-
Bitcoin
$81,455.1592
5.51% -
Ethereum
$1,577.8864
7.50% -
Tether USDt
$0.9995
0.02% -
XRP
$2.0084
12.35% -
BNB
$578.0579
3.99% -
USDC
$0.9999
0.00% -
Solana
$113.2360
8.62% -
Dogecoin
$0.1564
7.25% -
TRON
$0.2406
5.28% -
Cardano
$0.6211
10.88% -
UNUS SED LEO
$9.4120
2.79% -
Chainlink
$12.3719
10.53% -
Avalanche
$18.2079
12.56% -
Toncoin
$2.9964
0.09% -
Hedera
$0.1756
18.45% -
Stellar
$0.2346
7.87% -
Sui
$2.1780
14.32% -
Shiba Inu
$0.0...01196
9.43% -
MANTRA
$6.5400
6.48% -
Bitcoin Cash
$295.0003
8.98% -
Litecoin
$73.8510
4.59% -
Polkadot
$3.5341
4.65% -
Dai
$1.0000
0.02% -
Bitget Token
$4.2861
5.97% -
Ethena USDe
$0.9987
0.02% -
Hyperliquid
$13.7356
12.70% -
Pi
$0.5960
5.53% -
Monero
$202.7897
3.63% -
Uniswap
$5.1632
7.98% -
OKB
$53.2342
3.44%
How to use API trading on Huobi
Using Huobi's API can automate your trading, but securing your API key with strong passwords and 2FA is crucial to protect your account.
Apr 03, 2025 at 08:14 pm
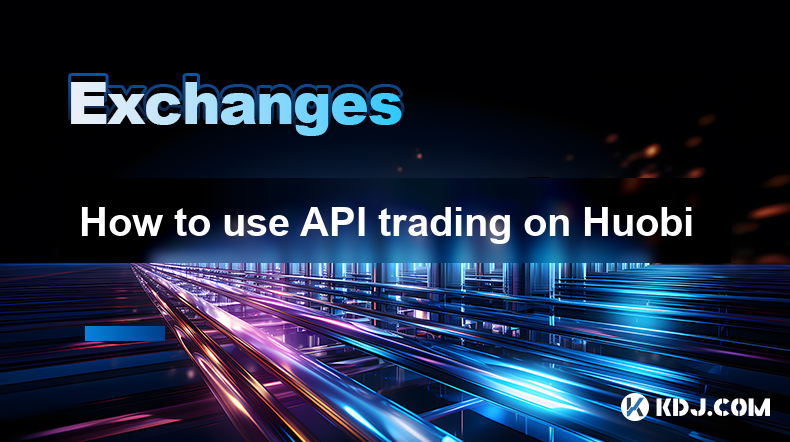
Using API trading on Huobi can significantly enhance your trading efficiency and automation. To get started, you first need to understand what an API is and how it works within the context of cryptocurrency trading. An API, or Application Programming Interface, allows different software systems to communicate with each other. In the case of Huobi, the API enables you to connect your trading algorithms or bots to the exchange, allowing you to execute trades, fetch market data, and manage your account programmatically.
To begin using the Huobi API, you'll need to create an API key. This involves navigating to the API Management section of your Huobi account. Here, you'll find options to generate new keys, which are crucial for securing your API interactions. Each key consists of an Access Key and a Secret Key. The Access Key is used to identify your account, while the Secret Key is used to sign your requests, ensuring that only you can execute trades on your behalf.
Setting Up Your API Key
To set up your API key, follow these steps:
- Log into your Huobi account.
- Navigate to the "API Management" section.
- Click on "Create New Key."
- Enter a label for your key to help you remember its purpose.
- Set permissions according to your needs, such as trading, withdrawal, or account management.
- Confirm your identity through two-factor authentication (2FA).
- Once created, you'll receive your Access Key and Secret Key. Keep the Secret Key safe and never share it.
After setting up your API key, you'll need to integrate it into your trading software or bot. Most trading platforms and bots will have specific fields where you can input your Access Key and Secret Key. Ensure that you're using a secure connection when entering these details to prevent unauthorized access.
