-
Bitcoin
$87,183.0218
2.51% -
Ethereum
$1,574.7724
-0.66% -
Tether USDt
$1.0000
0.01% -
XRP
$2.0751
0.16% -
BNB
$596.5259
0.77% -
Solana
$135.7967
-1.16% -
USDC
$0.9999
-0.01% -
Dogecoin
$0.1584
2.14% -
TRON
$0.2465
0.57% -
Cardano
$0.6201
-0.07% -
Chainlink
$13.0374
-2.17% -
UNUS SED LEO
$9.1386
-2.00% -
Avalanche
$19.7756
1.73% -
Stellar
$0.2503
2.79% -
Shiba Inu
$0.0...01233
-0.26% -
Toncoin
$2.8831
-4.01% -
Hedera
$0.1684
1.37% -
Sui
$2.1872
3.82% -
Bitcoin Cash
$344.2873
2.30% -
Hyperliquid
$18.0531
3.76% -
Polkadot
$3.7760
-2.94% -
Litecoin
$77.6272
-0.57% -
Dai
$0.9999
0.00% -
Bitget Token
$4.4329
0.59% -
Ethena USDe
$0.9993
0.00% -
Pi
$0.6345
-0.88% -
Monero
$215.1085
0.19% -
Uniswap
$5.2560
0.34% -
Pepe
$0.0...07738
2.61% -
OKB
$50.9648
1.42%
How to automate the buying and selling of AVAX through the API?
Automate AVAX trading using APIs from exchanges like Binance or Kraken, setting up scripts with Python and ccxt to buy below $30 and sell above $35.
Apr 21, 2025 at 02:56 pm
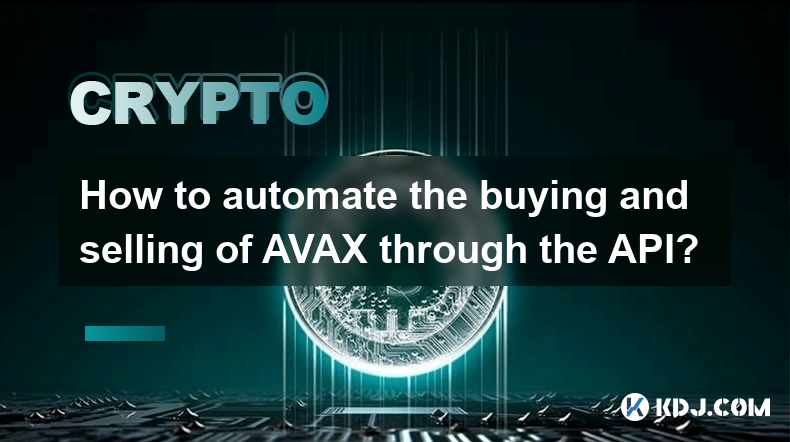
How to Automate the Buying and Selling of AVAX through the API?
Automating the buying and selling of cryptocurrencies like AVAX can streamline your trading process, allowing you to execute trades based on pre-set conditions without manual intervention. This guide will walk you through the steps needed to set up an automated trading system for AVAX using an API.
Choosing the Right Exchange and API
To automate AVAX trades, you'll need to select an exchange that supports AVAX trading and offers a robust API. Popular exchanges like Binance, Coinbase Pro, and Kraken are suitable options. Each exchange has its own API documentation, so it's important to choose one that aligns with your trading needs.
- Binance: Known for its extensive trading pairs and high liquidity, Binance offers a comprehensive API that supports both spot and futures trading.
- Coinbase Pro: Offers a user-friendly API with good documentation, suitable for beginners.
- Kraken: Known for its security and support for a wide range of cryptocurrencies, including AVAX.
Once you've chosen an exchange, you'll need to register for an API key. This key will allow your trading bot to interact with the exchange on your behalf.
Setting Up Your API Key
To set up your API key, follow these steps:
- Log into your exchange account and navigate to the API section.
