-
Bitcoin
$87,160.3686
2.59% -
Ethereum
$1,577.5291
-0.58% -
Tether USDt
$1.0000
0.00% -
XRP
$2.0804
0.22% -
BNB
$596.3334
0.99% -
Solana
$136.5658
-0.15% -
USDC
$1.0000
0.00% -
Dogecoin
$0.1584
1.77% -
TRON
$0.2458
-0.32% -
Cardano
$0.6208
0.05% -
Chainlink
$13.1006
-1.96% -
UNUS SED LEO
$9.1412
-1.97% -
Avalanche
$19.9880
2.50% -
Stellar
$0.2528
3.56% -
Shiba Inu
$0.0...01238
-0.19% -
Toncoin
$2.8930
-3.79% -
Sui
$2.2031
4.44% -
Hedera
$0.1692
1.25% -
Bitcoin Cash
$343.2002
2.28% -
Polkadot
$3.8296
-1.99% -
Hyperliquid
$17.9378
1.39% -
Litecoin
$78.0777
0.75% -
Dai
$0.9999
0.00% -
Bitget Token
$4.4322
0.90% -
Ethena USDe
$0.9993
0.00% -
Pi
$0.6347
-0.88% -
Monero
$214.7110
-0.08% -
Uniswap
$5.2643
-0.22% -
Pepe
$0.0...07787
2.99% -
Aptos
$4.9970
-0.84%
How to write and deploy smart contracts on NFT platforms?
To create and launch NFTs, you must understand smart contracts, set up a development environment, write and test the contract, deploy it on a blockchain, and integrate with NFT platforms.
Apr 19, 2025 at 07:29 pm
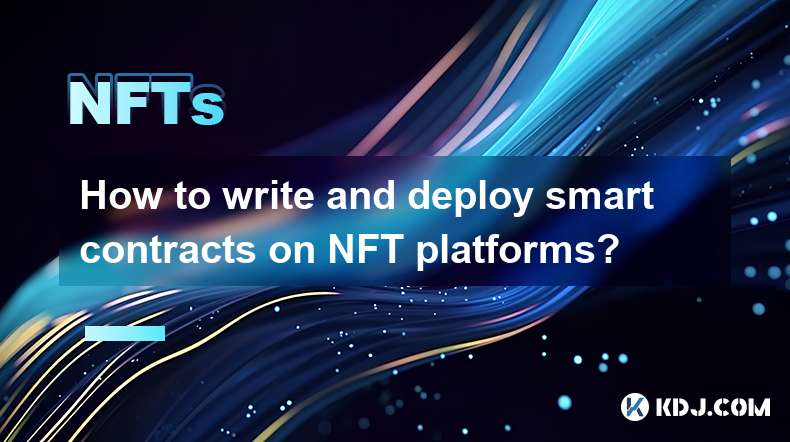
Writing and deploying smart contracts on NFT platforms involves several key steps, from understanding the basics of smart contracts to deploying them on a blockchain. This guide will walk you through the process in detail, ensuring you have a solid foundation to create and launch your NFTs.
Understanding Smart Contracts
Smart contracts are self-executing contracts with the terms of the agreement directly written into code. They run on blockchain technology, making them immutable and transparent. In the context of NFTs, smart contracts are used to define the rules and behaviors of the NFTs, such as ownership, transferability, and royalties.
To write a smart contract for an NFT, you typically need to use a programming language like Solidity, which is specifically designed for the Ethereum blockchain. However, other blockchains like Binance Smart Chain and Flow also support smart contracts with their respective languages.
Setting Up Your Development Environment
Before you start writing your smart contract, you need to set up your development environment. Here's how to do it:
Install Node.js and npm: Node.js is a JavaScript runtime, and npm is its package manager. You can download and install them from their official websites.
Set up Truffle: Truffle is a popular development framework for Ethereum. Install it using npm by running the command
npm install -g truffle
.Create a Truffle project: Run
truffle init
in your terminal to create a new Truffle project. This will set up the basic structure for your smart contract development.Install OpenZeppelin: OpenZeppelin is a library of secure smart contract components. Install it with
npm install @openzeppelin/contracts
.