Understanding API Endpoints
Huobi's API is divided into several endpoints, each serving a specific purpose. Some of the most commonly used endpoints include:
- Market Data Endpoints: These allow you to fetch real-time market data, such as price, volume, and order book information.
- Trading Endpoints: These enable you to place, cancel, and manage orders.
- Account Endpoints: These provide access to your account balance, deposit, and withdrawal information.
To use these endpoints effectively, you'll need to familiarize yourself with the API documentation provided by Huobi. The documentation outlines the structure of each request, the parameters you need to include, and the expected responses. It's crucial to understand these details to ensure that your API requests are successful.
Implementing API Requests
When implementing API requests, you'll need to use a programming language that supports HTTP requests. Popular choices include Python, JavaScript, and Java. Here's a basic example of how you might use Python to fetch the current price of a cryptocurrency:
import requests
import jsonaccess_key = 'your_access_key'
secret_key = 'your_secret_key'
url = 'https://api.huobi.pro/market/detail/merged?symbol=btcusdt'
response = requests.get(url)
data = json.loads(response.text)
print(data'tick')
This example fetches the current closing price of BTC/USDT. You can modify the symbol parameter to retrieve data for different trading pairs.
Managing Security and Risks
Security is paramount when using API trading. Here are some best practices to minimize risks:
- Use Strong Passwords: Ensure your Huobi account and any trading software you use have strong, unique passwords.
- Enable Two-Factor Authentication (2FA): Always use 2FA on your Huobi account to add an extra layer of security.
- Limit API Permissions: Only grant the necessary permissions to your API keys. For example, if you're only using the API for trading, don't enable withdrawal permissions.
- Monitor Your Account: Regularly check your account for any unauthorized activities and immediately revoke any compromised API keys.
- Use IP Whitelisting: Huobi allows you to whitelist specific IP addresses, adding another layer of security to your API interactions.
Automating Trading Strategies
One of the primary benefits of using API trading is the ability to automate your trading strategies. Whether you're implementing a simple moving average crossover strategy or a more complex machine learning model, the Huobi API can help you execute your trades automatically.
To automate a trading strategy, you'll need to:
- Define your trading logic in your chosen programming language.
- Use the Huobi API to fetch necessary market data.
- Implement decision-making algorithms based on your trading logic.
- Use the trading endpoints to place orders when your criteria are met.
Here's a simple example of an automated trading strategy using Python:
import requests
import json
import timeaccess_key = 'your_access_key'
secret_key = 'your_secret_key'
def get_price(symbol):
url = f'https://api.huobi.pro/market/detail/merged?symbol={symbol}'
response = requests.get(url)
data = json.loads(response.text)
return data['tick']['close']
def buy_order(symbol, amount):
# Implement buy order logic using Huobi's trading API
pass
def sell_order(symbol, amount):
# Implement sell order logic using Huobi's trading API
pass
while True:
btc_price = get_price('btcusdt')
if btc_price > 50000: # Example condition
buy_order('btcusdt', 0.1)
elif btc_price < 45000: # Example condition
sell_order('btcusdt', 0.1)
time.sleep(60) # Wait for 1 minute before checking again
This example demonstrates a basic automated trading strategy that buys Bitcoin when the price exceeds $50,000 and sells when it drops below $45,000. You can expand on this by incorporating more sophisticated logic and risk management techniques.
Handling Errors and Exceptions
When using the Huobi API, you'll inevitably encounter errors and exceptions. It's crucial to handle these gracefully to ensure your trading operations continue smoothly. Common errors include rate limiting, invalid parameters, and server errors. Here are some tips for handling errors:
- Implement Retry Logic: If you encounter a rate limit error, implement a retry mechanism with exponential backoff to avoid overwhelming the API.
- Validate Parameters: Before sending a request, validate your parameters to ensure they meet the API's requirements.
- Log Errors: Keep a log of all errors and exceptions to help diagnose issues and improve your trading software.