- Generate a new API key. You'll typically be asked to provide a name for the key and set permissions. For trading AVAX, you'll need to enable permissions for trading and account balance access.
- Save your API key and secret. These will be used in your trading script to authenticate your requests.
Choosing a Programming Language and Library
Next, you'll need to choose a programming language and a library to interact with the API. Python is a popular choice due to its simplicity and the availability of libraries like ccxt
and Binance API
.
- ccxt: A JavaScript / Python / PHP library for cryptocurrency trading and e-commerce with support for many bitcoin/ether/altcoin exchange markets and merchant APIs.
- Binance API: A Python library specifically designed for interacting with the Binance API.
For this example, we'll use Python and the ccxt
library.
Writing the Trading Script
Now, let's write a basic trading script to automate the buying and selling of AVAX. This script will use a simple strategy: buy AVAX when its price drops below a certain threshold and sell when it rises above another threshold.
Here's a sample script using ccxt
:
import ccxtInitialize the exchange
exchange = ccxt.binance({
'apiKey': 'YOUR_API_KEY',
'secret': 'YOUR_SECRET_KEY',
})
Define trading parameters
buy_threshold = 30 # Buy when AVAX price drops below $30
sell_threshold = 35 # Sell when AVAX price rises above $35
while True:
# Fetch the current AVAX/USDT price
ticker = exchange.fetch_ticker('AVAX/USDT')
current_price = ticker['last']
# Check if the current price meets our buy condition
if current_price < buy_threshold:
# Place a market buy order for 1 AVAX
order = exchange.create_market_buy_order('AVAX/USDT', 1)
print(f'Bought 1 AVAX at {current_price}')
# Check if the current price meets our sell condition
elif current_price > sell_threshold:
# Place a market sell order for 1 AVAX
order = exchange.create_market_sell_order('AVAX/USDT', 1)
print(f'Sold 1 AVAX at {current_price}')
# Wait for a short period before checking again
time.sleep(60) # Wait for 1 minute
This script will continuously monitor the AVAX price and execute trades based on the defined thresholds.
Implementing Risk Management
To ensure your trading strategy is sustainable, it's crucial to implement risk management techniques. Here are some key considerations:
- Stop-Loss Orders: Set a stop-loss order to automatically sell AVAX if its price drops below a certain level, limiting potential losses.
- Take-Profit Orders: Set a take-profit order to automatically sell AVAX if its price rises above a certain level, locking in profits.
- Position Sizing: Determine the size of your trades based on your total capital to manage risk effectively.
Here's how you can modify the script to include a stop-loss:
import ccxt
exchange = ccxt.binance({
'apiKey': 'YOUR_API_KEY',
'secret': 'YOUR_SECRET_KEY',
})
buy_threshold = 30
sell_threshold = 35
stop_loss = 28 # Stop-loss at $28
while True:
ticker = exchange.fetch_ticker('AVAX/USDT')
current_price = ticker['last']
if current_price < buy_threshold:
order = exchange.create_market_buy_order('AVAX/USDT', 1)
print(f'Bought 1 AVAX at {current_price}')
# Set a stop-loss order
stop_loss_order = exchange.create_order('AVAX/USDT', 'stop_loss', 'sell', 1, stop_loss)
print(f'Set stop-loss at {stop_loss}')
elif current_price > sell_threshold:
order = exchange.create_market_sell_order('AVAX/USDT', 1)
print(f'Sold 1 AVAX at {current_price}')
time.sleep(60)
Testing and Backtesting Your Strategy
Before deploying your trading script in a live environment, it's essential to test and backtest your strategy. Testing helps identify any bugs or errors in your code, while backtesting allows you to evaluate the performance of your strategy using historical data.
- Testing: Run your script in a simulated environment or with a small amount of capital to ensure it functions as expected.
