-
Bitcoin
$84,094.4129
1.60% -
Ethereum
$1,821.7742
0.59% -
Tether USDt
$0.9997
0.00% -
XRP
$2.1407
3.87% -
BNB
$598.6984
1.20% -
Solana
$123.4827
5.75% -
USDC
$1.0000
0.01% -
Dogecoin
$0.1722
7.24% -
Cardano
$0.6642
2.33% -
TRON
$0.2385
0.43% -
UNUS SED LEO
$9.5334
1.35% -
Chainlink
$13.0140
1.67% -
Toncoin
$3.4052
-5.95% -
Stellar
$0.2604
-0.03% -
Avalanche
$18.2768
0.91% -
Sui
$2.2810
1.88% -
Shiba Inu
$0.0...01232
0.77% -
Hedera
$0.1646
1.14% -
Litecoin
$84.6257
1.99% -
Polkadot
$4.0498
-0.10% -
MANTRA
$6.2711
-2.10% -
Bitcoin Cash
$302.6871
0.34% -
Bitget Token
$4.5346
1.16% -
Dai
$1.0000
0.00% -
Ethena USDe
$0.9991
-0.04% -
Hyperliquid
$12.2705
5.21% -
Monero
$217.6766
2.41% -
Uniswap
$5.9514
2.02% -
Pi
$0.5240
-7.74% -
Pepe
$0.0...07231
8.85%
How to use MetaMask wallet API?
MetaMask Wallet API enables seamless integration of Ethereum wallet functionalities into apps, allowing for user authentication and transaction handling.
Apr 03, 2025 at 03:29 pm
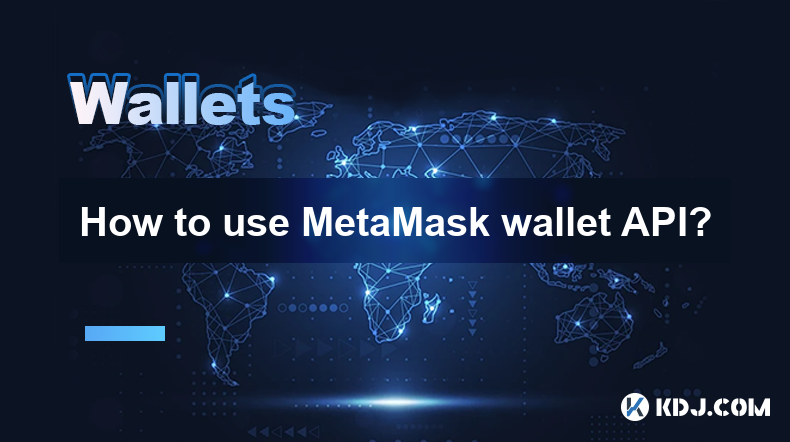
How to Use MetaMask Wallet API
MetaMask is a popular Ethereum wallet that allows users to interact with decentralized applications (dApps) directly from their browser. The MetaMask Wallet API provides developers with the tools to integrate MetaMask into their applications, enabling seamless user authentication and transaction handling. In this article, we will explore how to use the MetaMask Wallet API, covering its setup, key functionalities, and common use cases.
Setting Up MetaMask
Before diving into the API, ensure you have MetaMask installed and set up in your browser. Here's how to get started:
- Visit the MetaMask website and download the extension for your preferred browser.
- Install the extension and follow the prompts to create a new wallet or import an existing one.
- Once set up, you can access your wallet from the browser toolbar.
Connecting to MetaMask
To connect your application to MetaMask, you need to use the Ethereum provider injected by MetaMask into the browser's window object. Here's how you can detect and connect to MetaMask:
- First, check if MetaMask is available by detecting the
window.ethereum
object. - If available, you can request access to the user's accounts using
ethereum.request({ method: 'eth_requestAccounts' })
. - Once connected, you can interact with the Ethereum blockchain through the
ethereum
object.
if (typeof window.ethereum !== 'undefined') {
console.log('MetaMask is installed!');
window.ethereum.request({ method: 'eth_requestAccounts' }).then(accounts => {
console.log('Connected account:', accounts[0]);
})
.catch(error => {
console.error('Error connecting:', error);
});
} else {
console.log('MetaMask is not installed!');
}
Sending Transactions
One of the primary uses of the MetaMask Wallet API is to send transactions. Here’s how you can send a transaction using MetaMask:
- Ensure the user is connected to MetaMask.
