-
Bitcoin
$108,270.9768
2.07% -
Ethereum
$2,489.8066
2.50% -
Tether USDt
$1.0004
0.01% -
XRP
$2.2035
0.66% -
BNB
$661.6608
2.32% -
Solana
$150.6425
2.13% -
USDC
$0.9999
-0.01% -
TRON
$0.2810
0.90% -
Dogecoin
$0.1645
3.05% -
Cardano
$0.5743
4.91% -
Hyperliquid
$38.8419
-0.15% -
Bitcoin Cash
$504.3134
-2.64% -
Sui
$2.8096
4.35% -
Chainlink
$13.3095
2.21% -
UNUS SED LEO
$8.9469
0.33% -
Avalanche
$17.9231
3.93% -
Stellar
$0.2340
0.74% -
Toncoin
$2.8458
3.21% -
Shiba Inu
$0.0...01158
3.47% -
Litecoin
$86.0738
1.94% -
Hedera
$0.1507
2.99% -
Monero
$319.8544
2.31% -
Polkadot
$3.4081
1.95% -
Dai
$1.0000
0.01% -
Bitget Token
$4.5645
0.91% -
Ethena USDe
$1.0002
0.00% -
Uniswap
$7.2959
5.27% -
Aave
$272.4623
2.90% -
Pepe
$0.0...09680
2.96% -
Pi
$0.4955
0.78%
What is TypeScript?
TypeScript's static typing enhances dApp development by catching errors early, crucial for blockchain security in the cryptocurrency ecosystem.
Apr 08, 2025 at 12:01 pm
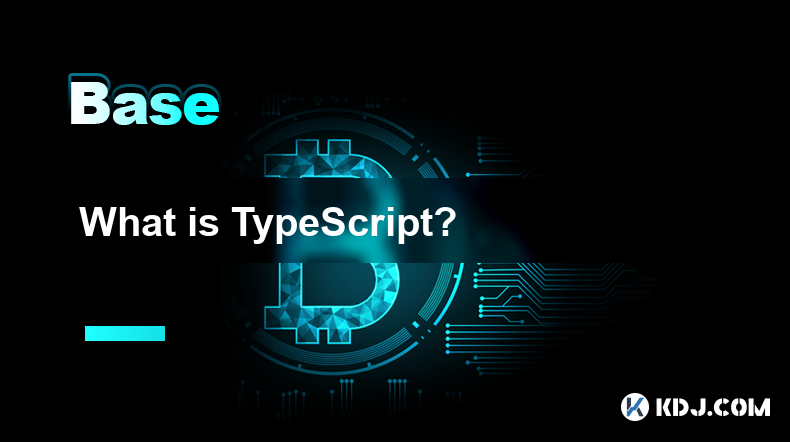
TypeScript is a programming language developed and maintained by Microsoft. It is a typed superset of JavaScript that compiles to plain JavaScript. TypeScript adds optional static typing, classes, and modules to JavaScript, making it easier to develop and maintain large-scale applications. In the context of the cryptocurrency circle, TypeScript is widely used for developing decentralized applications (dApps), blockchain platforms, and various tools and libraries that interact with cryptocurrencies.
Why TypeScript is Popular in the Cryptocurrency Circle
TypeScript's popularity in the cryptocurrency circle stems from its ability to enhance the development process of complex applications. The static typing feature of TypeScript helps developers catch errors early in the development cycle, which is crucial when working on blockchain and cryptocurrency projects where security and reliability are paramount. Additionally, TypeScript's compatibility with existing JavaScript codebases allows developers to gradually integrate it into their projects, making it an attractive choice for teams working on cryptocurrency-related software.
TypeScript in Decentralized Applications (dApps)
Decentralized applications, or dApps, are a significant part of the cryptocurrency ecosystem. TypeScript is often used in the development of dApps due to its robust type-checking capabilities. When building a dApp, developers can use TypeScript to define interfaces and types for smart contracts, ensuring that the interactions between the frontend and the blockchain are well-defined and less prone to errors. This is particularly important in the cryptocurrency world, where a single mistake can lead to significant financial losses.
TypeScript and Blockchain Platforms
Several blockchain platforms and frameworks support TypeScript, making it a go-to language for developers in the cryptocurrency space. For instance, Ethereum's Truffle Suite and The Graph both support TypeScript, allowing developers to write smart contracts and subgraphs with enhanced type safety. This support for TypeScript in blockchain platforms facilitates the creation of more secure and maintainable code, which is essential for the integrity of cryptocurrency networks.