- Backtesting: Use historical price data to simulate how your strategy would have performed in the past. Libraries like
backtrader
or zipline
can be used for backtesting in Python.
Here's a simple example of how you might backtest your strategy using historical data:
import pandas as pd
import ccxt
exchange = ccxt.binance()
ohlcv = exchange.fetch_ohlcv('AVAX/USDT', '1d')
df = pd.DataFrame(ohlcv, columns=['timestamp', 'open', 'high', 'low', 'close', 'volume'])
df['timestamp'] = pd.to_datetime(df['timestamp'], unit='ms')
buy_threshold = 30
sell_threshold = 35
stop_loss = 28
position = 0
balance = 1000 # Starting balance in USDT
for index, row in df.iterrows():
current_price = row['close']
if position == 0 and current_price < buy_threshold:
position = 1
buy_price = current_price
balance -= buy_price
print(f'Bought 1 AVAX at {buy_price}. Balance: {balance}')
elif position == 1:
if current_price > sell_threshold:
position = 0
sell_price = current_price
balance += sell_price
print(f'Sold 1 AVAX at {sell_price}. Balance: {balance}')
elif current_price < stop_loss:
position = 0
sell_price = stop_loss
balance += sell_price
print(f'Stop-loss triggered. Sold 1 AVAX at {sell_price}. Balance: {balance}')
print(f'Final balance: {balance}')
Deploying Your Trading Bot
Once you're satisfied with your strategy's performance, you can deploy your trading bot. Consider the following options:
- Local Deployment: Run your script on your local machine. This is suitable for testing but may not be reliable for long-term use due to potential downtime.
- Cloud Deployment: Use cloud services like AWS, Google Cloud, or DigitalOcean to host your trading bot. This ensures your bot runs continuously and can be easily scaled.
To deploy on a cloud service, you'll need to:
- Set up a virtual machine or a container service.
- Install the necessary dependencies, including Python and the
ccxt
library. - Upload your trading script and configure it to run automatically.
Here's a basic example of how to set up a cron job on a Linux-based system to run your script every minute:
crontab -e
Add the following line to your crontab file:
* /usr/bin/python3 /path/to/your/script.py
Monitoring and Maintenance
After deploying your trading bot, it's important to monitor its performance and maintain it regularly. Set up alerts to notify you of significant price movements or unexpected behavior. Regularly review your trading logs and adjust your strategy as needed based on market conditions.
Frequently Asked Questions
Q: Can I use the same script to trade other cryptocurrencies?
A: Yes, you can modify the script to trade other cryptocurrencies by changing the trading pair in the fetch_ticker
and create_order
functions. For example, to trade ETH/USDT, you would use 'ETH/USDT' instead of 'AVAX/USDT'.
Q: How do I handle API rate limits?
A: Exchanges have rate limits to prevent abuse. To handle these, you can implement a delay between API calls or use the exchange's built-in rate limit handling features. For example, ccxt
has a rateLimit
parameter that can be adjusted.
Q: Is it safe to store my API keys in the script?
A: Storing API keys directly in your script is not recommended due to security risks. Instead, use environment variables or a secure configuration file to store your keys. This way, your keys are not exposed if your script is shared or compromised.
Q: How can I improve the performance of my trading strategy?
A: To improve your strategy, consider incorporating more advanced indicators and technical analysis. You can also use machine learning models to predict price movements and adjust your thresholds dynamically based on market conditions.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- Coinbase Lists Reserve Rights (RSR), a Dual-Token Stablecoin Platform Aimed at Creating a Collateral-Backed, Self-Regulating Stablecoin Ecosystem
- 2025-04-22 06:40:13
- The Ultimate Guide to Capital Rotation in the Cryptocurrency Market
- 2025-04-22 06:40:13
- Mantra's CEO Keeps His Word – Massive Tokens Burned: Will $OM Price Go From Collapse to Catalyst?