- Use the
eth_sendTransaction
method to send a transaction. - MetaMask will prompt the user to confirm the transaction details before sending.
window.ethereum.request({
method: 'eth_sendTransaction',
params: [{from: '0xb60e8dd61c5d32be8058bb8eb970870f07233155',
to: '0xd46e8dd67c5d32be8058bb8eb970870f07233155',
value: '0x9184e72a000', // 10000000000000 wei (0.00001 ETH)
gasPrice: '0x09184e72a000', // 1000000000 wei
gas: '0x5208', // 21000 gas
}],
}).then(txHash => {
console.log('Transaction hash:', txHash);
}).catch(error => {
console.error('Error sending transaction:', error);
});
Signing Messages
Another common use case is signing messages, which can be used for authentication or other purposes. Here’s how you can sign a message using MetaMask:
- Use the
personal_sign
method to sign a message. - MetaMask will prompt the user to confirm the signing request.
const message = 'Hello, MetaMask!';
window.ethereum.request({
method: 'personal_sign',
params: [message, '0xb60e8dd61c5d32be8058bb8eb970870f07233155'],
}).then(signature => {
console.log('Signature:', signature);
}).catch(error => {
console.error('Error signing message:', error);
});
Handling Events
MetaMask provides several events that you can listen to in order to respond to changes in the user's wallet or network. Here are some key events to handle:
- Accounts Changed: This event is triggered when the user switches accounts in MetaMask.
- Network Changed: This event is triggered when the user switches networks in MetaMask.
- Chain Changed: This event is triggered when the user switches chains in MetaMask.
window.ethereum.on('accountsChanged', function (accounts) {
console.log('Accounts changed:', accounts);
});window.ethereum.on('networkChanged', function (networkId) {
console.log('Network changed:', networkId);
});
window.ethereum.on('chainChanged', function (chainId) {
console.log('Chain changed:', chainId);
});
Using MetaMask with Web3.js
Integrating MetaMask with Web3.js can enhance your application's capabilities. Here’s how you can set up Web3.js to work with MetaMask:
- Install Web3.js using npm or yarn.
- Initialize a new Web3 instance using the
window.ethereum
provider.
const Web3 = require('web3');
const web3 = new Web3(window.ethereum);
Once set up, you can use Web3.js methods to interact with the Ethereum blockchain, such as fetching account balances, sending transactions, and interacting with smart contracts.
web3.eth.getAccounts().then(accounts => {
console.log('Accounts:', accounts);
});web3.eth.getBalance('0xb60e8dd61c5d32be8058bb8eb970870f07233155').then(balance => {
console.log('Balance:', web3.utils.fromWei(balance, 'ether'), 'ETH');
});
Advanced Use Cases
For more advanced use cases, you might want to explore additional functionalities provided by the MetaMask Wallet API, such as:
- Customizing Transaction Requests: You can customize transaction requests by specifying gas limits, gas prices, and other parameters.
- Interacting with Smart Contracts: Use the
eth_call
method to interact with smart contracts without sending a transaction. - Batch Requests: Send multiple requests to the Ethereum blockchain in a single call using the
eth_batchRequest
method.
const contractAddress = '0x123456789abcdef';
const contractABI = [...]; // ABI of the smart contract
const contract = new web3.eth.Contract(contractABI, contractAddress);contract.methods.someMethod().call()
.then(result => {
console.log('Result:', result);
})
.catch(error => {
console.error('Error calling method:', error);
});
Security Considerations
When using the MetaMask Wallet API, it's crucial to consider security implications. Here are some best practices:
- Never Store Private Keys: MetaMask manages private keys securely on the user's device. Never ask users to share their private keys.
- Use HTTPS: Ensure your application uses HTTPS to prevent man-in-the-middle attacks.
- Validate User Input: Always validate and sanitize user input to prevent malicious data from being sent to the blockchain.
- Error Handling: Implement robust error handling to gracefully manage failed transactions or API calls.
Common Errors and Troubleshooting
When working with the MetaMask Wallet API, you might encounter various errors. Here are some common issues and how to troubleshoot them:
- User Rejected Request: This error occurs when the user denies a transaction or signing request. Ensure your application handles this gracefully and provides clear instructions to the user.
