% -
Dai
$1.0002
0.02% -
Bitget Token
$4.2676
-1.86% -
Hyperliquid
$15.0668
-8.19% -
Ethena USDe
$0.9992
0.01% -
Pi
$0.6164
-16.96% -
Monero
$219.0365
3.06% -
Uniswap
$5.1848
-3.95% -
OKB
$52.3728
0.37% -
Pepe
$0.0...07093
-4.62%
How to use Bitfinex's REST API?
Bitfinex's REST API lets you trade, get market data, and manage your account programmatically; this guide provides steps and examples for effective use.
Apr 14, 2025 at 03:35 am
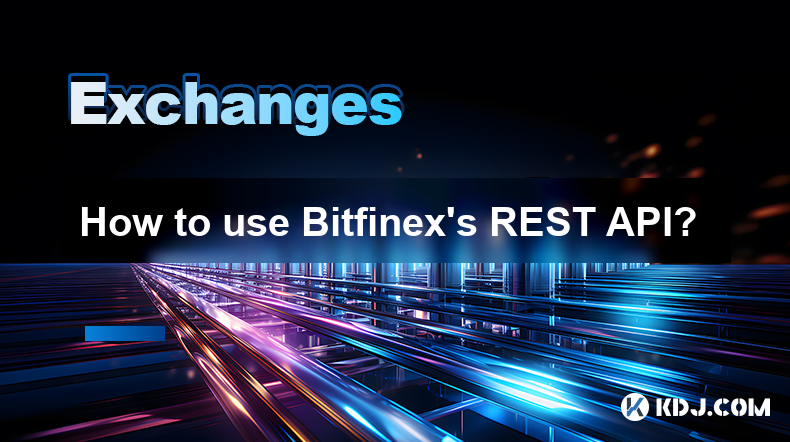
Using Bitfinex's REST API allows you to interact programmatically with the exchange, enabling you to perform tasks such as trading, retrieving market data, and managing your account. This guide will walk you through the essential steps and provide detailed instructions on how to use Bitfinex's REST API effectively.
Getting Started with Bitfinex's REST API
Before you can start using Bitfinex's REST API, you need to set up an API key. To do this, follow these steps:
- Log in to your Bitfinex account.
- Navigate to the 'Account' section and select 'API'.
- Click on 'New Key' to generate a new API key.
- Choose the permissions you want to grant to the key, such as trading, withdrawals, or read-only access.
- Confirm the creation of the key and securely store the API key and secret.
Once you have your API key and secret, you can start making requests to the Bitfinex REST API. The API uses HTTPS and supports JSON for data exchange.
Authentication and Security
Authentication is crucial for securing your API interactions. Bitfinex uses a combination of your API key and a signature generated using your API secret. Here's how to authenticate your requests:
- Generate a nonce: A unique number that ensures each request is unique. This can be a timestamp or an incrementing integer.
- Create a payload: Combine the API path, nonce, and any additional parameters into a JSON object.
- Generate the signature: Use the HMAC-SHA384 algorithm with your API secret to sign the payload.
- Include the headers: Add the API key, payload, and signature to your request headers.
Here is an example of how to create the necessary headers in Python:
import time
import json
import hmac
import hashlibapi_key = 'your_api_key'
api_secret = 'your_api_secret'.encode()
Generate nonce
nonce = str(int(time.time() * 1000))
Create payload
payload = {
'request': '/v1/balance',
'nonce': nonce
}
Convert payload to JSON and encode
payload_json = json.dumps(payload).encode()
Generate signature
signature = hmac.new(api_secret, payload_json, hashlib.sha384).hexdigest()
Prepare headers
headers = {
'X-BFX-APIKEY': api_key,
'X-BFX-PAYLOAD': payload_json.decode(),
'X-BFX-SIGNATURE': signature
}
Making API Requests
With authentication in place, you can now make requests to Bitfinex's REST API. Here are some common endpoints and how to use them:
- Retrieve Account Balances: Use the
/v1/balances
endpoint to check your account balances.
import requestsurl = 'https://api.bitfinex.com/v1/balances'
response = requests.get(url, headers=headers)
print(response.json())
- Place an Order: Use the
/v1/order/new
endpoint to place a new order. You need to specify the symbol, amount, price, and order type.