- 2025-04-22 06:35:14
- TRUMP Token Price Soars After Unlocking 40M New Coins
- 2025-04-22 06:35:14
- Avalanche Foundation Launches the Avalanche Card, Allowing Holders to Access Their Crypto Assets Seamlessly Around the World
- 2025-04-22 06:30:12
- The OM Token Crash: A Stark Reminder of Crypto's Wild West Nature
- 2025-04-22 06:30:12
Related knowledge
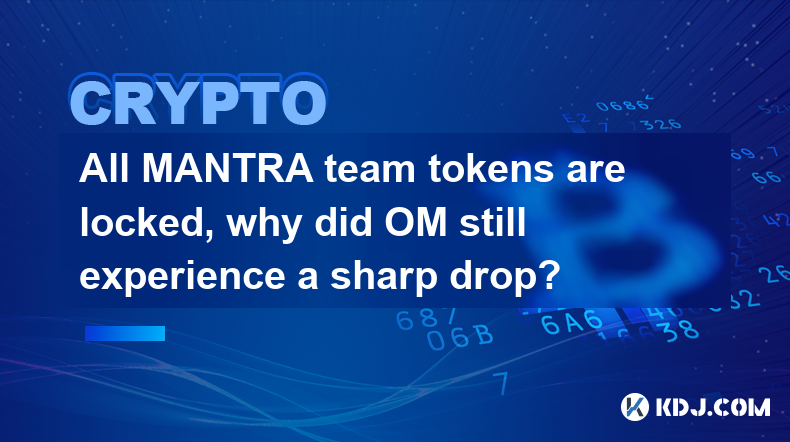
All MANTRA team tokens are locked, why did OM still experience a sharp drop?
Apr 20,2025 at 11:14am
Introduction to MANTRA and OM TokenThe MANTRA project is a blockchain platform that aims to provide a scalable and secure environment for decentralized applications (dApps). The native token of the MANTRA ecosystem is OM, which plays a crucial role in governance, staking, and other functionalities within the platform. Recently, the MANTRA team announced...
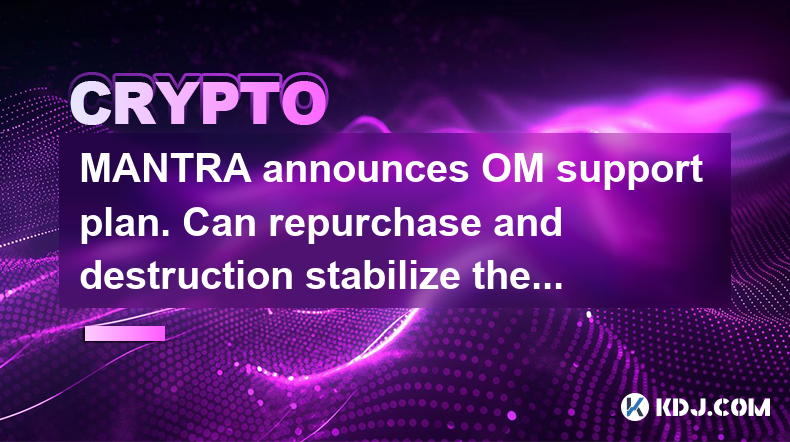
MANTRA announces OM support plan. Can repurchase and destruction stabilize the currency price?
Apr 21,2025 at 01:57pm
MANTRA, a notable player in the cryptocurrency ecosystem, has recently announced an OM support plan that includes mechanisms for repurchasing and destroying tokens. This move has sparked significant interest and discussion within the crypto community, particularly around its potential impact on the stability of the OM token's price. In this article, we ...