- Network Request Failed: This can happen if the user is not connected to the correct network. Prompt the user to switch to the required network.
- Insufficient Funds: If a transaction fails due to insufficient funds, inform the user and suggest they add more funds to their wallet.
FAQs
Q: How do I install MetaMask?
A: Visit the MetaMask website, download the extension for your preferred browser, and follow the prompts to create a new wallet or import an existing one.
Q: How can I detect if MetaMask is installed in the browser?
A: You can detect MetaMask by checking for the window.ethereum
object. If it exists, MetaMask is installed.
Q: What is the eth_requestAccounts
method used for?
A: The eth_requestAccounts
method is used to request access to the user's Ethereum accounts. It prompts the user to connect their MetaMask wallet to your application.
Q: How do I send a transaction using MetaMask?
A: Use the eth_sendTransaction
method to send a transaction. MetaMask will prompt the user to confirm the transaction details before sending.
Q: Can I sign messages with MetaMask?
A: Yes, you can sign messages using the personal_sign
method. MetaMask will prompt the user to confirm the signing request.
Q: What events should I listen to when using MetaMask?
A: Key events to listen to include accountsChanged
, networkChanged
, and chainChanged
. These events help you respond to changes in the user's wallet or network.
Q: How can I integrate MetaMask with Web3.js?
A: Install Web3.js and initialize a new Web3 instance using the window.ethereum
provider. You can then use Web3.js methods to interact with the Ethereum blockchain.
Q: What are some security best practices when using the MetaMask Wallet API?
A: Never store private keys, use HTTPS, validate user input, and implement robust error handling to ensure the security of your application.
Q: What should I do if a user rejects a transaction request?
A: Handle the "User Rejected Request" error gracefully and provide clear instructions to the user on how to proceed.
Q: How can I troubleshoot network request failures with MetaMask?
A: Prompt the user to switch to the required network if a network request fails due to being on the wrong network.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- As Excitement Continues to Swell Around XRP's Bullish Potential
- 2025-04-05 06:30:12
- Bitcoin (BTC) Drops to $82,000 Following Donald Trump's Widespread Tariffs
- 2025-04-05 06:30:12
- Bitcoin (BTC) is trading at $84,003, facing potential bearish pressure across multiple timeframes
- 2025-04-05 06:25:12
- Binance (BNB) Confirms That FDUSD's Reserves Exceed Its Circulation
- 2025-04-05 06:25:12
- Payment giant PayPal adds Solana (SOL) and Chainlink (LINK) to its growing list of supported digital assets
- 2025-04-05 06:20:12
- Binance Declines to List Pi Network in Its Newest Vote to List Initiative
- 2025-04-05 06:20:12
Related knowledge
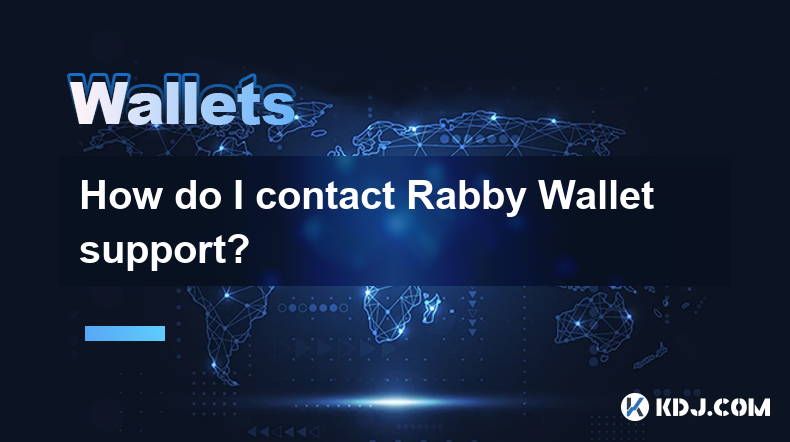
How do I contact Rabby Wallet support?
Apr 04,2025 at 08:42am
Introduction to Rabby Wallet SupportIf you are a user of Rabby Wallet and need assistance, knowing how to contact their support team is crucial. Rabby Wallet offers various methods to reach out for help, ensuring that users can get the support they need efficiently. This article will guide you through the different ways to contact Rabby Wallet support, ...