TypeScript in Cryptocurrency Tools and Libraries
Beyond dApps and blockchain platforms, TypeScript is also used in various tools and libraries that are integral to the cryptocurrency ecosystem. Libraries like ethers.js and web3.js, which are used for interacting with Ethereum and other blockchain networks, have TypeScript versions that provide better developer experience and code reliability. These libraries are crucial for developers who need to build applications that interact with cryptocurrency networks, and TypeScript's features help ensure that these interactions are robust and error-free.
Getting Started with TypeScript in Cryptocurrency Development
To start using TypeScript in cryptocurrency development, developers need to follow a few key steps. Here's a detailed guide on how to set up a TypeScript environment for working on cryptocurrency projects:
Install Node.js and npm: TypeScript requires Node.js and npm (Node Package Manager) to be installed on your system. You can download and install them from the official Node.js website.
Install TypeScript: Once Node.js and npm are installed, you can install TypeScript globally using the following command in your terminal:
npm install -g typescript
Initialize a TypeScript Project: Create a new directory for your project and navigate to it in the terminal. Then, initialize a new TypeScript project with:
tsc --init
This command will create a
tsconfig.json
file in your project directory, which you can customize to suit your project's needs.Write Your First TypeScript File: Create a new file with a
.ts
extension, for example,main.ts
. You can start writing TypeScript code in this file. Here's a simple example of a TypeScript file that could be used in a cryptocurrency project:interface Transaction {
from: string;
to: string;
amount: number;
}function processTransaction(transaction: Transaction): void {
console.log(Processing transaction from ${transaction.from} to ${transaction.to} for ${transaction.amount} units.
);
}const exampleTransaction: Transaction = {
from: "0x123456789",
to: "0x987654321",
amount: 100
};processTransaction(exampleTransaction);
Compile TypeScript to JavaScript: To run your TypeScript code, you need to compile it to JavaScript. Use the following command to compile your
main.ts
file:tsc main.ts
This will generate a
main.js
file that you can run using Node.js.Run the Compiled JavaScript: Finally, you can run the compiled JavaScript file using Node.js:
node main.js
By following these steps, developers can set up a TypeScript environment and start building cryptocurrency-related applications with enhanced type safety and maintainability.
TypeScript and Smart Contract Development
Smart contracts are a fundamental component of many cryptocurrency platforms, and TypeScript can play a significant role in their development. When writing smart contracts, developers can use TypeScript to define the structure and behavior of the contract with clear type annotations. This can help prevent common errors such as incorrect data types or missing function parameters, which are critical in the context of smart contracts where errors can lead to financial losses.
For example, when developing a smart contract for a token on the Ethereum blockchain, developers can use TypeScript to define the token's interface and implement the contract logic with type safety. Here's a simple example of how TypeScript can be used to define a token smart contract:
interface Token {
name: string;
symbol: string;
totalSupply: number;
balanceOf(address: string): number;
transfer(from: string, to: string, amount: number): boolean;
}class MyToken implements Token {
name: string = "MyToken";
symbol: string = "MTK";
totalSupply: number = 1000000;
private balances: { [address: string]: number } = {};
constructor() {
this.balances["0x123456789"] = this.totalSupply;
}
balanceOf(address: string): number {
return this.balances[address] || 0;
}
transfer(from: string, to: string, amount: number): boolean {
if (this.balances[from] < amount) {
return false;
}
this.balances[from] -= amount;
this.balances[to] = (this.balances[to] || 0) + amount;
return true;
}
}
const token = new MyToken();
console.log(token.balanceOf("0x123456789")); // Output: 1000000
console.log(token.transfer("0x123456789", "0x987654321", 1000)); // Output: true
console.log(token.balanceOf("0x987654321")); // Output: 1000
This example demonstrates how TypeScript can be used to define a token smart contract with clear type annotations, making it easier to understand and maintain the contract's logic.
TypeScript in Cryptocurrency Wallets
Cryptocurrency wallets are another area where TypeScript is commonly used. When developing a wallet application, TypeScript can help ensure that the code handling sensitive operations like key management and transaction signing is robust and less prone to errors. For instance, TypeScript can be used to define interfaces for wallet addresses, private keys, and transaction data, ensuring that these critical components are handled correctly.