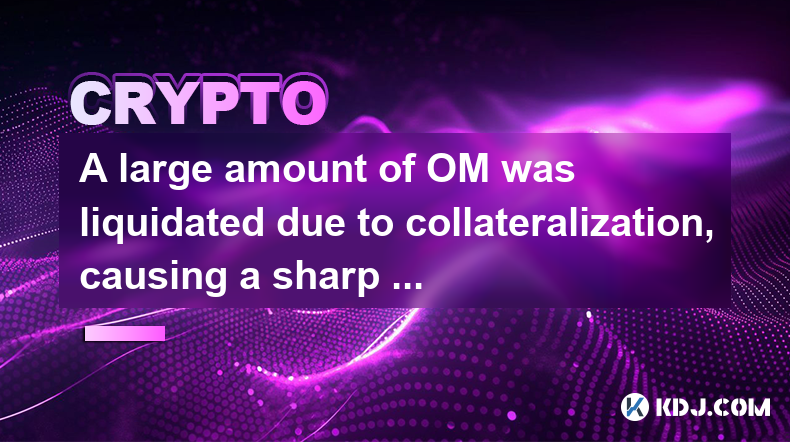
A large amount of OM was liquidated due to collateralization, causing a sharp drop? MANTRA analyzes the reasons for the market turmoil
Apr 21,2025 at 01:57am
The cryptocurrency market is known for its volatility, and sharp price movements can often be attributed to a variety of factors. Recently, a large amount of OM (Mantra DAO's native token) was liquidated due to collateralization issues, leading to significant market turmoil. In this article, MANTRA analyzes the reasons behind this event and the subseque...
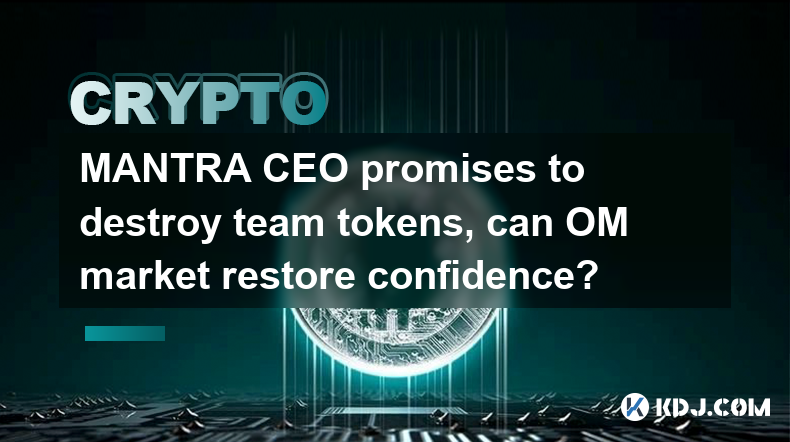
MANTRA CEO promises to destroy team tokens, can OM market restore confidence?
Apr 21,2025 at 08:28am
The recent announcement from the CEO of MANTRA about destroying team tokens has sparked a wave of discussions within the cryptocurrency community. This move is seen as a strategic effort to restore confidence in the OM market, which has been facing various challenges. The decision to burn team tokens is not just a simple action; it involves a series of ...
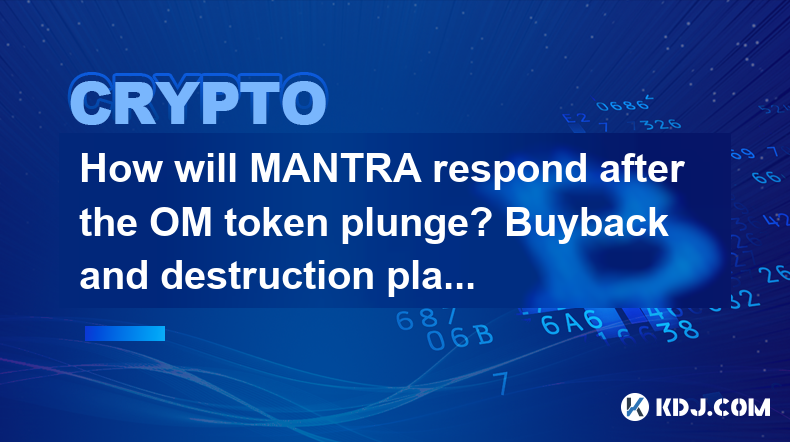
How will MANTRA respond after the OM token plunge? Buyback and destruction plan revealed
Apr 19,2025 at 11:42pm
The recent plunge in the OM token price has left many investors and enthusiasts of the MANTRA ecosystem concerned about the future stability and value of their holdings. In response to these market fluctuations, MANTRA has announced a comprehensive buyback and destruction plan aimed at restoring confidence and supporting the long-term health of the OM t...