Here's an example of how you might handle errors in Python:
import requests
import json
import time
def get_price_with_retry(symbol, max_retries=3):
for attempt in range(max_retries):
try:
url = f'https://api.huobi.pro/market/detail/merged?symbol={symbol}'
response = requests.get(url)
response.raise_for_status() # Raise an exception for bad status codes
data = json.loads(response.text)
return data['tick']['close']
except requests.exceptions.RequestException as e:
if attempt < max_retries - 1:
wait_time = (2 ** attempt) # Exponential backoff
time.sleep(wait_time)
else:
raise e # Re-raise the exception if all retries fail
btc_price = get_price_with_retry('btcusdt')
print(btc_price)
This example demonstrates how to implement retry logic with exponential backoff when fetching market data.
Optimizing Performance
To optimize the performance of your API trading, consider the following strategies:
- Batch Requests: Where possible, use batch requests to reduce the number of API calls you need to make. Huobi's API supports batch operations for certain endpoints.
- Caching: Implement caching mechanisms to store frequently accessed data, reducing the need for repeated API calls.
- Asynchronous Programming: Use asynchronous programming techniques to handle multiple API requests concurrently, improving the overall efficiency of your trading software.
Here's an example of using asynchronous programming in Python to fetch multiple prices simultaneously:
import asyncio
import aiohttp
import jsonasync def get_price(session, symbol):
url = f'https://api.huobi.pro/market/detail/merged?symbol={symbol}'
async with session.get(url) as response:
data = await response.json()
return data['tick']['close']
async def main():
async with aiohttp.ClientSession() as session:
tasks = [
get_price(session, 'btcusdt'),
get_price(session, 'ethusdt'),
get_price(session, 'ltcusdt')
]
prices = await asyncio.gather(*tasks)
print(prices)
asyncio.run(main())
This example demonstrates how to use asynchronous programming to fetch prices for multiple trading pairs concurrently, improving performance.
Monitoring and Analytics
Monitoring your API trading activities is essential for optimizing your strategies and ensuring everything is running smoothly. Huobi provides various tools and endpoints for monitoring your trades and account activity. Additionally, you can implement your own monitoring and analytics solutions to track performance metrics and identify areas for improvement.
Some key metrics to monitor include:
- Trade Volume: Monitor the volume of trades executed through your API to understand your trading activity.
- Profit and Loss (P&L): Track your P&L to assess the performance of your trading strategies.
- API Usage: Monitor your API usage to ensure you're not hitting rate limits and to optimize your API calls.
Here's an example of how you might implement basic monitoring in Python:
import requests
import json
import timedef get_account_balance():
url = 'https://api.huobi.pro/v1/account/accounts'
response = requests.get(url)
data = json.loads(response.text)
return data['data'][0]['balance']
def monitor_trades():
while True:
balance = get_account_balance()
print(f'Current Balance: {balance}')
time.sleep(300) # Check every 5 minutes
monitor_trades()
This example demonstrates a simple monitoring script that periodically checks your account balance.
Common Questions
Q: What is an API key, and why is it important for Huobi trading?
A: An API key is a unique identifier used to authenticate your requests to the Huobi API. It consists of an Access Key and a Secret Key. The Access Key identifies your account, while the Secret Key is used to sign your requests, ensuring security. It's crucial for trading because it allows you to automate your trading strategies and manage your account programmatically.
Q: How can I secure my API key on Huobi?
A: To secure your API key on Huobi, use strong passwords, enable two-factor authentication (2FA), limit API permissions to only what's necessary, monitor your account regularly, and consider using IP whitelisting to restrict access to your API keys.
Q: What are some common API endpoints used for trading on Huobi?
A: Some common API endpoints used for trading on Huobi include market data endpoints for fetching real-time market data, trading endpoints for placing and managing orders, and account endpoints for accessing your account balance and managing deposits and withdrawals.
Q: How can I automate my trading strategies using the Huobi API?