Writing the Smart Contract
Now that your environment is set up, you can start writing your smart contract. Here's a basic example of an NFT smart contract using Solidity and OpenZeppelin:
pragma solidity ^0.8.0;import "@openzeppelin/contracts/token/ERC721/ERC721.sol";
import "@openzeppelin/contracts/utils/Counters.sol";
contract MyNFT is ERC721 {
using Counters for Counters.Counter;
Counters.Counter private _tokenIds;
constructor() ERC721("MyNFT", "NFT") {}
function mintNFT(address recipient, string memory tokenURI) public returns (uint256) {
_tokenIds.increment();
uint256 newItemId = _tokenIds.current();
_mint(recipient, newItemId);
_setTokenURI(newItemId, tokenURI);
return newItemId;
}
}
This contract defines an ERC721 token, which is the standard for NFTs on Ethereum. The mintNFT
function allows you to create new NFTs and assign them to a recipient.
Testing Your Smart Contract
Before deploying your smart contract, it's crucial to test it to ensure it works as expected. Truffle provides a testing framework that you can use:
- Write test cases: Create a new file in the
test
directory of your Truffle project. Here's an example test case:
const MyNFT = artifacts.require("MyNFT");contract("MyNFT", accounts => {
it("should mint an NFT", async () => {
const instance = await MyNFT.deployed();
const result = await instance.mintNFT(accounts[0], "https://example.com/tokenURI");
assert.equal(result.receipt.status, true, "NFT was not minted");
});
});
- Run the tests: Use the command
truffle test
to run your tests. This will execute the test cases and report any failures.
Deploying Your Smart Contract
Once your smart contract is tested and ready, you can deploy it to a blockchain. Here's how to deploy it using Truffle:
- Set up a deployment script: Create a new file in the
migrations
directory of your Truffle project. Here's an example:
const MyNFT = artifacts.require("MyNFT");module.exports = function(deployer) {
deployer.deploy(MyNFT);
};
Deploy to a test network: You can deploy to a test network like Rinkeby using Truffle. First, set up an
.env
file with your Infura project ID and a test account private key. Then, runtruffle migrate --network rinkeby
.Deploy to the mainnet: To deploy to the Ethereum mainnet, you need to set up a similar
.env
file with your mainnet account details. Runtruffle migrate --network mainnet
.
Interacting with Your Smart Contract
After deployment, you can interact with your smart contract using tools like Truffle Console or Web3.js. Here's how to use Truffle Console:
Open Truffle Console: Run
truffle console --network rinkeby
to open a console connected to the Rinkeby test network.Interact with the contract: You can call functions on your deployed contract. For example, to mint an NFT:
const instance = await MyNFT.deployed();
const result = await instance.mintNFT("0xYourAddress", "https://example.com/tokenURI");
console.log(result);
This will mint a new NFT and log the result to the console.
Integrating with NFT Platforms
To make your NFTs available on popular platforms like OpenSea, you need to follow their guidelines for smart contract integration. Here's how to do it for OpenSea:
Ensure ERC721 compliance: Your smart contract must comply with the ERC721 standard, which it does if you used the example above.
Add metadata: OpenSea requires metadata for each NFT, which you can set using the
tokenURI
in yourmintNFT
function.List your NFT on OpenSea: Once your smart contract is deployed and you've minted an NFT, you can list it on OpenSea by connecting your wallet and following their listing process.
Frequently Asked Questions
Q: Can I deploy my smart contract on multiple blockchains?
A: Yes, you can deploy your smart contract on multiple blockchains, but you'll need to adapt your code to the specific requirements of each blockchain. For example, Ethereum uses Solidity, while Binance Smart Chain uses a similar language called BEP-20.
Q: How do I handle gas fees when deploying my smart contract?
A: Gas fees are required to deploy smart contracts on Ethereum. You can estimate the gas cost using tools like Remix or Truffle, and you'll need to have enough ETH in your wallet to cover these fees. Some platforms like Polygon offer lower gas fees, which might be a good alternative.