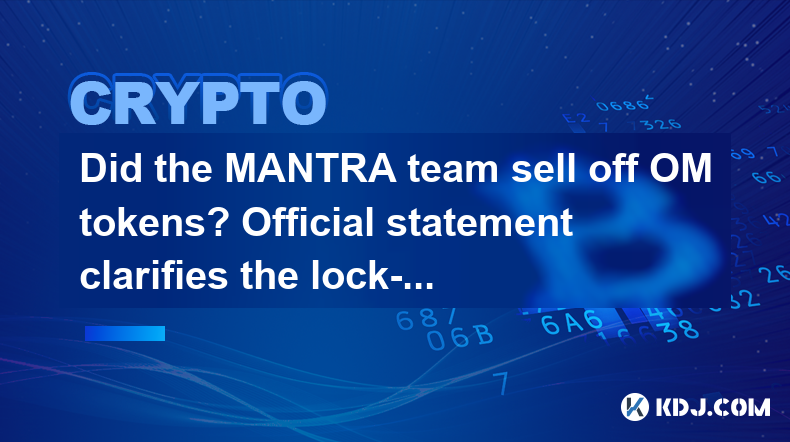
Did the MANTRA team sell off OM tokens? Official statement clarifies the lock-up situation
Apr 19,2025 at 10:56pm
The recent buzz around the MANTRA project and its native token, OM, has led to speculation and concerns within the cryptocurrency community about whether the MANTRA team has sold off their OM tokens. To address these concerns and clarify the situation, the MANTRA team has released an official statement detailing the lock-up situation of their tokens. Th...
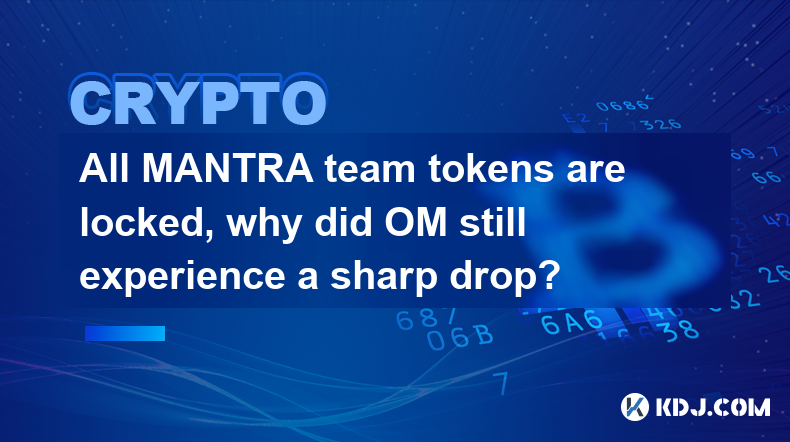
All MANTRA team tokens are locked, why did OM still experience a sharp drop?
Apr 20,2025 at 11:14am
Introduction to MANTRA and OM TokenThe MANTRA project is a blockchain platform that aims to provide a scalable and secure environment for decentralized applications (dApps). The native token of the MANTRA ecosystem is OM, which plays a crucial role in governance, staking, and other functionalities within the platform. Recently, the MANTRA team announced...