Here's an example of how TypeScript can be used in a simple wallet application:
interface WalletAddress {
address: string;
privateKey: string;
}interface TransactionData {
from: string;
to: string;
amount: number;
fee: number;
}
class Wallet {
private addresses: WalletAddress[] = [];
addAddress(address: WalletAddress): void {
this.addresses.push(address);
}
getBalance(address: string): number {
// Simulated balance retrieval
return Math.floor(Math.random() * 1000);
}
sendTransaction(transaction: TransactionData): boolean {
// Simulated transaction sending
if (this.getBalance(transaction.from) < transaction.amount + transaction.fee) {
return false;
}
console.log(`Sending ${transaction.amount} from ${transaction.from} to ${transaction.to} with fee ${transaction.fee}`);
return true;
}
}
const wallet = new Wallet();
wallet.addAddress({ address: "0x123456789", privateKey: "privateKey1" });
wallet.addAddress({ address: "0x987654321", privateKey: "privateKey2" });
const transaction: TransactionData = {
from: "0x123456789",
to: "0x987654321",
amount: 100,
fee: 1
};
console.log(wallet.sendTransaction(transaction)); // Output: true or false based on balance
This example shows how TypeScript can be used to define interfaces and implement wallet functionality with type safety, ensuring that the wallet application is more reliable and secure.
Frequently Asked Questions
Q: Can TypeScript be used with existing JavaScript cryptocurrency projects?
A: Yes, TypeScript is designed to be a superset of JavaScript, which means it can be integrated with existing JavaScript projects. Developers can gradually add TypeScript to their codebase, taking advantage of its type-checking features without needing to rewrite their entire project.
Q: Are there any performance differences between TypeScript and JavaScript in cryptocurrency applications?
A: TypeScript itself does not introduce performance differences since it compiles to JavaScript. However, the use of TypeScript can lead to more efficient development and maintenance, which can indirectly improve the performance of cryptocurrency applications by reducing errors and improving code quality.
Q: How does TypeScript help with security in cryptocurrency development?
A: TypeScript helps with security in cryptocurrency development by providing static type checking, which can catch errors early in the development process. This is particularly important in cryptocurrency applications where security is critical, as it helps prevent common mistakes that could lead to vulnerabilities or financial losses.
Q: Can TypeScript be used for developing cryptocurrency exchanges?
A: Yes, TypeScript can be used for developing cryptocurrency exchanges. Its type safety features can help ensure that the complex logic involved in trading and order management is more reliable and less prone to errors, which is crucial for the security and integrity of a cryptocurrency exchange.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- XRP Price Targets $2.40 After Descending Channel Breakout: Is $40 Next?
- 2025-07-03 08:50:12
- All Blacks' Loose Forward Conundrum: New Faces and Familiar Battles
- 2025-07-03 08:30:12
- Bitcoin's Wild Ride: Open Interest, Institutional Bets, and Billions on the Line
- 2025-07-03 08:30:12
- Bitcoin, Strategy, & Profit: MSTR's Crypto Playbook and Trump's Digital Diversification
- 2025-07-03 08:50:12
- INJ Price Bull Rally: Smashing Long-Term Resistance – Can It Last?
- 2025-07-03 09:10:12
- Robinhood, OpenAI, and Wallet History: A Tokenized Tale of Intrigue
- 2025-07-03 09:10:12
Related knowledge
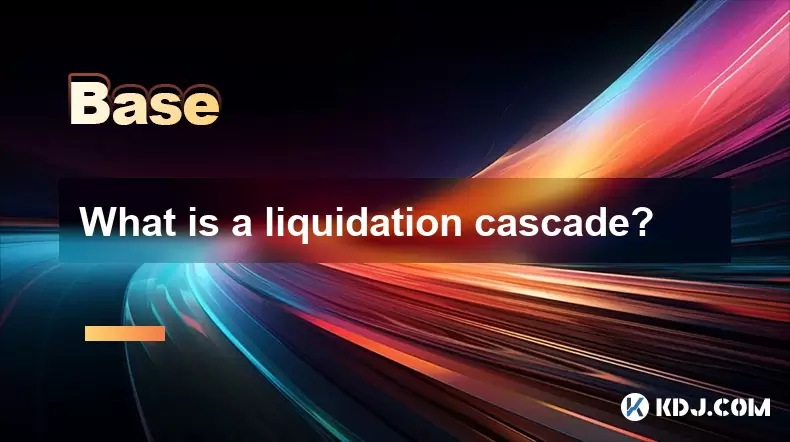
What is a liquidation cascade?