payload = {'request': '/v1/order/new',
'nonce': nonce,
'symbol': 'btcusd',
'amount': '0.01',
'price': '10000',
'exchange': 'bitfinex',
'type': 'exchange limit',
'side': 'buy'
}
payload_json = json.dumps(payload).encode()
signature = hmac.new(api_secret, payload_json, hashlib.sha384).hexdigest()
headers = {
'X-BFX-APIKEY': api_key,
'X-BFX-PAYLOAD': payload_json.decode(),
'X-BFX-SIGNATURE': signature
}
url = 'https://api.bitfinex.com/v1/order/new'
response = requests.post(url, headers=headers)
print(response.json())
- Retrieve Market Data: Use the
/v1/pubticker
endpoint to get the current ticker for a specific trading pair.
url = 'https://api.bitfinex.com/v1/pubticker/btcusd'
response = requests.get(url)
print(response.json())
Handling Errors and Responses
When using the Bitfinex REST API, it's important to handle errors and interpret responses correctly. Here are some tips:
- Check the HTTP status code: A 200 status code indicates a successful request, while other codes indicate errors.
- Parse the response JSON: The response will contain a JSON object with the requested data or error messages.
- Common error codes: Familiarize yourself with common error codes like 400 (Bad Request), 401 (Unauthorized), and 500 (Internal Server Error).
Here's an example of how to handle errors in Python:
import requestsurl = 'https://api.bitfinex.com/v1/balances'
response = requests.get(url, headers=headers)
if response.status_code == 200:
print('Request successful:', response.json())
else:
print('Error:', response.status_code, response.text)
Using WebSockets for Real-Time Data
While the REST API is suitable for many tasks, using WebSockets can provide real-time data updates. To connect to Bitfinex's WebSocket API, follow these steps:
- Establish a WebSocket connection: Use a WebSocket library like
websocket-client
in Python.
import websocket
ws = websocket.WebSocket()
ws.connect('wss://api-pub.bitfinex.com/ws/2')
- Subscribe to channels: Send a JSON message to subscribe to specific channels, such as ticker or order book updates.
subscribe_msg = {'event': 'subscribe',
'channel': 'ticker',
'symbol': 'tBTCUSD'
}
ws.send(json.dumps(subscribe_msg))
- Process incoming messages: Parse the incoming JSON messages to handle real-time data.
result = ws.recv()
print(result)
Managing API Rate Limits
Bitfinex imposes rate limits on API requests to prevent abuse. To manage these limits effectively:
- Understand the limits: Bitfinex has different rate limits for authenticated and unauthenticated requests. Authenticated requests are typically limited to 90 requests per minute.
- Implement rate limiting in your code: Use libraries like
ratelimit
in Python to ensure you stay within the limits.
from ratelimit import limits, sleep_and_retry@sleep_and_retry
@limits(calls=90, period=60)
def call_api():
# Make your API call here
pass
Use the function
call_api()
Frequently Asked Questions
Q: Can I use Bitfinex's REST API to automate trading strategies?
A: Yes, you can use the REST API to automate trading strategies by programmatically placing orders, checking balances, and retrieving market data. Ensure you implement proper error handling and rate limiting to maintain a stable trading environment.
Q: Is it possible to withdraw funds using the Bitfinex REST API?
A: Yes, you can withdraw funds using the /v1/withdraw
endpoint. You need to specify the withdrawal method, amount, and address. Make sure you have the necessary permissions on your API key to perform withdrawals.
Q: How can I test my API requests without affecting my live account?
A: Bitfinex offers a testnet environment where you can test your API requests without affecting your live account. You can sign up for a testnet account and use the testnet API endpoints to simulate trading and other operations.
Q: What should I do if I encounter an authentication error?
A: If you encounter an authentication error, double-check your API key, secret, and the signature generation process. Ensure the nonce is unique for each request and that the payload is correctly formatted. If issues persist, consider regenerating your API key and secret.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- MEDIA took a nosedive today, dropping over 60% after Coinbase officially delisted the token
- 2025-04-16 16:25:14
- Movement Labs Investigates Market Maker Issues Following Binance Delisting of MOVE Token Partner
- 2025-04-16 16:25:14
- List Of 5 No KYC Online Casinos Of 2025 - Latest Bonuses!