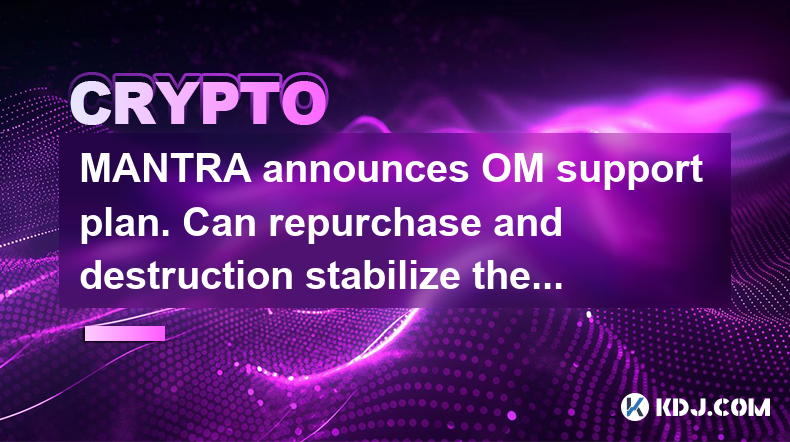
MANTRA announces OM support plan. Can repurchase and destruction stabilize the currency price?
Apr 21,2025 at 01:57pm
MANTRA, a notable player in the cryptocurrency ecosystem, has recently announced an OM support plan that includes mechanisms for repurchasing and destroying tokens. This move has sparked significant interest and discussion within the crypto community, particularly around its potential impact on the stability of the OM token's price. In this article, we ...
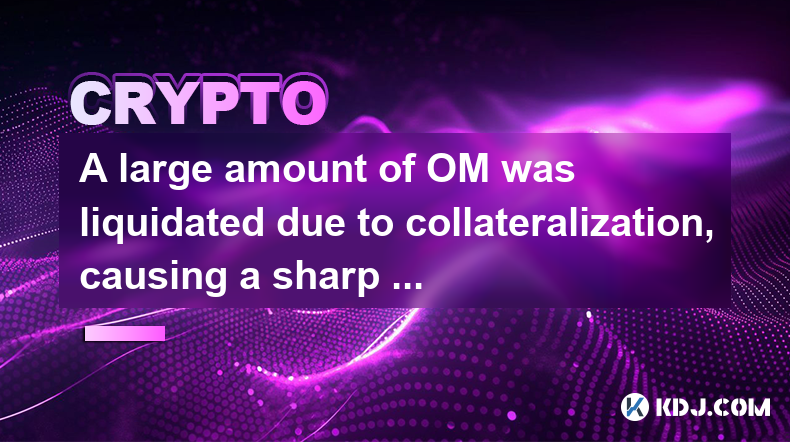
A large amount of OM was liquidated due to collateralization, causing a sharp drop? MANTRA analyzes the reasons for the market turmoil
Apr 21,2025 at 01:57am
The cryptocurrency market is known for its volatility, and sharp price movements can often be attributed to a variety of factors. Recently, a large amount of OM (Mantra DAO's native token) was liquidated due to collateralization issues, leading to significant market turmoil. In this article, MANTRA analyzes the reasons behind this event and the subseque...
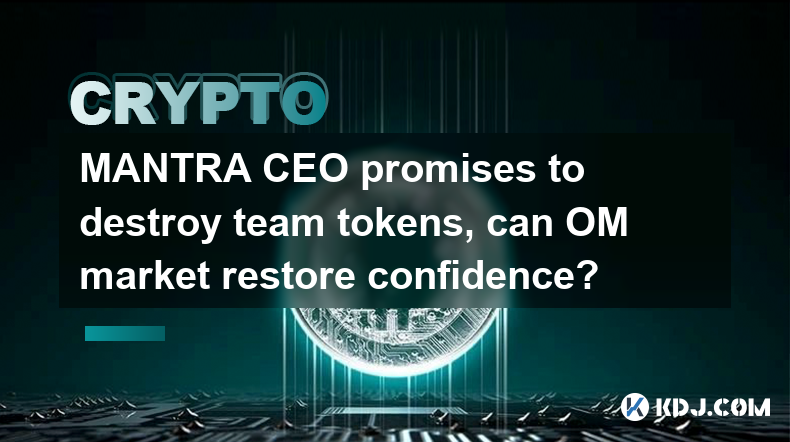
MANTRA CEO promises to destroy team tokens, can OM market restore confidence?