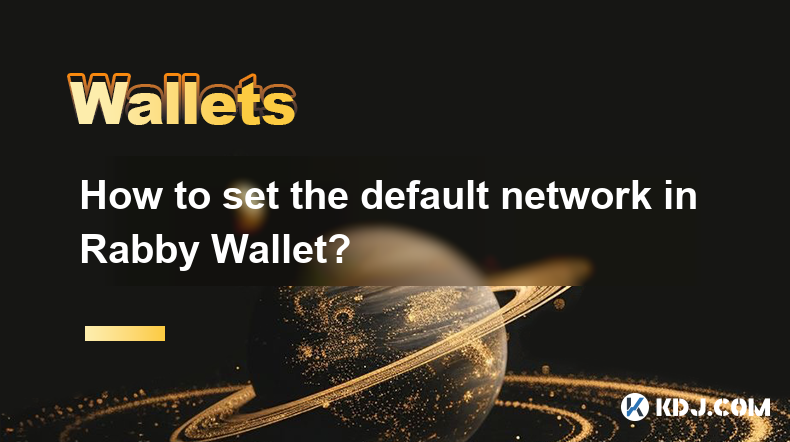
How to set the default network in Rabby Wallet?
Apr 04,2025 at 06:35am
Setting the default network in Rabby Wallet is a crucial step for users who frequently interact with different blockchain networks. This guide will walk you through the process of setting your preferred network as the default, ensuring a seamless experience when managing your cryptocurrencies. Whether you're using Ethereum, Binance Smart Chain, or any o...
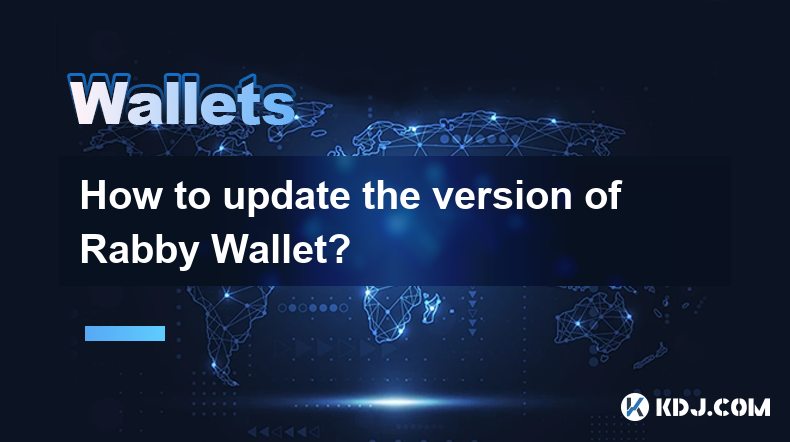
How to update the version of Rabby Wallet?
Apr 05,2025 at 02:14am
Updating the version of Rabby Wallet is an essential task to ensure you have the latest features, security enhancements, and bug fixes. This guide will walk you through the process of updating Rabby Wallet on different platforms, including desktop and mobile devices. Let's dive into the detailed steps for each platform. Updating Rabby Wallet on DesktopU...
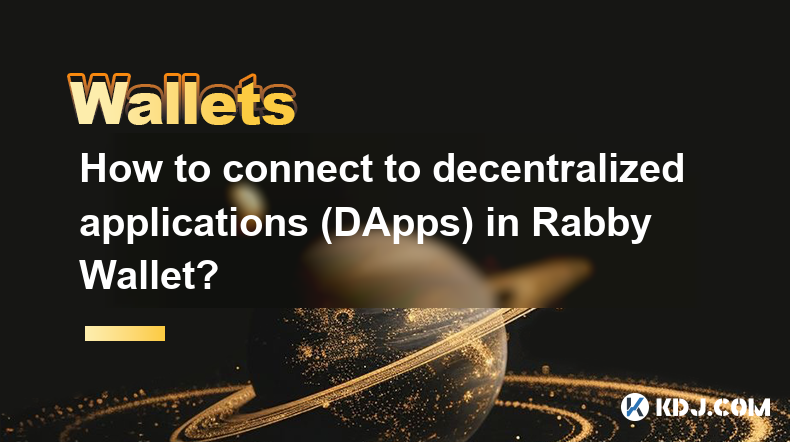
How to connect to decentralized applications (DApps) in Rabby Wallet?