A: To automate trading strategies using the Huobi API, you need to define your trading logic in a programming language, use the API to fetch necessary market data, implement decision-making algorithms, and use the trading endpoints to place orders when your criteria are met. You can also implement error handling, performance optimization, and monitoring to enhance your automated trading system.
Q: What are some best practices for handling errors when using the Huobi API?
A: Best practices for handling errors when using the Huobi API include implementing retry logic with exponential backoff, validating parameters before sending requests, logging errors for diagnostics, and using asynchronous programming to handle multiple requests concurrently.
Q: How can I optimize the performance of my API trading on Huobi?
A: To optimize the performance of your API trading on Huobi, use batch requests to reduce API calls, implement caching to store frequently accessed data, and use asynchronous programming to handle multiple requests concurrently. Monitoring your API usage and optimizing your trading logic can also improve performance.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- GameStop Adds Bitcoin to Its Treasury Reserves
- 2025-04-10 21:35:18
- XRPTurbo ($XRT) is a revolutionary launchpad being built specifically to turbocharge decentralized finance (DeFi) on the XRP blockchain.
- 2025-04-10 21:35:17
- Trump Media Expands into Finance with ETF Collaboration
- 2025-04-10 21:30:19
- Arthur Hayes Predicts Ethereum Will Hit $5k Before Solana Reaches $300
- 2025-04-10 21:30:19
- As we approach the festive season, the value of the crypto coin market begins to gain momentum, and several coins grab the attention of investors.
- 2025-04-10 21:20:13
- Pi Network Faces Significant Hurdles on Its Journey to Listing
- 2025-04-10 21:20:13
Related knowledge
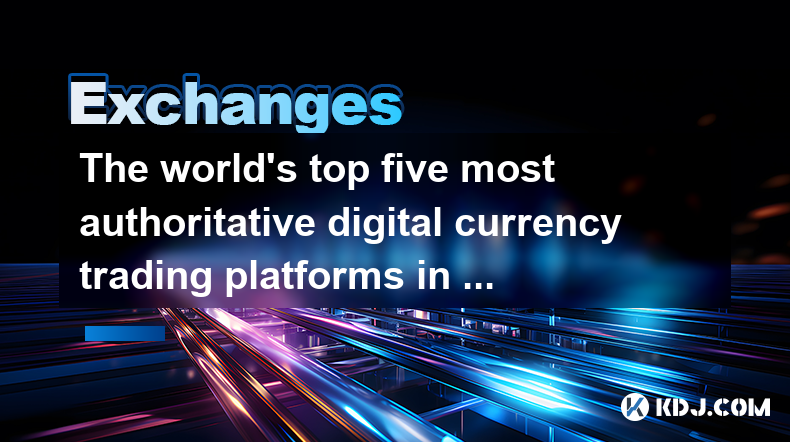
The world's top five most authoritative digital currency trading platforms in 2025
Apr 10,2025 at 02:23pm
With the continuous development and evolution of the market, different trading platforms have shown different advantages in dimensions such as security, trading variety richness, transaction costs, and user services. The following shows you the five most authoritative digital currency trading platforms in the world in 2025. These platforms have won wide...
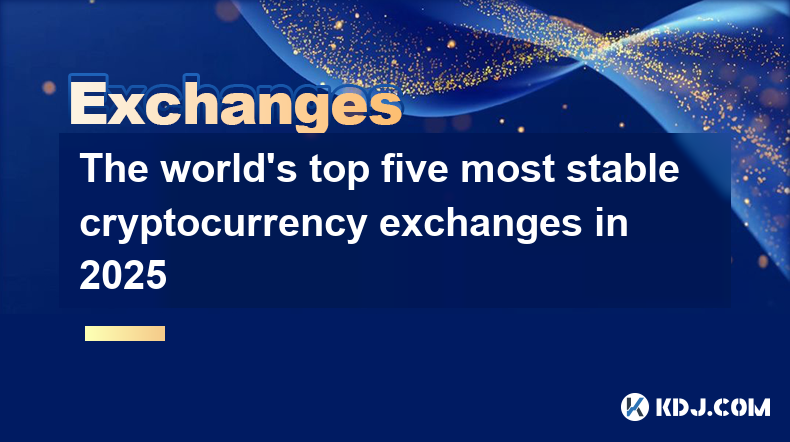
The world's five most stable cryptocurrency exchanges in 2025
Apr 10,2025 at 02:20pm
In the field of digital currency trading, security and stability have always been the core elements that investors pay attention to. With the continuous evolution of the market, various trading platforms continue to strengthen their own strength and strive to provide users with a reliable trading environment. The following are the five most stable digit...