- 2025-04-16 16:20:15
- Trump Family Has Poured Over $1 Billion into Cryptocurrency Projects
- 2025-04-16 16:20:15
- Could Bitcoin Be Your Safe Harbor Amid Global Market Uncertainty?
- 2025-04-16 16:20:13
- Wolf Game 2.0 on Solana Promises New Mechanics, But Backlash From Community Results in Cancelled Relaunch
- 2025-04-16 16:20:13
Related knowledge

Does Bithumb have 24-hour customer service support?
Apr 16,2025 at 05:14pm
Does Bithumb Have 24-Hour Customer Service Support?When engaging with cryptocurrency exchanges, one critical aspect users often consider is the availability and responsiveness of customer service. Bithumb, one of the leading cryptocurrency exchanges in South Korea, has a significant user base that relies on its services. A common question among potentia...
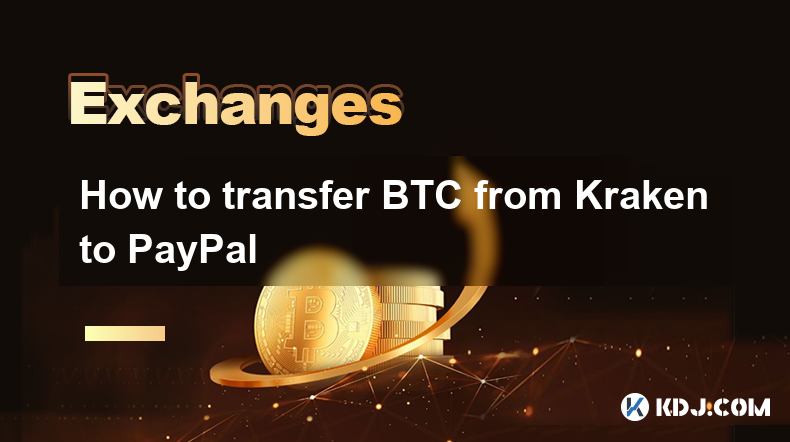
How to transfer BTC from Kraken to PayPal
Apr 16,2025 at 02:28pm
Transferring Bitcoin (BTC) from Kraken to PayPal involves a series of steps that require careful attention to detail. While Kraken does not directly support transfers to PayPal, you can achieve this by using a third-party service that converts your cryptocurrency into fiat currency, which can then be sent to your PayPal account. In this article, we will...
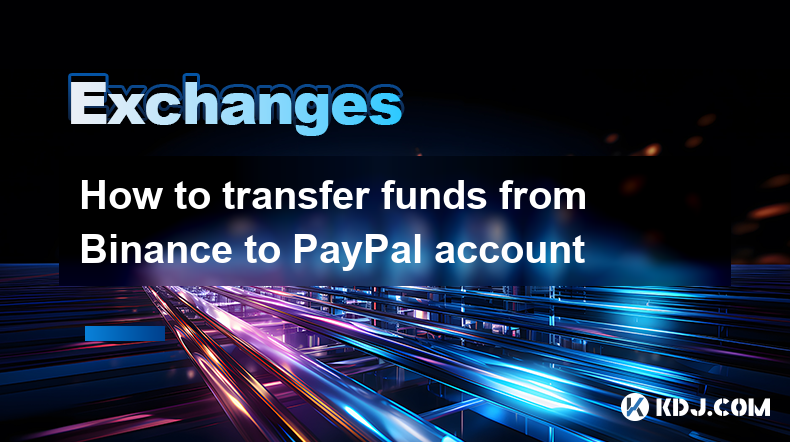
How to transfer funds from Binance to PayPal account
Apr 16,2025 at 02:50pm
Transferring funds from Binance to a PayPal account involves several steps and considerations due to the nature of cryptocurrency transactions and the policies of both platforms. This process is not direct, as Binance does not offer a straightforward option to send funds directly to PayPal. Instead, you will need to convert your cryptocurrency to a fiat...