Apr 05,2025 at 01:28am
Connecting to decentralized applications (DApps) using Rabby Wallet is a straightforward process that enhances your interaction with the burgeoning world of blockchain technology. Rabby Wallet, known for its user-friendly interface and robust security features, allows users to seamlessly interact with a variety of DApps across different blockchains. Thi...
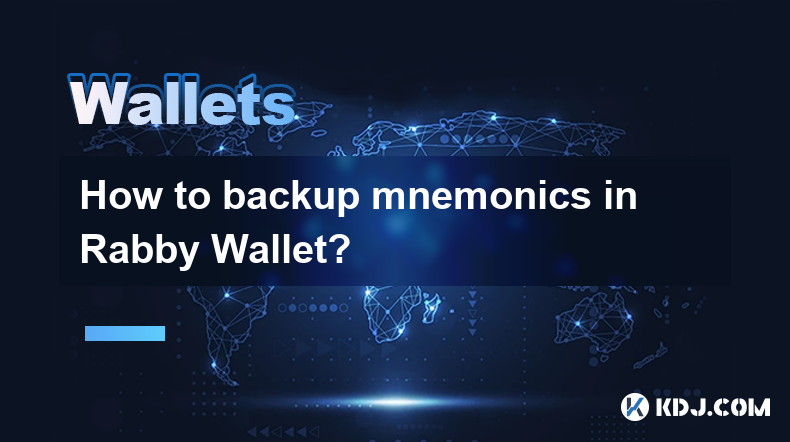
How to backup mnemonics in Rabby Wallet?
Apr 04,2025 at 02:21pm
Introduction to Rabby Wallet and MnemonicsRabby Wallet is a popular cryptocurrency wallet that offers users a secure way to manage their digital assets. One of the key features of Rabby Wallet is the use of mnemonics, which are a series of words that serve as a backup for your wallet. These mnemonics are crucial because they allow you to recover your wa...
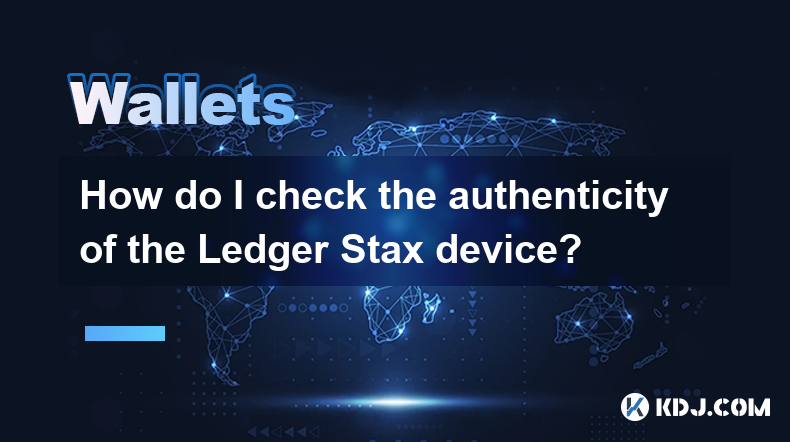
How do I check the authenticity of the Ledger Stax device?
Apr 04,2025 at 11:07am
Understanding the Importance of AuthenticityWhen investing in a hardware wallet like the Ledger Stax, ensuring its authenticity is crucial. A genuine device guarantees the security of your cryptocurrencies, protecting them from potential hacks and unauthorized access. Counterfeit devices can compromise your private keys, leading to significant financial...
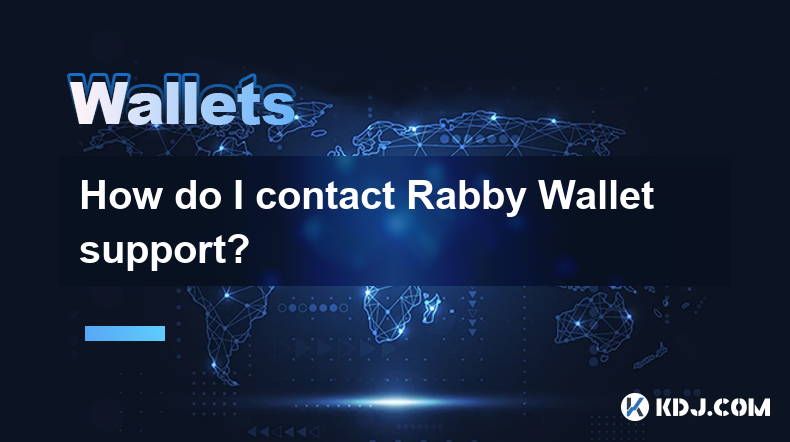
How do I contact Rabby Wallet support?