Q: What are the common pitfalls to avoid when writing smart contracts for NFTs?
A: Common pitfalls include not handling edge cases, not implementing proper security measures, and not testing thoroughly. Always use established libraries like OpenZeppelin, and consider having your contract audited by a professional before deployment.
Q: Can I update my smart contract after deployment?
A: Smart contracts on Ethereum are immutable by design, meaning you can't update them after deployment. However, you can deploy a new version of your contract and migrate data from the old one to the new one if necessary.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- Time to Buy This PEPE and Shiba Inu Rival Before Price Explodes? Presale Goes Live, Combines AI + Football Narrative!
- 2025-04-22 04:30:13
- 72 crypto-related ETFs are currently waiting for approval from the U.S. Securities and Exchange Commission (SEC)
- 2025-04-22 04:30:13
- title: MANTRA Founder and CEO John Patrick Mullin Will Burn 150M OM Tokens Worth $82M to Rebuild Trust
- 2025-04-22 04:25:13
- MANTRA Burns 150 Million $OM Tokens to Boost Its Project Following a Sharp Market Decline
- 2025-04-22 04:25:13
- The cryptocurrency XRP is about to wake up from its slumber. An extreme tightening of the Bollinger Bands warns analysts: a volatility explosion is brewing.
- 2025-04-22 04:20:13
- Most Bitcoin Companies in El Salvador Are Now Dormant
- 2025-04-22 04:20:13
Related knowledge
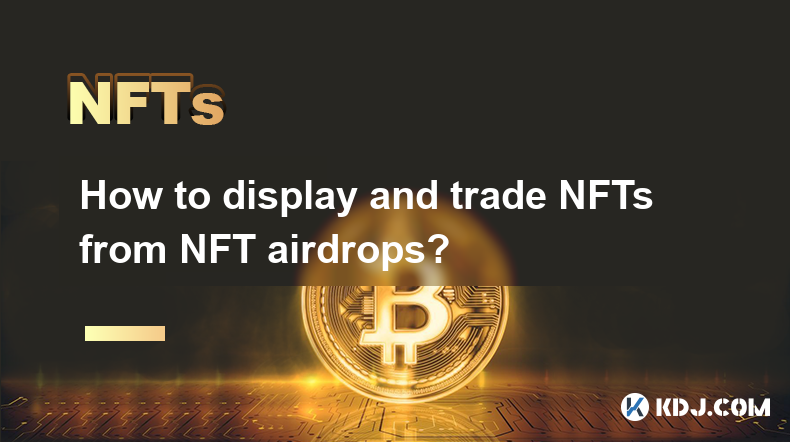
How to display and trade NFTs from NFT airdrops?
Apr 18,2025 at 04:42am
How to Display and Trade NFTs from NFT Airdrops? NFT airdrops have become a popular way for projects to distribute their tokens and engage with their community. If you've received NFTs through an airdrop, you might be wondering how to display and trade them. This article will guide you through the process step-by-step, ensuring you can showcase your NFT...
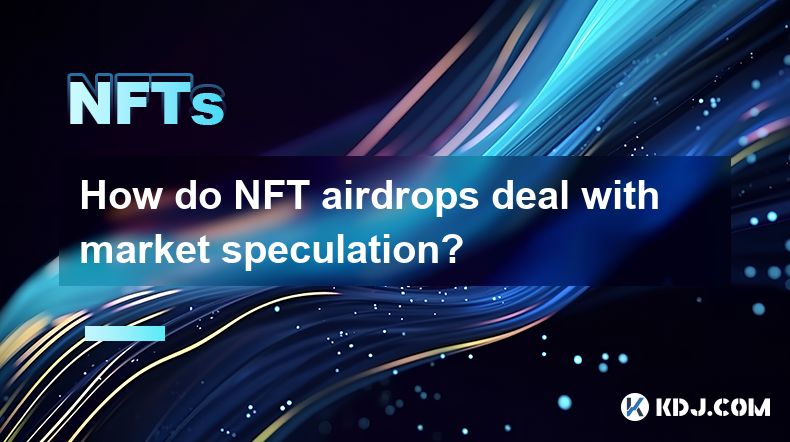
How do NFT airdrops deal with market speculation?