Jul 03,2025 at 07:15am
Understanding the Concept of LiquidationIn the realm of cryptocurrency trading, liquidation refers to the process by which a trader's position is automatically closed due to insufficient funds to maintain the leveraged trade. This typically occurs when the market moves against the trader's position and their account equity falls below the required maint...
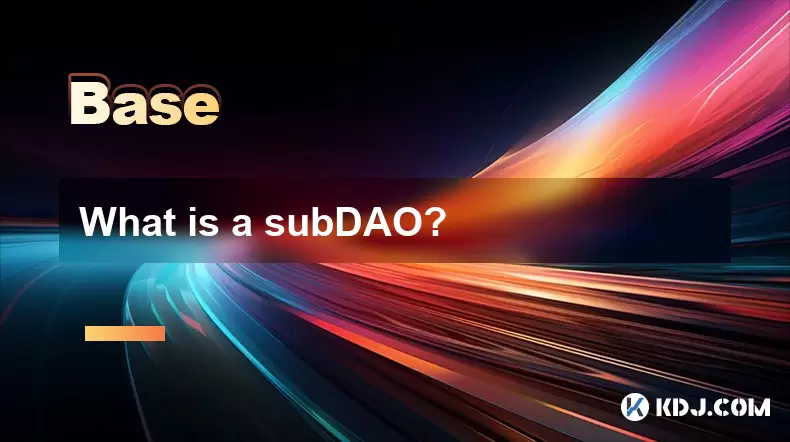
What is a subDAO?
Jul 03,2025 at 09:36am
Understanding the Concept of SubDAOA SubDAO, short for Sub-Decentralized Autonomous Organization, is a specialized entity that operates under the umbrella of a larger DAO (Decentralized Autonomous Organization). It functions with its own set of rules, governance mechanisms, and tokenomics while remaining aligned with the overarching goals of the parent ...
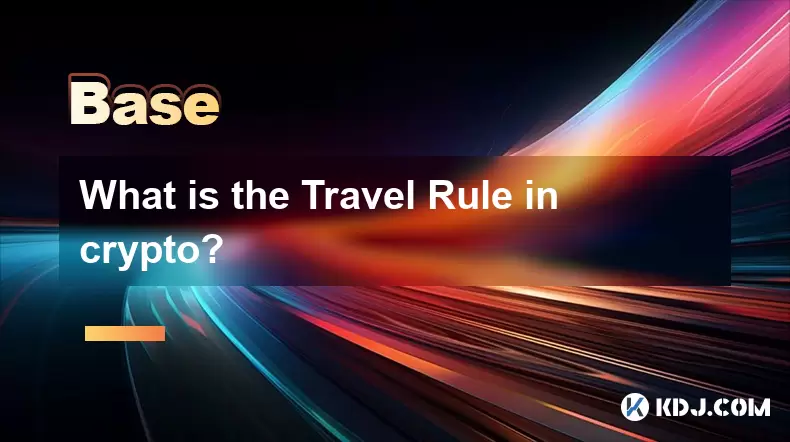
What is the Travel Rule in crypto?
Jul 03,2025 at 10:28am
Understanding the Travel Rule in CryptocurrencyThe Travel Rule is a regulatory requirement initially introduced by the Financial Action Task Force (FATF) for traditional financial institutions. It has since been extended to cryptocurrency transactions, especially those involving Virtual Asset Service Providers (VASPs). The core purpose of this rule is t...
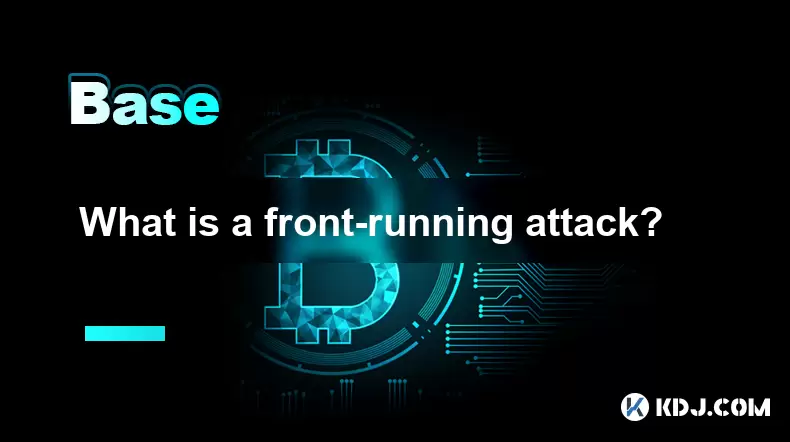
What is a front-running attack?