Apr 04,2025 at 08:42am
Introduction to Rabby Wallet SupportIf you are a user of Rabby Wallet and need assistance, knowing how to contact their support team is crucial. Rabby Wallet offers various methods to reach out for help, ensuring that users can get the support they need efficiently. This article will guide you through the different ways to contact Rabby Wallet support, ...
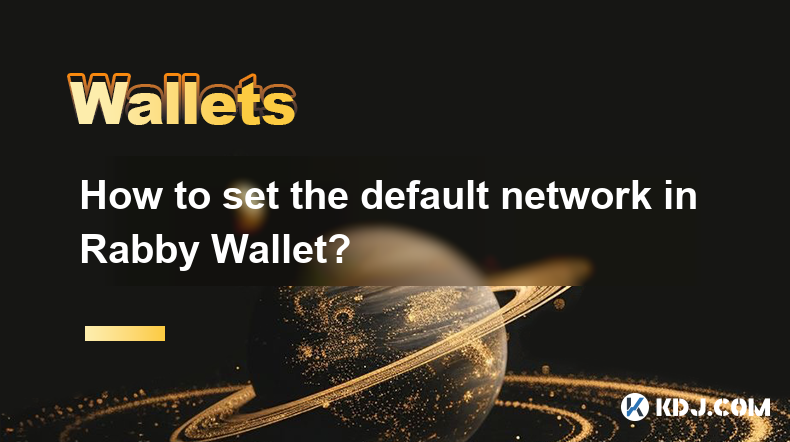
How to set the default network in Rabby Wallet?
Apr 04,2025 at 06:35am
Setting the default network in Rabby Wallet is a crucial step for users who frequently interact with different blockchain networks. This guide will walk you through the process of setting your preferred network as the default, ensuring a seamless experience when managing your cryptocurrencies. Whether you're using Ethereum, Binance Smart Chain, or any o...
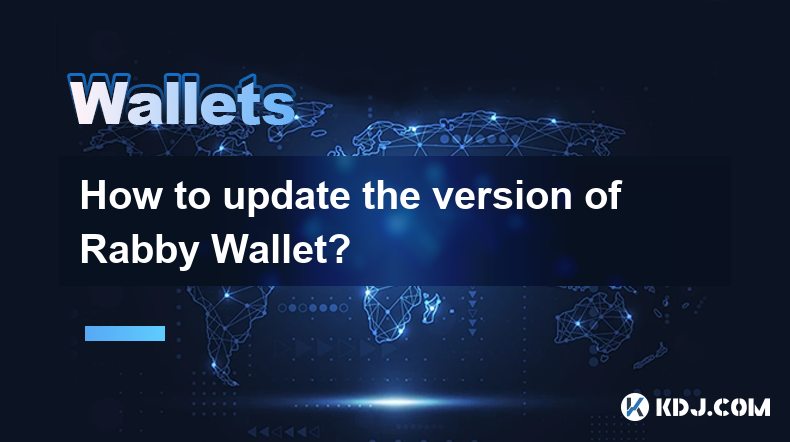
How to update the version of Rabby Wallet?
Apr 05,2025 at 02:14am
Updating the version of Rabby Wallet is an essential task to ensure you have the latest features, security enhancements, and bug fixes. This guide will walk you through the process of updating Rabby Wallet on different platforms, including desktop and mobile devices. Let's dive into the detailed steps for each platform. Updating Rabby Wallet on DesktopU...