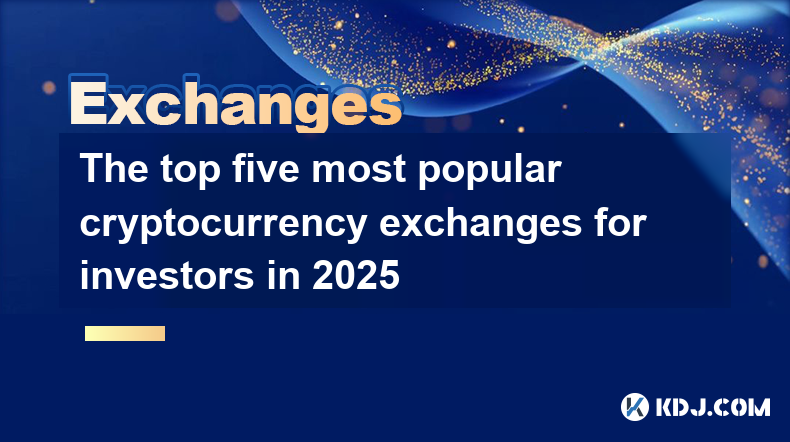
The top five most popular cryptocurrency exchanges for investors in 2025
Apr 10,2025 at 02:11pm
With the continuous development of the digital currency market in 2025, many exchanges have shown their strengths. The following is a detailed introduction to the five most popular digital currency exchanges among investors in 2025. They performed outstandingly in terms of security, trading products, liquidity, trading fees and user experience, providin...
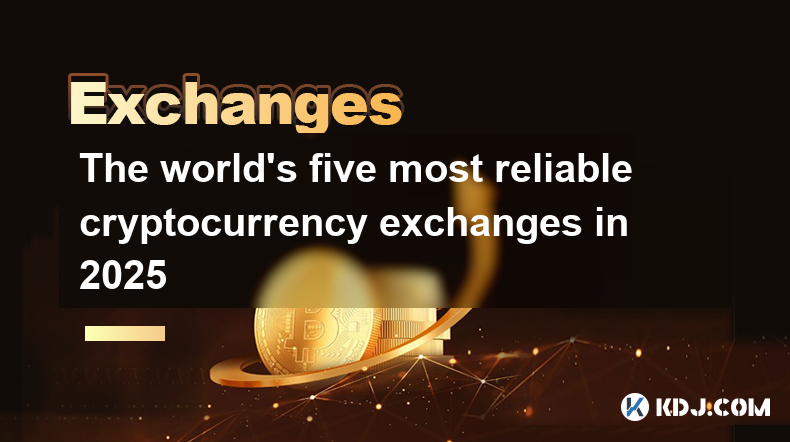
The world's five most reliable cryptocurrency exchanges in 2025
Apr 10,2025 at 01:41pm
In 2025, the global cryptocurrency trading platform pattern continues to change, taking into account many factors such as security, liquidity, trading products, and user experience. The following are the five most reliable cryptocurrency trading platforms in the world: 1. BinanceBinance is the world's leading cryptocurrency trading platform, and is ...