Apr 21,2025 at 08:28am
The recent announcement from the CEO of MANTRA about destroying team tokens has sparked a wave of discussions within the cryptocurrency community. This move is seen as a strategic effort to restore confidence in the OM market, which has been facing various challenges. The decision to burn team tokens is not just a simple action; it involves a series of ...
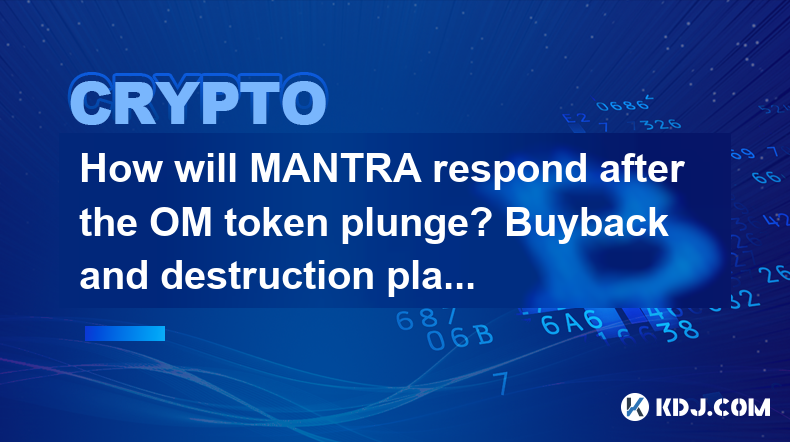
How will MANTRA respond after the OM token plunge? Buyback and destruction plan revealed
Apr 19,2025 at 11:42pm
The recent plunge in the OM token price has left many investors and enthusiasts of the MANTRA ecosystem concerned about the future stability and value of their holdings. In response to these market fluctuations, MANTRA has announced a comprehensive buyback and destruction plan aimed at restoring confidence and supporting the long-term health of the OM t...
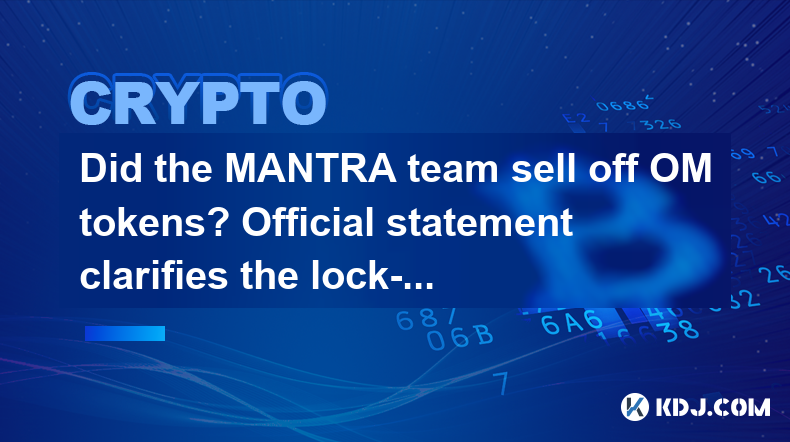
Did the MANTRA team sell off OM tokens? Official statement clarifies the lock-up situation
Apr 19,2025 at 10:56pm
The recent buzz around the MANTRA project and its native token, OM, has led to speculation and concerns within the cryptocurrency community about whether the MANTRA team has sold off their OM tokens. To address these concerns and clarify the situation, the MANTRA team has released an official statement detailing the lock-up situation of their tokens. Th...
See all articles