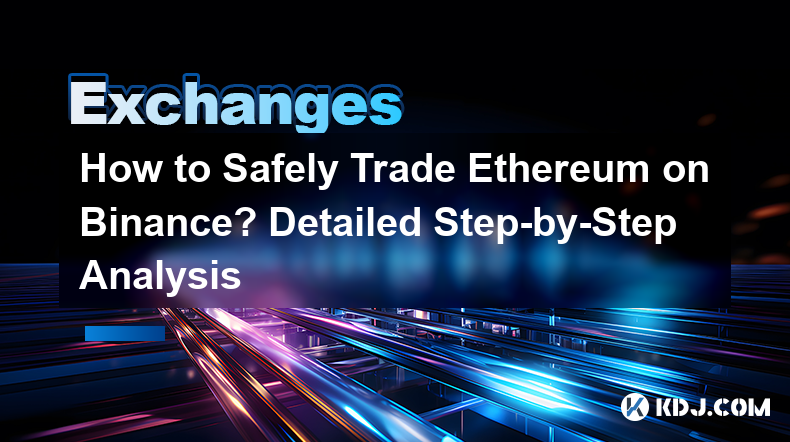
How to Safely Trade Ethereum on Binance? Detailed Step-by-Step Analysis
Apr 16,2025 at 04:57pm
Trading Ethereum on Binance can be a lucrative venture, but it requires careful planning and execution to ensure safety and profitability. This article provides a detailed step-by-step analysis on how to safely trade Ethereum on Binance, covering everything from setting up your account to executing trades and managing your assets securely. Setting Up Yo...
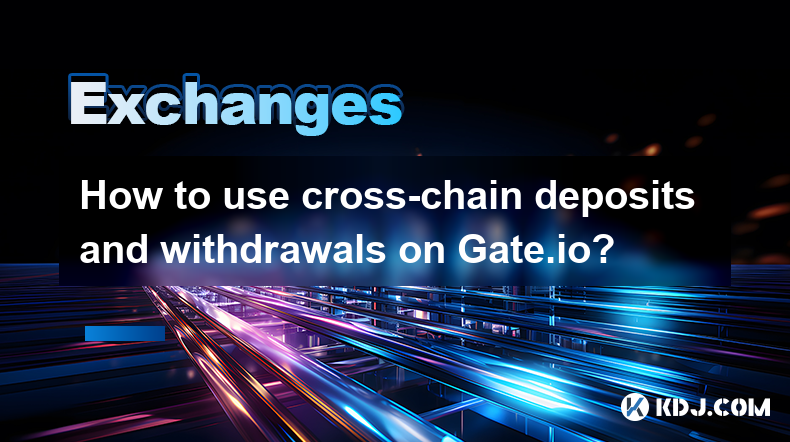
How to use cross-chain deposits and withdrawals on Gate.io?
Apr 16,2025 at 03:08pm
Using cross-chain deposits and withdrawals on Gate.io can significantly enhance your cryptocurrency management by allowing you to transfer assets across different blockchain networks efficiently. This guide will walk you through the process step-by-step, ensuring you understand every aspect of the operation. Understanding Cross-Chain TransactionsCross-c...
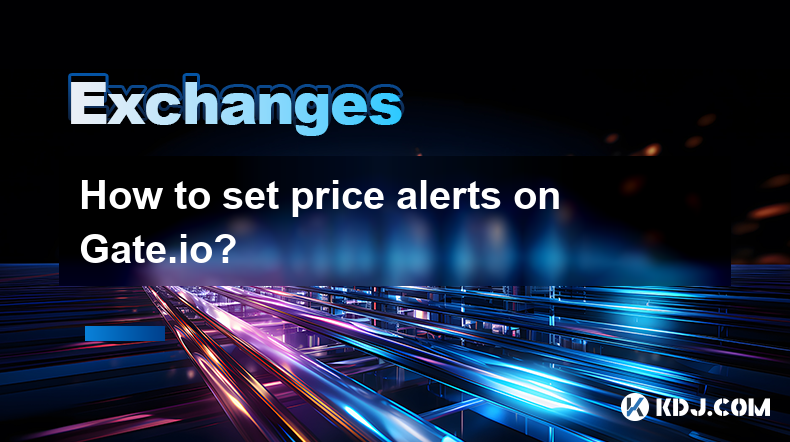
How to set price alerts on Gate.io?