Jul 03,2025 at 07:36am
Understanding Front-Running in the Cryptocurrency EcosystemIn the decentralized and fast-paced world of cryptocurrency, front-running is a controversial practice that exploits transaction transparency to gain unfair advantages. Unlike traditional finance, where such practices are often executed by insiders with access to non-public data, crypto front-ru...
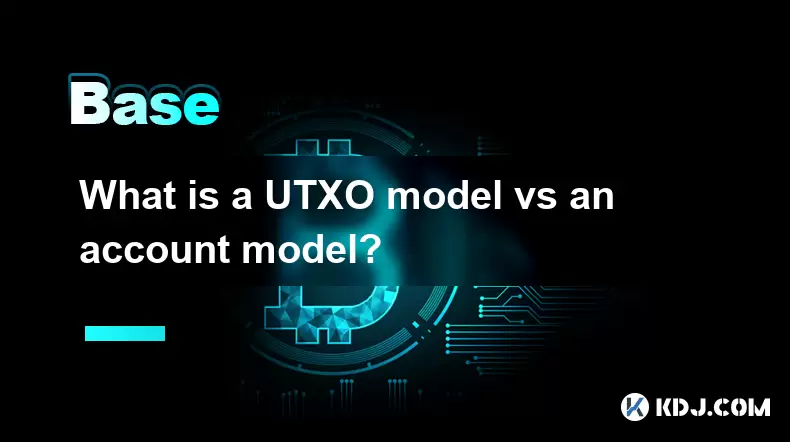
What is a UTXO model vs an account model?
Jul 03,2025 at 05:43am
Understanding the UTXO ModelThe UTXO (Unspent Transaction Output) model is a fundamental concept in blockchain technology, particularly prominent in Bitcoin and other similar cryptocurrencies. In this model, transactions are structured as inputs and outputs. When a user sends cryptocurrency, they reference previous unspent outputs as inputs and create n...
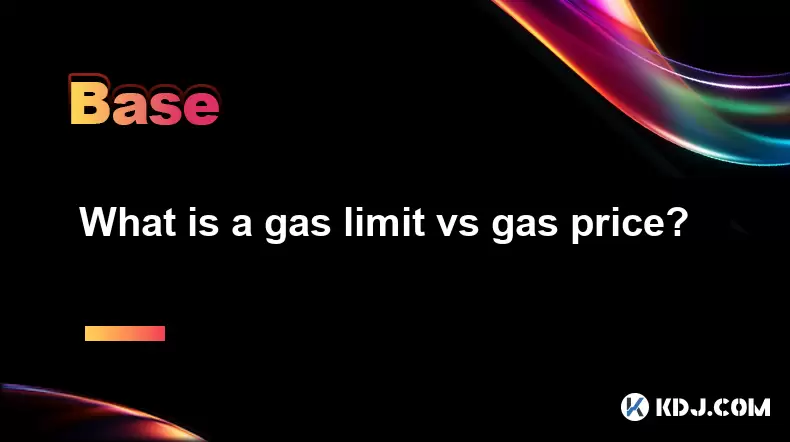
What is a gas limit vs gas price?
Jul 03,2025 at 07:42am
Understanding the Basics of Gas in Blockchain TransactionsIn the world of blockchain, especially within Ethereum-based networks, gas is a crucial concept that determines how transactions are processed. It represents the fee required to successfully conduct a transaction or execute a smart contract on the network. The term 'gas' is metaphorical, much lik...