Apr 20,2025 at 10:28pm
NFT airdrops have become a significant phenomenon in the cryptocurrency space, often used as a marketing tool to distribute tokens or digital assets to a wide audience. However, they also introduce elements of market speculation that can impact the value and perception of NFTs. This article explores how NFT airdrops deal with market speculation, delving...
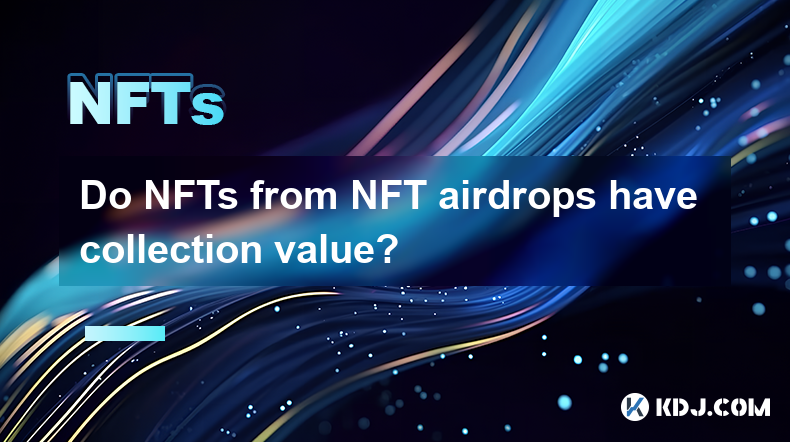
Do NFTs from NFT airdrops have collection value?
Apr 18,2025 at 11:49pm
NFTs, or non-fungible tokens, have become a significant part of the cryptocurrency ecosystem, and NFT airdrops are one way for projects to distribute these digital assets to their community. A common question that arises is whether NFTs received from airdrops have any collection value. To answer this question, we need to delve into various aspects of NF...
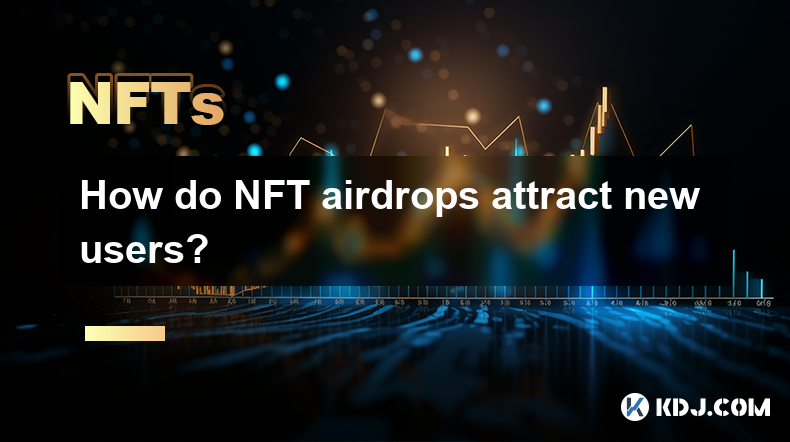
How do NFT airdrops attract new users?
Apr 21,2025 at 07:21am
NFT airdrops have become a popular strategy within the cryptocurrency community to attract new users and engage existing ones. By distributing free NFTs to a targeted audience, projects can create buzz, increase visibility, and foster a sense of community. This method leverages the allure of free digital assets to draw in participants who might not have...