Apr 16,2025 at 02:14pm
Setting price alerts on Gate.io can be a vital tool for traders looking to stay informed about market movements without constantly monitoring their screens. Whether you're interested in a specific cryptocurrency or multiple assets, setting up price alerts can help you make timely decisions. This guide will walk you through the detailed steps required to...

Does Bithumb have 24-hour customer service support?
Apr 16,2025 at 05:14pm
Does Bithumb Have 24-Hour Customer Service Support?When engaging with cryptocurrency exchanges, one critical aspect users often consider is the availability and responsiveness of customer service. Bithumb, one of the leading cryptocurrency exchanges in South Korea, has a significant user base that relies on its services. A common question among potentia...
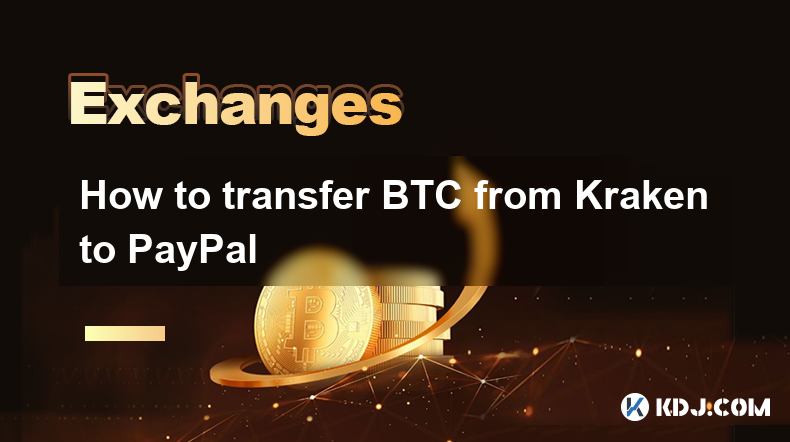
How to transfer BTC from Kraken to PayPal
Apr 16,2025 at 02:28pm
Transferring Bitcoin (BTC) from Kraken to PayPal involves a series of steps that require careful attention to detail. While Kraken does not directly support transfers to PayPal, you can achieve this by using a third-party service that converts your cryptocurrency into fiat currency, which can then be sent to your PayPal account. In this article, we will...
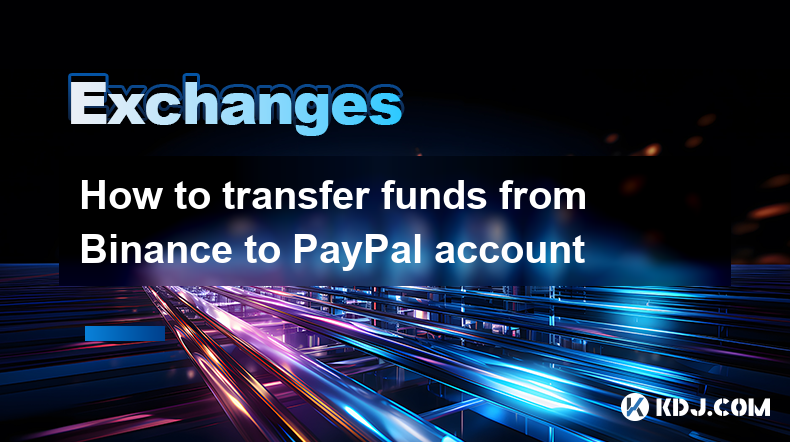
How to transfer funds from Binance to PayPal account
Apr 16,2025 at 02:50pm
Transferring funds from Binance to a PayPal account involves several steps and considerations due to the nature of cryptocurrency transactions and the policies of both platforms. This process is not direct, as Binance does not offer a straightforward option to send funds directly to PayPal. Instead, you will need to convert your cryptocurrency to a fiat...