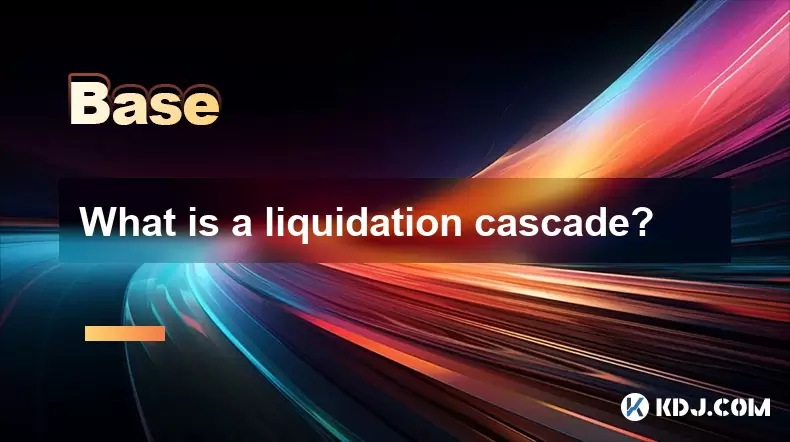
What is a liquidation cascade?
Jul 03,2025 at 07:15am
Understanding the Concept of LiquidationIn the realm of cryptocurrency trading, liquidation refers to the process by which a trader's position is automatically closed due to insufficient funds to maintain the leveraged trade. This typically occurs when the market moves against the trader's position and their account equity falls below the required maint...
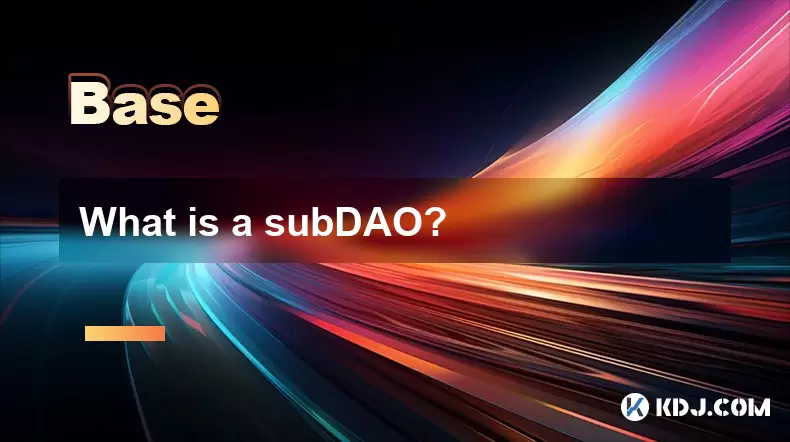
What is a subDAO?
Jul 03,2025 at 09:36am
Understanding the Concept of SubDAOA SubDAO, short for Sub-Decentralized Autonomous Organization, is a specialized entity that operates under the umbrella of a larger DAO (Decentralized Autonomous Organization). It functions with its own set of rules, governance mechanisms, and tokenomics while remaining aligned with the overarching goals of the parent ...
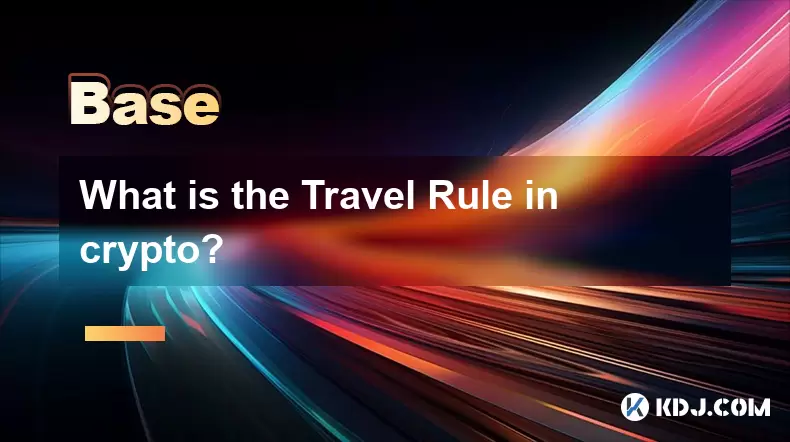
What is the Travel Rule in crypto?
Jul 03,2025 at 10:28am
Understanding the Travel Rule in CryptocurrencyThe Travel Rule is a regulatory requirement initially introduced by the Financial Action Task Force (FATF) for traditional financial institutions. It has since been extended to cryptocurrency transactions, especially those involving Virtual Asset Service Providers (VASPs). The core purpose of this rule is t...