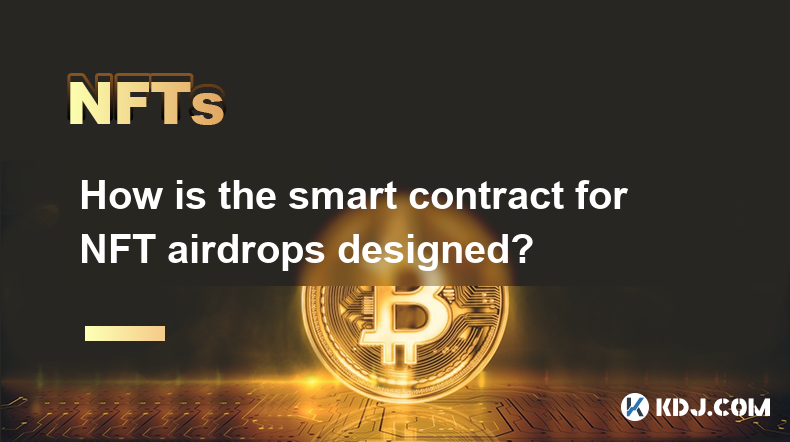
How is the smart contract for NFT airdrops designed?
Apr 18,2025 at 03:10am
The design of a smart contract for NFT airdrops is a complex process that requires careful consideration of various factors to ensure the airdrop is executed smoothly and securely. This article will delve into the intricacies of how such a smart contract is designed, focusing on key components, security measures, and the implementation process. Key Comp...
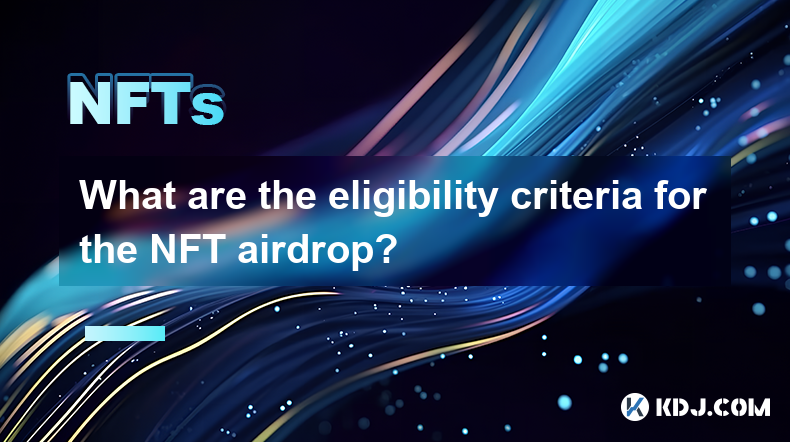
What are the eligibility criteria for the NFT airdrop?
Apr 17,2025 at 04:56pm
Understanding NFT AirdropsNFT airdrops are a popular method used by blockchain projects to distribute non-fungible tokens (NFTs) to their community members. These airdrops can serve various purposes, such as rewarding loyal users, promoting new projects, or increasing the visibility of existing ones. To participate in an NFT airdrop, individuals must me...
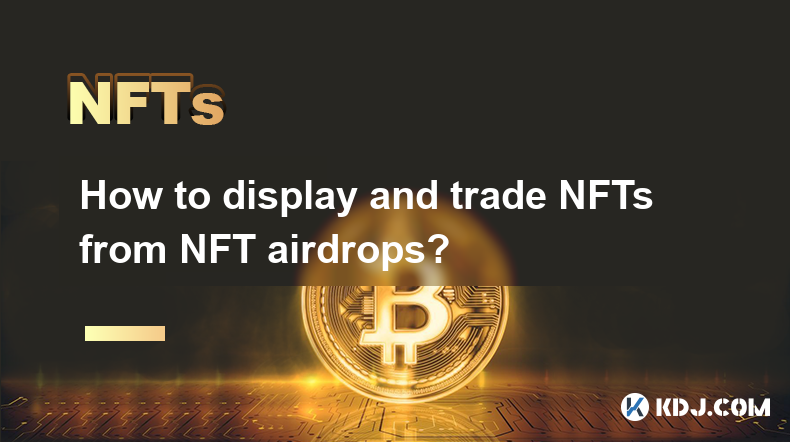
How to display and trade NFTs from NFT airdrops?