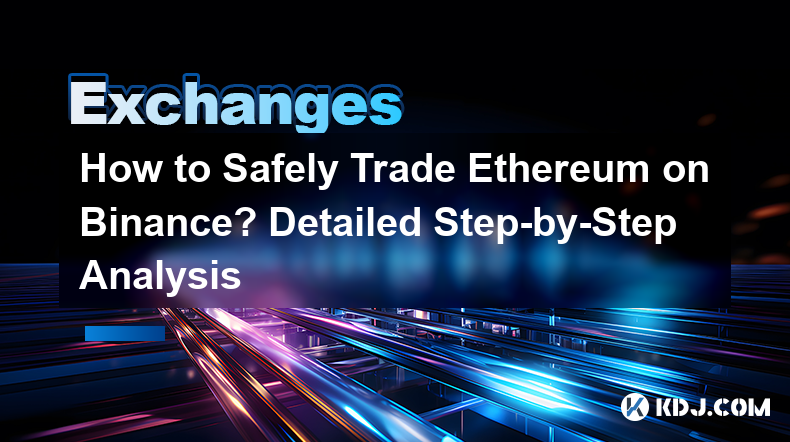
How to Safely Trade Ethereum on Binance? Detailed Step-by-Step Analysis
Apr 16,2025 at 04:57pm
Trading Ethereum on Binance can be a lucrative venture, but it requires careful planning and execution to ensure safety and profitability. This article provides a detailed step-by-step analysis on how to safely trade Ethereum on Binance, covering everything from setting up your account to executing trades and managing your assets securely. Setting Up Yo...
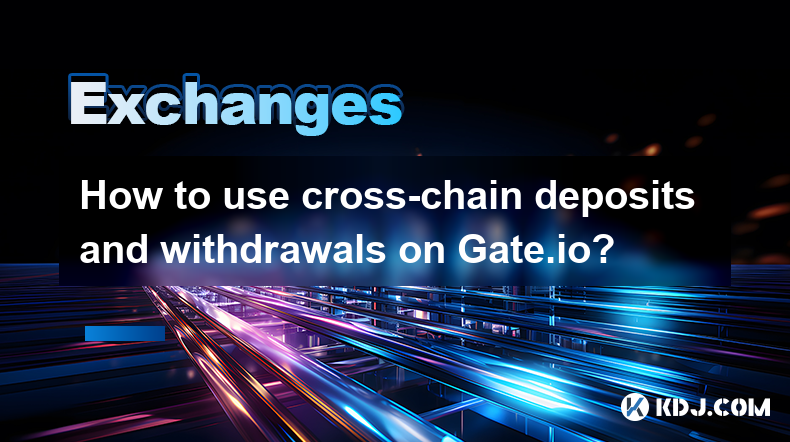
How to use cross-chain deposits and withdrawals on Gate.io?
Apr 16,2025 at 03:08pm
Using cross-chain deposits and withdrawals on Gate.io can significantly enhance your cryptocurrency management by allowing you to transfer assets across different blockchain networks efficiently. This guide will walk you through the process step-by-step, ensuring you understand every aspect of the operation. Understanding Cross-Chain TransactionsCross-c...
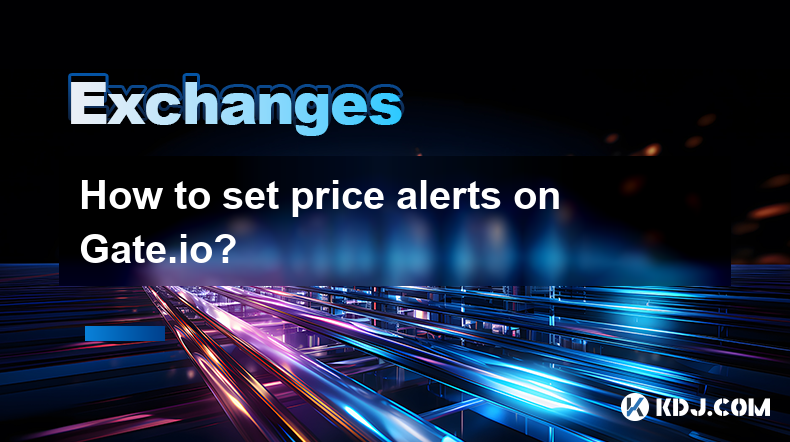
How to set price alerts on Gate.io?
Apr 16,2025 at 02:14pm
Setting price alerts on Gate.io can be a vital tool for traders looking to stay informed about market movements without constantly monitoring their screens. Whether you're interested in a specific cryptocurrency or multiple assets, setting up price alerts can help you make timely decisions. This guide will walk you through the detailed steps required to...
See all articles