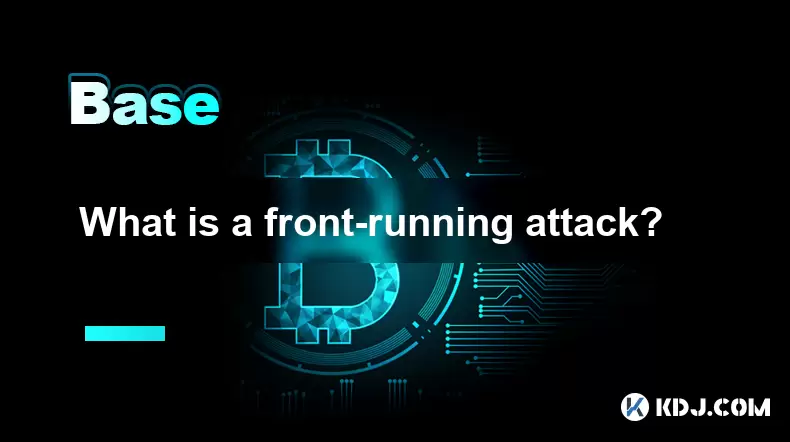
What is a front-running attack?
Jul 03,2025 at 07:36am
Understanding Front-Running in the Cryptocurrency EcosystemIn the decentralized and fast-paced world of cryptocurrency, front-running is a controversial practice that exploits transaction transparency to gain unfair advantages. Unlike traditional finance, where such practices are often executed by insiders with access to non-public data, crypto front-ru...
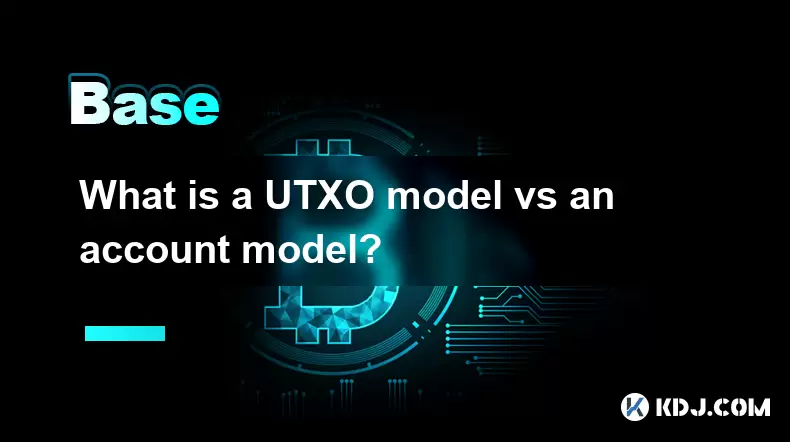
What is a UTXO model vs an account model?
Jul 03,2025 at 05:43am
Understanding the UTXO ModelThe UTXO (Unspent Transaction Output) model is a fundamental concept in blockchain technology, particularly prominent in Bitcoin and other similar cryptocurrencies. In this model, transactions are structured as inputs and outputs. When a user sends cryptocurrency, they reference previous unspent outputs as inputs and create n...
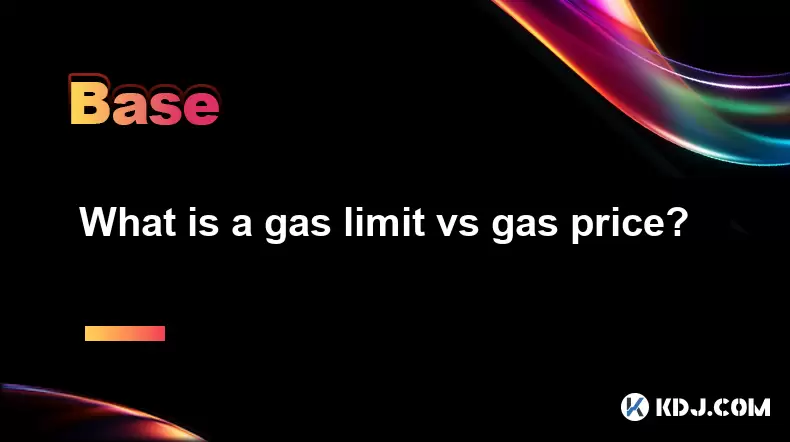
What is a gas limit vs gas price?
Jul 03,2025 at 07:42am
Understanding the Basics of Gas in Blockchain TransactionsIn the world of blockchain, especially within Ethereum-based networks, gas is a crucial concept that determines how transactions are processed. It represents the fee required to successfully conduct a transaction or execute a smart contract on the network. The term 'gas' is metaphorical, much lik...
See all articles