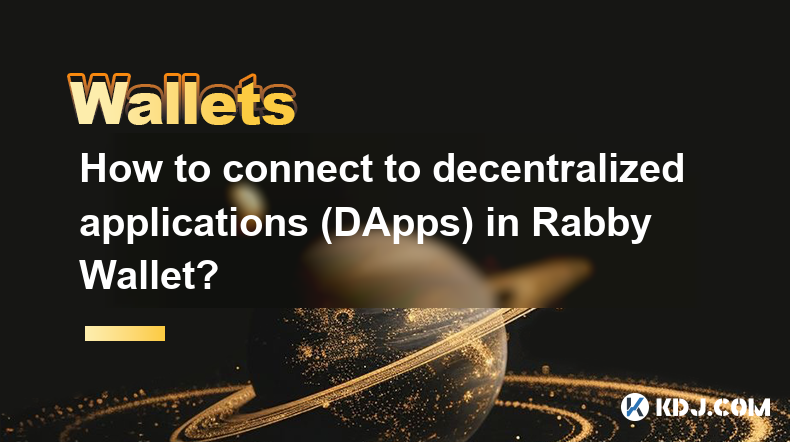
How to connect to decentralized applications (DApps) in Rabby Wallet?
Apr 05,2025 at 01:28am
Connecting to decentralized applications (DApps) using Rabby Wallet is a straightforward process that enhances your interaction with the burgeoning world of blockchain technology. Rabby Wallet, known for its user-friendly interface and robust security features, allows users to seamlessly interact with a variety of DApps across different blockchains. Thi...
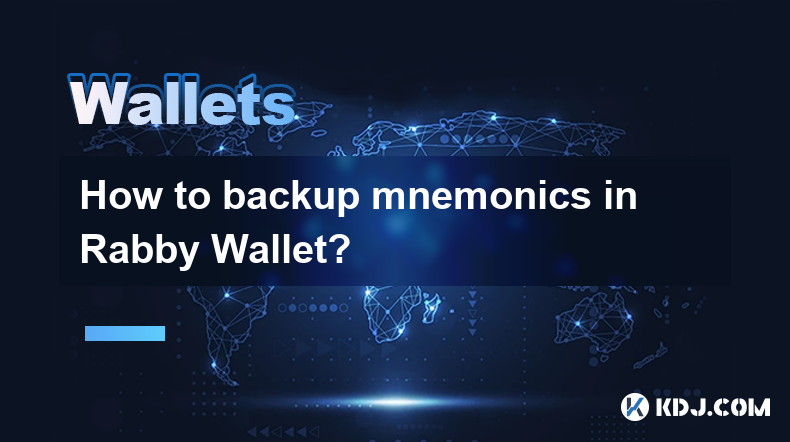
How to backup mnemonics in Rabby Wallet?
Apr 04,2025 at 02:21pm
Introduction to Rabby Wallet and MnemonicsRabby Wallet is a popular cryptocurrency wallet that offers users a secure way to manage their digital assets. One of the key features of Rabby Wallet is the use of mnemonics, which are a series of words that serve as a backup for your wallet. These mnemonics are crucial because they allow you to recover your wa...
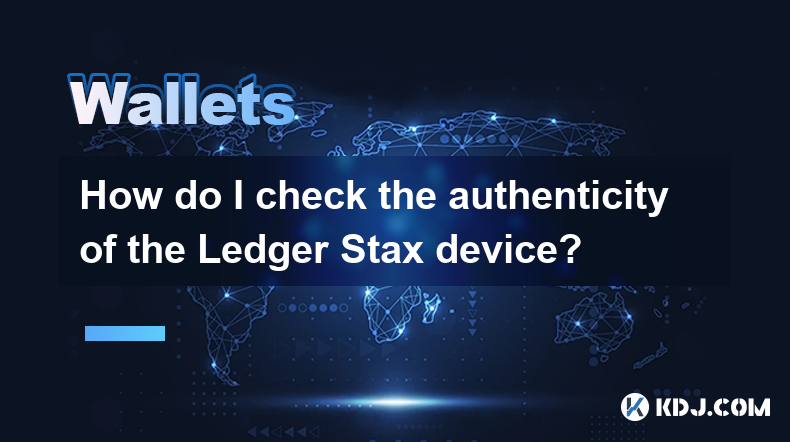
How do I check the authenticity of the Ledger Stax device?
Apr 04,2025 at 11:07am
Understanding the Importance of AuthenticityWhen investing in a hardware wallet like the Ledger Stax, ensuring its authenticity is crucial. A genuine device guarantees the security of your cryptocurrencies, protecting them from potential hacks and unauthorized access. Counterfeit devices can compromise your private keys, leading to significant financial...
See all articles