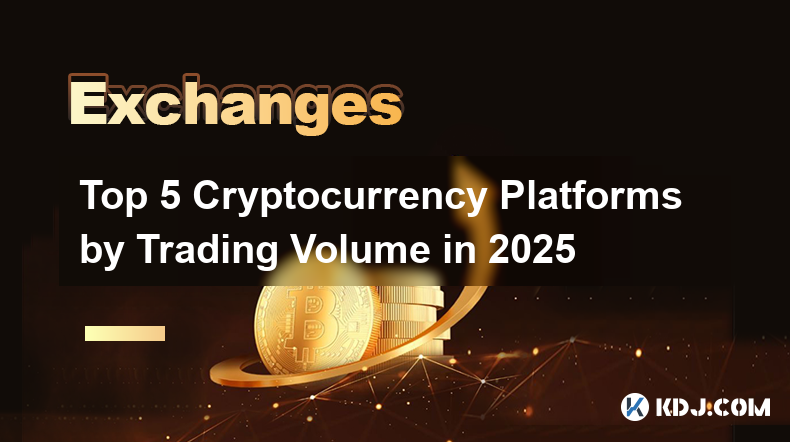
Top 5 Cryptocurrency Platforms by Trading Volume in 2025
Apr 10,2025 at 01:37pm
In the cryptocurrency field in 2025, the pattern of trading platforms has evolved, and trading volume has become a key indicator for measuring their influence and activity. Many platforms are highly competitive. Here are the top five cryptocurrency platforms with the highest trading volume in the world in 2025. These platforms have taken the lead in the...
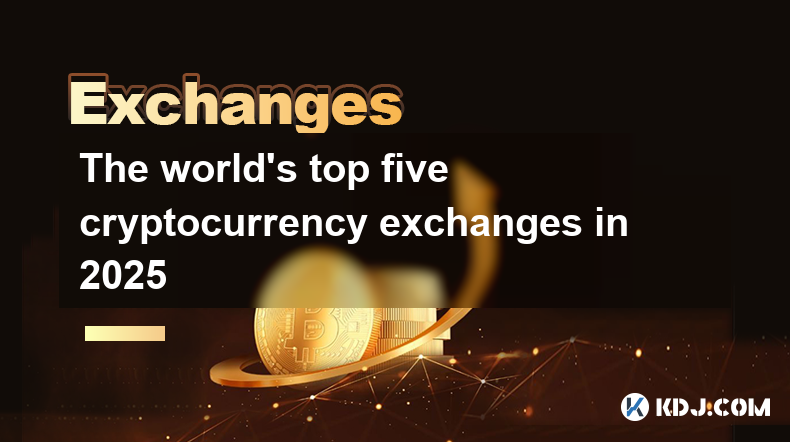
The world's top five cryptocurrency exchanges in 2025
Apr 10,2025 at 01:33pm
In the ever-changing cryptocurrency market, cryptocurrency exchanges serve as a key bridge connecting investors with digital assets, and their importance is self-evident. In 2025, cryptocurrency trading activities around the world continue to be active, and major exchanges are also constantly innovating and developing in the fierce competition. The foll...
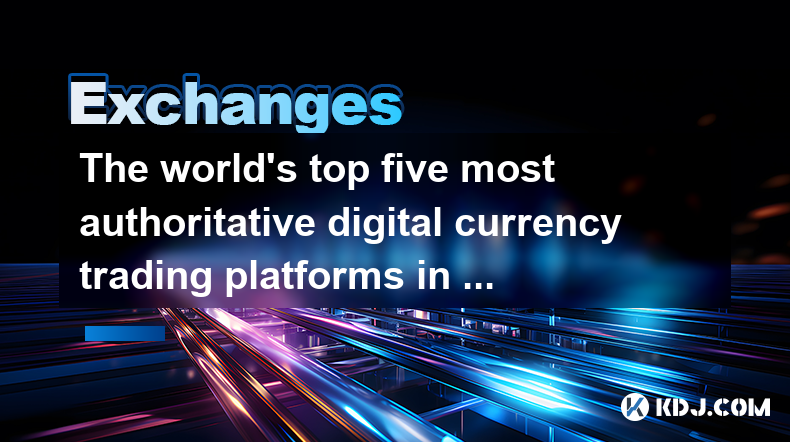
The world's top five most authoritative digital currency trading platforms in 2025
Apr 10,2025 at 02:23pm
With the continuous development and evolution of the market, different trading platforms have shown different advantages in dimensions such as security, trading variety richness, transaction costs, and user services. The following shows you the five most authoritative digital currency trading platforms in the world in 2025. These platforms have won wide...