Apr 18,2025 at 04:42am
How to Display and Trade NFTs from NFT Airdrops? NFT airdrops have become a popular way for projects to distribute their tokens and engage with their community. If you've received NFTs through an airdrop, you might be wondering how to display and trade them. This article will guide you through the process step-by-step, ensuring you can showcase your NFT...
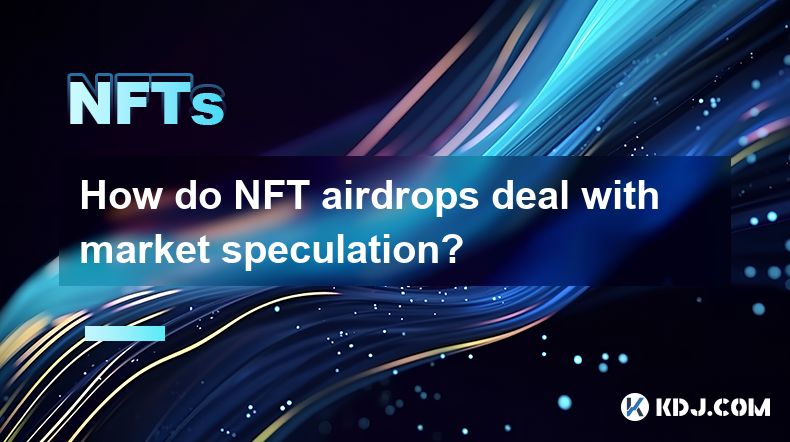
How do NFT airdrops deal with market speculation?
Apr 20,2025 at 10:28pm
NFT airdrops have become a significant phenomenon in the cryptocurrency space, often used as a marketing tool to distribute tokens or digital assets to a wide audience. However, they also introduce elements of market speculation that can impact the value and perception of NFTs. This article explores how NFT airdrops deal with market speculation, delving...
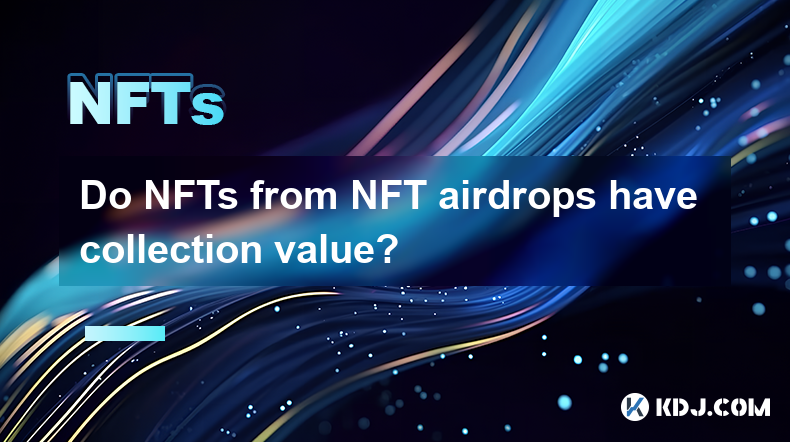
Do NFTs from NFT airdrops have collection value?
Apr 18,2025 at 11:49pm
NFTs, or non-fungible tokens, have become a significant part of the cryptocurrency ecosystem, and NFT airdrops are one way for projects to distribute these digital assets to their community. A common question that arises is whether NFTs received from airdrops have any collection value. To answer this question, we need to delve into various aspects of NF...
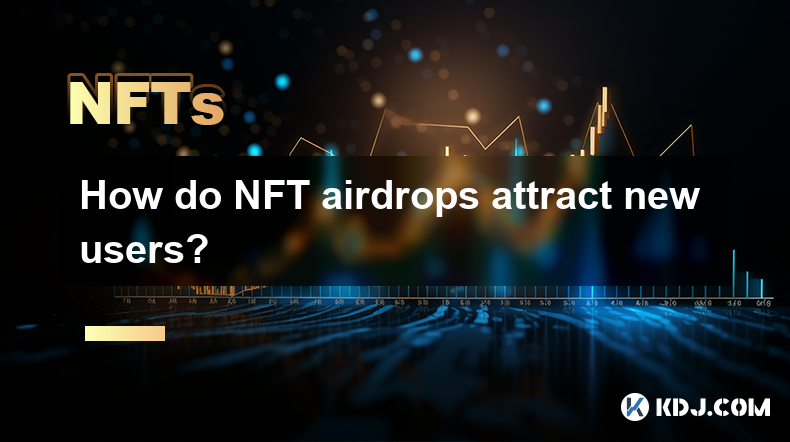
How do NFT airdrops attract new users?