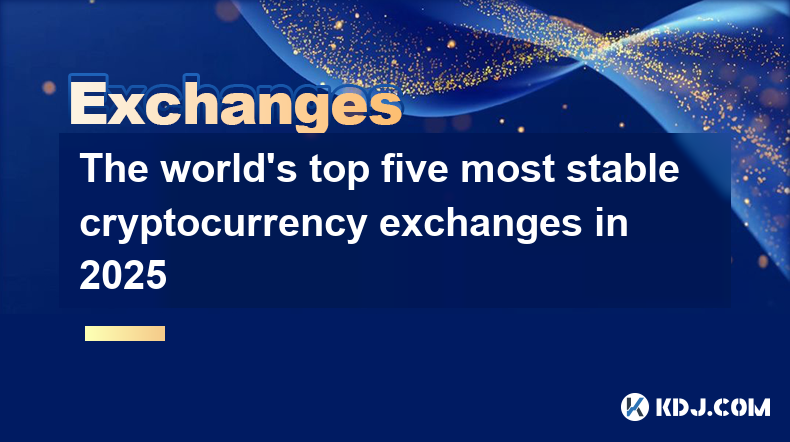
The world's five most stable cryptocurrency exchanges in 2025
Apr 10,2025 at 02:20pm
In the field of digital currency trading, security and stability have always been the core elements that investors pay attention to. With the continuous evolution of the market, various trading platforms continue to strengthen their own strength and strive to provide users with a reliable trading environment. The following are the five most stable digit...
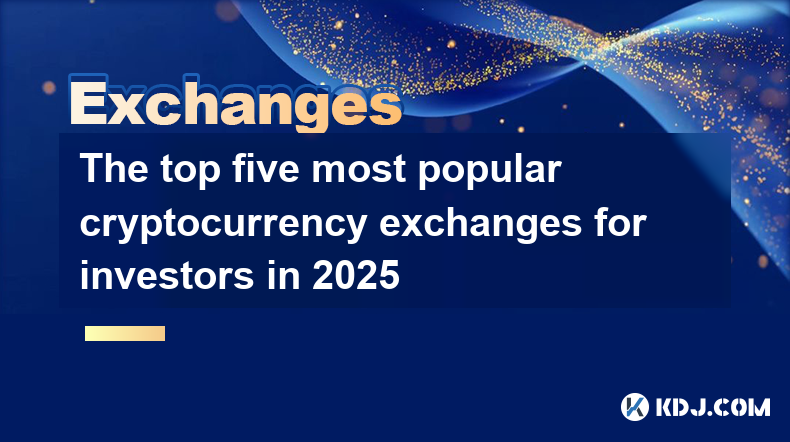
The top five most popular cryptocurrency exchanges for investors in 2025
Apr 10,2025 at 02:11pm
With the continuous development of the digital currency market in 2025, many exchanges have shown their strengths. The following is a detailed introduction to the five most popular digital currency exchanges among investors in 2025. They performed outstandingly in terms of security, trading products, liquidity, trading fees and user experience, providin...