Apr 21,2025 at 07:21am
NFT airdrops have become a popular strategy within the cryptocurrency community to attract new users and engage existing ones. By distributing free NFTs to a targeted audience, projects can create buzz, increase visibility, and foster a sense of community. This method leverages the allure of free digital assets to draw in participants who might not have...
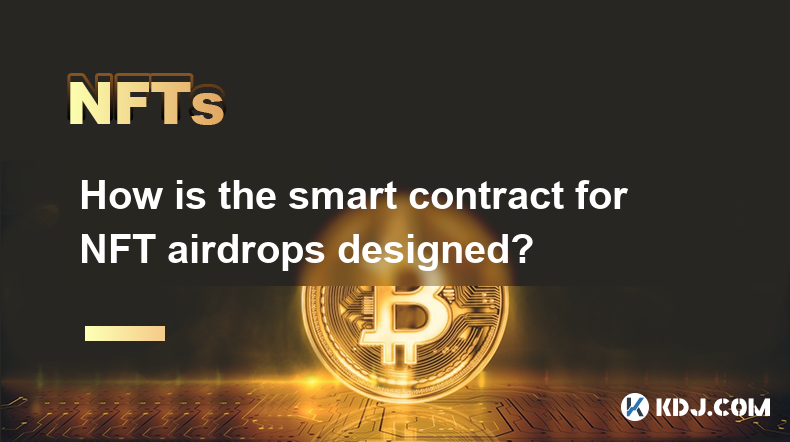
How is the smart contract for NFT airdrops designed?
Apr 18,2025 at 03:10am
The design of a smart contract for NFT airdrops is a complex process that requires careful consideration of various factors to ensure the airdrop is executed smoothly and securely. This article will delve into the intricacies of how such a smart contract is designed, focusing on key components, security measures, and the implementation process. Key Comp...
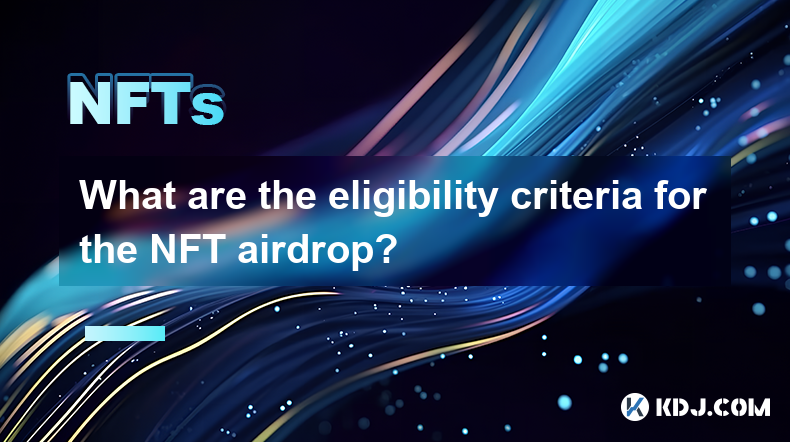
What are the eligibility criteria for the NFT airdrop?
Apr 17,2025 at 04:56pm
Understanding NFT AirdropsNFT airdrops are a popular method used by blockchain projects to distribute non-fungible tokens (NFTs) to their community members. These airdrops can serve various purposes, such as rewarding loyal users, promoting new projects, or increasing the visibility of existing ones. To participate in an NFT airdrop, individuals must me...
See all articles