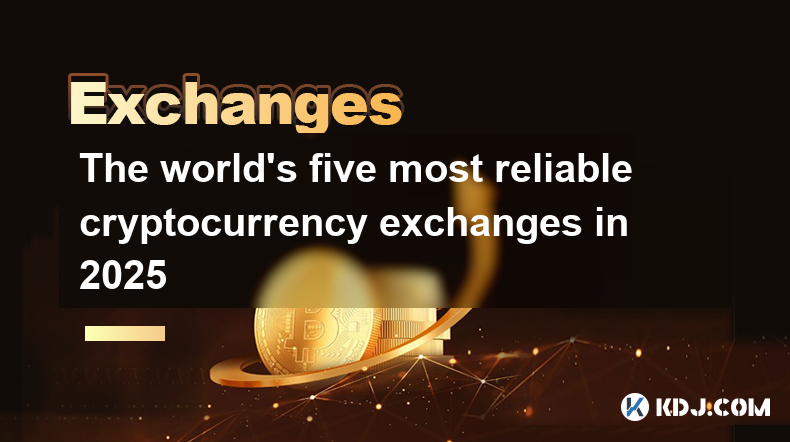
The world's five most reliable cryptocurrency exchanges in 2025
Apr 10,2025 at 01:41pm
In 2025, the global cryptocurrency trading platform pattern continues to change, taking into account many factors such as security, liquidity, trading products, and user experience. The following are the five most reliable cryptocurrency trading platforms in the world: 1. BinanceBinance is the world's leading cryptocurrency trading platform, and is ...
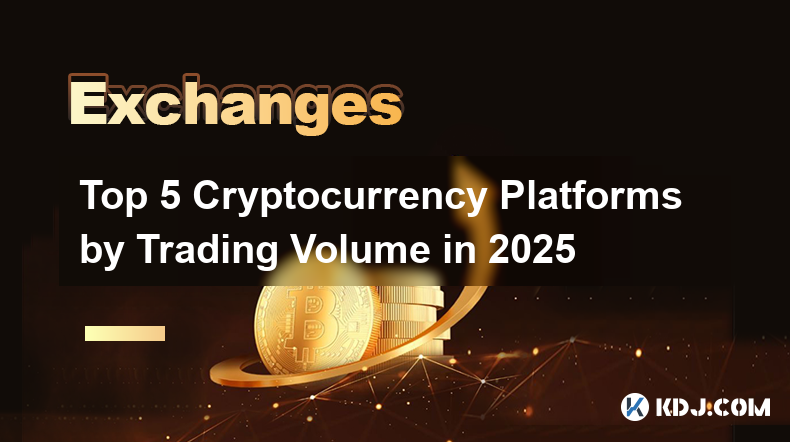
Top 5 Cryptocurrency Platforms by Trading Volume in 2025
Apr 10,2025 at 01:37pm
In the cryptocurrency field in 2025, the pattern of trading platforms has evolved, and trading volume has become a key indicator for measuring their influence and activity. Many platforms are highly competitive. Here are the top five cryptocurrency platforms with the highest trading volume in the world in 2025. These platforms have taken the lead in the...
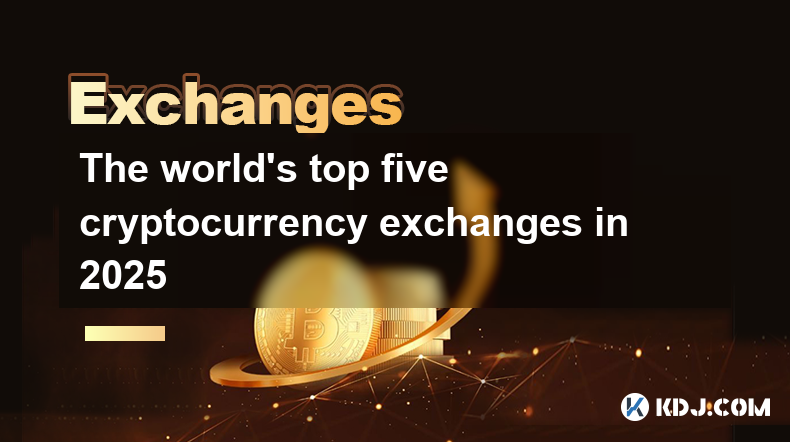
The world's top five cryptocurrency exchanges in 2025
Apr 10,2025 at 01:33pm
In the ever-changing cryptocurrency market, cryptocurrency exchanges serve as a key bridge connecting investors with digital assets, and their importance is self-evident. In 2025, cryptocurrency trading activities around the world continue to be active, and major exchanges are also constantly innovating and developing in the fierce competition. The foll...
See all articles
